Permutations in C# Using Recursion
Introduction
Recently, while searching for a method to descramble a string of characters, I came across an article by Alexander Bogomolny, entitled ‘Counting and Listing All Permutations’. The article, from Interactive Mathematics Miscellany and Puzzles, introduces three separate algorithms all capable of generating a list of permutations for a given set of elements.
To rewrite a word descrambler program in C# 3.0, originally created a number of years ago in VBA for Microsoft Excel, I used one of article’s three Java-based algorithms, the recursive algorithm, rewriting in C#. In addition, I added a few enhancements, including the ability of the recursive algorithm to apply the permutations to a string of characters, input by the user, and return each permutation of the input string back to the user.
Background
First, a quick math review. Permutations are the possible combinations of elements in a set. For example, given a set of three integers: { 0, 1, 2 }, there are six possible permutations of the three integers: { 012, 021, 102, 120, 201, 210 }. The total number of permutations of a set of elements can be expressed as 3! or n factorial, where n represents the number of elements in the set. Three elements have 3! permutations, or 1 x 2 x 3 = 6. Four elements have 4! permutations, or 1 x 2 x 3 x 4 = 24. Five elements have 120; six elements have 720, and so on. The variations add up quickly; their growth is exponential.
Note, the recursive algorithm presented by Bogomolny returns all of the permutations of a given set of elements, and does not account for duplicate elements within the set, which would in turn, produce duplicate permutations. For example, given the set of four characters { t, e, s, t }, the algorithm will return { test } twice; once for the permutation { 0123 } and once for { 3120 }. To calculate permutations with repetition of elements using C#, I recommend starting with the CodeProject article by Adrian Akison entitled ‘Permutations, Combinations, and Variations using C# Generics’.
There are many other comprehensive articles on the Internet and on CodeProject dealing with permutations. I will not repeat what has already been written. The goal of this article is to demonstrate how Bogomolny’s deceivingly simple code can produce a complete list of permutations of a given set of input characters.
Using the Code
To demonstrate the recursive algorithm, I have created a simple console application using Microsoft Visual Studio 2008. The source code for this article consists of a single class (.cs) file containing two classes. When compiled and executed, the console application asks the user to input a string of characters (alpha, numeric, or symbols). The application converts the input string to a character array and stores its length (the number of characters input).
class Program
{
static void Main(string[] args)
{
Console.Write("Input String>");
string inputLine = Console.ReadLine();
Recursion rec = new Recursion();
rec.InputSet = rec.MakeCharArray(inputLine);
rec.CalcPermutation(0);
Console.Write("# of Permutations: " + rec.PermutationCount);
}
}
Using recursion, the application then calculates each possible permutation of a set of elements given the number of characters input, as a series of integers, representing each characters initial position, starting from 0. For example, the user inputs the character string ‘ugb’, from which the application calculates the six possible permutations for three elements starting from 0: { 012, 021, 102, 120, 201, 210 }. The application then retrieves individual characters from the character array { u, g, b }, corresponding to the permutations and returns them to the user: { ugb, ubg, gub, gbu, bug, bgu }.
/// <summary>
/// Algorithm Source: A. Bogomolny, Counting And Listing
/// All Permutations from Interactive Mathematics Miscellany and Puzzles
/// http://www.cut-the-knot.org/do_you_know/AllPerm.shtml, Accessed 11 June 2009
/// </summary>
class Recursion
{
private int elementLevel = -1;
private int numberOfElements;
private int[] permutationValue = new int[0];
private char[] inputSet;
public char[] InputSet
{
get { return inputSet; }
set { inputSet = value; }
}
private int permutationCount = 0;
public int PermutationCount
{
get { return permutationCount; }
set { permutationCount = value; }
}
public char[] MakeCharArray(string InputString)
{
char[] charString = InputString.ToCharArray();
Array.Resize(ref permutationValue, charString.Length);
numberOfElements = charString.Length;
return charString;
}
Read more: Codeproject
6 steps to implement DUAL security on WCF using User name + SSL
Posted by
jasper22
at
10:49
|
Introduction and Goal
Basics Transport and Message level security
Step 1:- Customize ‘WsHttp’ Bindings with security mode and credential type
Step 2:- Create your custom validator class
Step 3:- Define runtime behavior
Step 4:- Define SSL for your WCF service
Step 5 :- Consume WCF Service
Step 6: Run your WCF service
Source code
My other WCF articles
Basics Transport and Message level security
Step 1:- Customize ‘WsHttp’ Bindings with security mode and credential type
Step 2:- Create your custom validator class
Step 3:- Define runtime behavior
Step 4:- Define SSL for your WCF service
Step 5 :- Consume WCF Service
Step 6: Run your WCF service
Source code
My other WCF articles
Introduction and Goal
In the article we will try to apply DUAL security using transport plus message on WCF services. So we will first try to understand the basic concepts of WCF security i.e. transport and message. Once we understand the concept we will move step by step in to how to implement SSL and user name security on WCF services.
In case you are a complete fresher to WCF you can start from here.
Watch my 500 videos on various topics like design patterns,WCF, WWF , WPF, LINQ ,Silverlight,UML, Sharepoint ,Azure,VSTS and lot more @ click here , you can also view my WCF videos Part :-1 and Part:- 2
Enjoy my free ebook which covers major .NET related topics like WCF,WPF,WWF,Ajax,Core .NET,SQL Server, Architecture and lot more Download from here

Basics Transport and Message level security
On a broader basis WCF supports two kinds of security, transport level and message level security. Transport means the medium on which WCF data travels while message means the actual data packets sent by WCF.
Transport medium can be protocols like TCP, HTTP, MSMQ etc. These transport mediums by themself provide security features like HTTP can have SSL security (HTTPS). WCF has the capability of leveraging underlying transport security features on WCF service calls.
Message level security is provided in the data itself using WS-Security. In other words it’s independent of the transport protocol. Some examples of message level security are messages encrypted using encryption algorithm, messages encrypted using X509 certificate etc, messages protected using username etc.
WCF gives you an option to either just use message level security in stand alone, transport level in stand alone or combination of both. If you are interested in how to do message level security and transport security in a standalone manner you can read more from here.
Read more: .NET Funda
Add Facebook Like to your Silverlight Application
Introduction
In this article you will find out what you need to do if you want to add a Facebook Like button to your Silverlight application.
If you want to see a Live Example see my XAML blog.
Using the code
The strait forward approach is to add a button to your application and make it look the same as the one on Facebook. Then you need to Facebook authenticate the person that clicked the like button and add your Facebook page to list of pages that he likes. This is very difficult to implement and requires knowledge of the Facebook API. You can also try to use the Codeplex Facebook SDK but that requires you to have a Facebook application, if you have a Facebook personal page or a website the SDK will not help.
The simple way to do this is to integrate the HTML Facebook like button into your Silverlight application. In order to do this you need an HtmlHost control that will display the Html like button. Since the standard Microsoft WebBrowser control only works when running in out-of-browser mode I have used the divelements free HtmlHost control. In order to use this control you need to set windowless parameter of your Silverlight plugin to true.
Here is a step by step example:
Create a new project named FacebookLikeApplication in Visual Studio 2010 using the SilverlightApplication template; when creating the project, check the “Host the Silverlight application in a new Web site” option.
Download the divelements Silverlight Tools from here; extract, unblock, and add a reference of Divelements.SilverlightTools.dll to your FacebookLikeApplication project.
Open FacebookLikeApplicationTestPage.aspx and FacebookLikeApplicationTestPage.html, identify the <object .. /> element and add the windowless parameter.
<form id="form1" runat="server" style="height:100%">
<div id="silverlightControlHost">
<object data="data:application/x-silverlight-2,"
type="application/x-silverlight-2"
width="100%" height="100%">
<param name="source" value="ClientBin/FacebookLikeApplication.xap"/>
<param name="onError" value="onSilverlightError" />
<param name="background" value="white" />
<param name="minRuntimeVersion" value="4.0.50826.0" />
<param name="autoUpgrade" value="true" />
<param name="windowless" value="true" />
<a href="<a href="http://go.microsoft.com/fwlink/?LinkID=149156&v=4.0.50826.0" >
style="text-decoration:none">
Open the MainPage.xaml and add the HtmlHost control.
<UserControl x:Class="FacebookLikeApplication.MainPage"
xmlns="<a href="http://schemas.microsoft.com/winfx/2006/xaml/presentation" >
xmlns:x="<a href="http://schemas.microsoft.com/winfx/2006/xaml" >
xmlns:d="<a href="http://schemas.microsoft.com/expression/blend/2008" >
xmlns:mc="<a href="http://schemas.openxmlformats.org/markup-compatibility/2006" >
xmlns:divtools="clr-namespace:Divelements.SilverlightTools;
assembly=Divelements.SilverlightTools"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
Open the Facebook Like button website from here, fill in the fields, and retrieve the Facebook Like iframe.
Open MainPage.xaml.cs and set the Facebook Like iframe to the htmlHost.SourceHtml property; after you set the value, replace the double quotes from the iframe text with a single quote.
Read more: Codeproject
Silverlight, HttpWebRequest, HTTP Stacks and the UI Thread
Posted by
jasper22
at
15:46
|
This one comes from a customer who thought that they were seeing an issue in using the browser HTTP stack in Silverlight to make lots of HTTP requests. The issue seemed to be that the stack was blocking the UI thread and causing the UI to stutter.
The suspicion was that the blocking was occurring while data was being read from the stream that is handed back to you when you’re reading an HTTP response.
I thought I’d see if I could reproduce this behaviour myself and so I set out to put something together that made quite a lot of use of the browser HTTP stack and quite a lot of HTTP requests.
By the way – if this talk of browser/client HTTP stacks in Silverlight doesn’t resonate then take a look at this video over here which runs through the basics of it.
I put together a quick UI;
<Grid
x:Name="LayoutRoot"
Background="Black">
<MediaElement
Stretch="Fill"
AutoPlay="True"
MediaEnded="MediaElement_MediaEnded"
Source="Wildlife.wmv" />
<Viewbox>
<StackPanel>
<TextBlock
Foreground="White"
Text="{Binding MegabytesRead,StringFormat=MB Read \{0:F2\} }" />
<TextBlock
Foreground="White"
Text="{Binding MegabytesPerSecond,StringFormat=MBps \{0:F2\} }" />
<TextBlock
Foreground="White"
Text="{Binding OpenRequests,StringFormat=Requests \{0\} }" />
<TextBlock
Foreground="White"
Text="{Binding TimerTick,StringFormat=Timer \{0\} }" />
</StackPanel>
</Viewbox>
</Grid>
This is playing a video (wildlife.wmv which ships with Windows 7) which I have embedded into my XAP. It’s also attempting to display (via binding) 4 pieces of data;
Total megabytes downloaded over HTTP
Number of megabytes read in the last second
Number of open HTTP requests in flight
A “tick” text block which will simple turn True/False as a timer ticks
and I put some code together behind this;
public partial class MainPage : UserControl, INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
//#error This needs a Uri to a big file to download (mine is 1.5MB)
static Uri videoFile = new Uri("http://localhost/wildlife.wmv", UriKind.Absolute);
const int threadCount = 10;
long bytesRead = 0;
long requestsOpen = 0;
DateTime startTime;
List<byte[]> bufferPool;
public bool TimerTick
{
get
{
return (_TimerTick);
}
set
{
_TimerTick = value;
RaisePropertyChanged("TimerTick");
}
}
bool _TimerTick;
public double MegabytesPerSecond
{
get
{
return (_MegabytesPerSecond);
}
set
{
_MegabytesPerSecond = value;
RaisePropertyChanged("MegabytesPerSecond");
}
}
double _MegabytesPerSecond;
public double MegabytesRead
{
get
{
return (_Count);
}
Read more: Mike Taulty's Blog
MVVM Light - what's the Messenger?
Posted by
jasper22
at
15:45
|
MVVM Light offers a class specific to every ViewModel instance that is used in the context of an application - Messenger. One might ask - what exactly is it and why it is needed? The answer is simple - in a more complex application, ViewModel instances are not standalone entities. Chances are you would like to pass specific data from one model to another, and Messenger is facilitating this process.
Let's look at specific source code to illustrate its activity. Initially, I have a standard test view model (named TestViewModel):
public class TestViewModel : ViewModelBase
{
public TestViewModel()
{
}
private string tS = "tS";
public string TestString
{
get
{
return tS;
}
set
{
tS = value;
RaisePropertyChanged("TestString");
}
}
}
Nothing super useful here - just a dummy string property with an associated field that has a default value. Let's say I have another ViewModel instance that contains additional data (not necessarily related to the ViewModel above):
public class MainViewModel : ViewModelBase
{
public MainViewModel()
{
}
private string pA = "pA";
public string PropertyA
{
get
{
return pA;
}
set
{
RaisePropertyChanged("PropertyA");
}
}
}
Read more: Windows Phone 7
convert Blend4 sketchflow to sl4 application (c#)
Posted by
jasper22
at
15:29
|
After making a nice project prototype you feel the need to use in a real deployment then follow the following steps.
back up your project
In the Projects panel, right-click the top project folder (e.g. SilverlightPrototype1), and then click Open Folder in Windows Explorer.
In Windows Explorer, right-click the .csproj file (for example, SilverlightPrototype1.csproj), click Open With, and then click Notepad++ or scite.
In the text file, locate and then delete the following two lines (capability flags):
<ExpressionBlendPrototypingEnabled>false</ExpressionBlendPrototypingEnabled>
<ExpressionBlendPrototypeHarness>true</ExpressionBlendPrototypeHarness>
Save and close the text file. When prompted to reload the project file, click Yes.
In the References folder, locate and right-click Microsoft.Expression.Prototyping.Runtime.dll, and then click Remove from Project.
Right-click the top project folder again, and then click Add Reference. In the Add Reference dialog box, browse to the Microsoft Silverlight SDK, and then locate and click the System.Windows.Controls.Navigation.dll. The default location is C:\Program Files\Microsoft SDKs\Silverlight\v3.0\Libraries\Client.
Click Open.
In the Projects panel, right-click the next project folder (for example, SilverlightPrototype1Screens), and then click Open Folder in Windows Explorer.
In Windows Explorer, right-click the .csproj file (for example, SilverlightPrototype1Screens.csproj), click Open With, and then click Notepad.
In the text file, locate and then delete the following two lines (capability flags):
<ExpressionBlendPrototypingEnabled>false</ExpressionBlendPrototypingEnabled>
<ExpressionBlendPrototypeHarness>true</ExpressionBlendPrototypeHarness>
Save and close the text file.
In the References folder, locate and right-click Microsoft.Expression.Prototyping.Runtime.dll, and then click Remove from Project.
In the top project folder, expand the App.xaml node, and then double-click App.xaml.cs to open the file.
In the App.xaml.cs file, locate the following code:
[assembly: Microsoft.Expression.Prototyping.Services.SketchFlowLibraries("MyProject.Screens")]
Note the name of the screens assembly for your project, which appears in place of MyProject.Screens in the code
Read more: http://erew.org/?p=624
Silverlight: Adding Google Streets View
Posted by
jasper22
at
15:08
|
This summary is not available. Please
click here to view the post.
BitLocker Drive Encryption Deployment Guide for Windows 7
Posted by
jasper22
at
15:08
|
This document describes the various aspects of deploying BitLocker™ Drive Encryption on computers running Windows® 7 Enterprise or Windows® 7 Ultimate in an organizational environment. This guide is intended for use by a deployment specialist or deployment team. It assumes that you have a good understanding of automated Windows deployment, Active Directory® Domain Services (AD DS) schema extensions, and Group Policy. There is no single recommended method to deploy Windows® 7 with BitLocker, and most deployments of Windows 7 in large organizations differ based on environmental-specific requirements. This guide provides information on the following topics that you should be familiar with when you deploy BitLocker in an organization:
- Getting Started with BitLocker Drive Encryption
- Best Practices for BitLocker in Windows 7
- Planning to Deploy Windows 7 BitLocker Drive Encryption
- Unlocking Removable Drives on Windows XP and Windows Vista
- Backing Up BitLocker and TPM Recovery Information to AD DS
- Using Certificates with BitLocker
- Using Smart Cards with BitLocker
- Using Data Recovery Agents with BitLocker
- Enabling BitLocker by Using the Command Line
- Using the BitLocker Drive Preparation Tool for Windows 7
- Enabling BitLocker by Using a WMI Script
- Auditing BitLocker Deployments
- BitLocker Deployment Guide: Appendices
Read more: Technet
כלי הפיתוח שאתם פשוט חייבים
Posted by
jasper22
at
12:44
|
רגע לפני שאנחנו יוצאים לקרב המכריע רצוי מאוד לוודא שכולם עשו את ההכנות הרצויות, כדי שלא נגלה באמצע הדרך שהמימיות ריקות. גם בעולם פיתוח התוכנה צריך לבצע את ההכנות הללו. אמנם מדי פעם תמצאו חבורה מצומצמת של יוצאי ממר"ם או 8200 שמצליחים לעשות קפיצה קוואנטית ולשנות את התעשייה, אבל גם הם בד"כ הגיעו עם הרגלים ותשתיות מוכנות מהבית.
אז מה צריך להכין?
- Source Control: המקום שבו ישמר הנכס החשוב ביותר שלכם אחרי האנשים. הכלים המובילים היום הם TFS בעולם המיקרוסופט, SVN בעולם שאינו מיקרוסופט (וגם לא מעט מפתחי .Net נהנים פחות או יותר להשתמש בו) ו - Git הכוכב העולה החדש המגיע מעולם הפיתוח המבוזר של הקוד הפתוח. כמובן שיש כלים נוספים בינהם כלי Rational.
- כלי ניהול משימות: המקום שבו תרשמו את המשימות שלכם. הכלי הבסיסי ביותר שכולכם תחזרו אליו (או תתחילו איתו) כששום דבר לא יעבוד: הלוח המחיק או ה - White Board. מלבדו ישנם כלים רבים המתחלקים לשלושה סוגים:
- כלים הכוללים תמיכה בניהול תהליך ניפוי הבאגים (Bugzilla ו - Trac).
- כלים עם אוריאנטציה של ניהול משימות כדוגמת BaseCampHQ (מוגש בהמלצה חמה).
- כלים שמשתלבים בסביבת הפיתוח כדוגמת ה - TFS של מיקרוסופט וה - Rational של IBM.
- סביבת הפיתוח: לכל שפה ולכל מפתח יש סביבת העבודה המועדפת עליו, ולכן אני אמנע מלהמליץ לכם במה לבחור. עם זאת ישנם תוספים רבים שמאוד מומלצים כדי לשפר את איכות הקוד ומהירות הכתיבה שלו. לדוגמה למפתחי .Net השימוש ב - Resharper הוא הכרח ותצטרכו לפתוח את הכיס בשבילו.
- Unit Tests: כתבתם קוד? רצוי מאוד שתהיה לכם סביבה מסודרת לכתיבת בדיקות יחידה (וגם בדיקות נרחבות יותר במקרה הצורך). מימוש טוב של בדיקות אלו יאפשר לכם לבצע אוטומציה מלאה לקוד ולזהות שבירה שלו (ולחסוך הרבה שעות שינה וכאבי לב). הסביבה הנפוצה ביותר בתחום היא משפחת ה - XUnit הכוללת את JUnit ל - Java, ה - NUnit לעולם ה - .Net ו - SimpleTest לעולם ה - PHP. ההמלצה שלי: אל תחפשו בכלל פתרון אחר למשפחה הזאת. טיפ למתקדמים: תוכלו לשלב פתרונות Mock המסמלצים מוצרים חיצוניים כמו בסיסי נתונים על מנת לבודד את בדיקות היחידה שלכם. אחת החברות המובילות בשוק זה היא TypeMock הישראלית.
- Code Review Procedures: רגע לפני שאתם מכניסים את הקוד לתוך כלי ניהול התצורה שבחרתם, מומלץ מאוד שכל שורת קוד ששונתה תעבור לפחות עין בוחנת נוספת. איך עושים את זה? ראו את הפוסט אז מה היה לנו?
- Continuous Integration: או בקיצור CI: אחרי שכתבתם קוד מסודר, הטמעתם מערכת ניהול תצורה ויש לכם אפילו 100% כיסוי עם בדיקות אוטומטיות, למה לא לדאוג שהגרסה האחרונה שנמצאת במערכת בקרת התצורה תקינה ואין בה באגים? למה לחכות עד לסיום הגרסה ובדיקות האינטגרציה? בחירה בכלי מתאים כדוגמת TeamCity, FinalBuilder או Jenkins (לשעבר Hudson) יעשו את העבודה. הכלים הללו יודעים לזהות ביצוע Commit במערכת ה - Source Control שלכם, למשוך את הקוד, לבצע Build ואפילו להריץ את כל (או חלק מ)בדיקות האוטומציה שלכם. המתקדמים ביניכם יוכלו לשלב גם Build לילי עם בדיקות מסיביות יותר כדוגמת בדיקות עומסים. רוצים להוציא מהם עוד? הכלים הללו גם יודעים לאתחל את בסיס הנתונים ואפילו לממש את השלב הבא: Continuous Deployment שיביא את הקוד שלכם ממש אל המשתמשים.
- Continuous Deployment: או בקיצור CD: מימשתם את התהליך שבודק את הקוד? נותנים שירות ללקוחות שלכם (לדוגמה מערכת SaaS)? פתרונות ה - CD לוקחים אתכם צעד אחד קדימה, ומתקינים את הקוד בשידור חי אצל המשתמשים שלכם. עם קצת מיומנות ובטחון תגיעו לעד עשרות התקנות בייצור ביום! הכלים שישמשו אתכם למהלך הם Selenium, BuildBot, Atlassian Bamboo, Chef ו - Puppet.
- Logging/Tracing/Analytics: מעלים את הקוד לייצור? רוצים לדעת מה הולך שמה? רוצים לראות איזה Exceptions עפו? במה משתמשים באמת משתמשים ובמה לא? מבחר כלים ואמצעים יעמדו לרשותכם:
Read more: הבלוג הפתוח למנהל הפיתוח
WPF 4: Using ObjectDataProvider for DataBinding
Posted by
jasper22
at
12:40
|
Windows Presentation Foundation (WPF) has provided many features for developing Data Driven applications. Using the DataBinding feature of WPF, effective data representation can easily be achieved. WPF allows developers to define an instance of the Data Access Object directly in XAML. ObjectDataProvider is one of the effective mechanisms for DataBinding in WPF. We use this provider if the application contains a separate Data Access Layer. ObjectDataProvider defines the instance of the class and makes call to the various methods of the class. Follow these steps to use the ObjectDataProvider for Databinding in WPF applications
Step 1: In VS 2010, create a WPF application and name it as ‘WPF40_Database’. To this application, add a new class file and name it as ‘DataAccessLayer.cs’. Write the following code in it:
Note: To reduce code, I haven’t used the try-catch-finally block but make sure you do that in your application. Alternatively use the using block to release resources, once they are used.
using System;
using System.Collections.ObjectModel;
using System.Data.SqlClient;
namespace WPF40_Database
{
public class ImageEmployee
{
public int EmpNo { get; set; }
public string EmpName { get; set; }
public int Salary { get; set; }
public int DeptNo { get; set; }
public byte[] EmpImage { get; set; }
}
public class CDataAccess
{
ObservableCollection<ImageEmployee> _EmpCollection;
public ObservableCollection<ImageEmployee> EmpCollection
{
get { return _EmpCollection; }
set { _EmpCollection = value; }
}
public CDataAccess()
{
_EmpCollection = new ObservableCollection<ImageEmployee>();
}
public ObservableCollection<ImageEmployee> GetEmployees()
{
SqlConnection conn =
new SqlConnection("Data Source=.;Initial Catalog=Company;" +
"Integrated Security=SSPI");
SqlCommand cmd = new SqlCommand();
conn.Open();
cmd.Connection = conn;
cmd.CommandText = "Select * from ImageEmployee";
The above code contains two classes ‘ImageEmployee’ - which defines properties compatible to the Table column and the class ‘CDataAccess’, with a method called ‘GetEmployees()’ which makes a call to the database and retrieves rows from the table. The code in this method stores all the data in an ObservableCollection<T>.
Step 2: Open MainWindow.xaml and write the following XAML code:
<Window x:Class="WPF40_Database.MainWindow"
xmlns:data="clr-namespace:WPF40_Database"
Title="MainWindow" Height="350" Width="912">
<Window.Resources>
<ObjectDataProvider x:Key="objDs"
ObjectType="{x:Type data:CDataAccess}"
MethodName="GetEmployees">
</ObjectDataProvider>
<DataTemplate x:Key="EmpDataTemplate">
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding EmpName}"></TextBlock>
<Image Source="{Binding EmpImage}" Height="60" Width="60"/>
</StackPanel>
</DataTemplate>
</Window.Resources>
<Grid DataContext="{Binding Source={StaticResource objDs}}">
<ComboBox Height="80" HorizontalAlignment="Left" Margin="29,30,0,0"
Name="lstEmployee" VerticalAlignment="Top" Width="274"
ItemsSource="{Binding}"
ItemTemplate="{StaticResource EmpDataTemplate}"
IsSynchronizedWithCurrentItem="True"/>
Read more: devCurry
Skype Challenger Releases Viber 2.0: Free Text Messages And More
Posted by
jasper22
at
12:39
|

Viber Media, the Israeli startup behind the Viber service, which lets iPhone users make free calls to each other, has released version 2.0 of its application in the App Store.
The company’s still gearing up for the launch of their Android application, but in the meantime the update to the iPhone app brings a couple of goodies, in particular the ability to text message other Viber users free of charge. Sure, there isn’t exactly a shortage of free messaging apps for the iPhone these days, but it’s always nice to have a free app that supports both voice calls and text messages.
Viber 2.0 comes with a new tab dedicated to ‘Messages’, where users can see all of their messages and from which they can send new ones to their contacts. Viber sends push notifications to users when they receive a text, so it’s potentially a replacement for SMS.
In addition, the ‘Contacts’ interface has been redesigned to let users filter their contacts and easily see which ones are already on on Viber, or just their favorites if they prefer.
Viber’s calling mechanism has also been improved. When users place a call, the app will first enter a ‘Calling’ state. Once the other party’s Viber app has been contacted and it begins ringing, Viber will enter a ‘Ringing’ state, letting the user know that there’s a connection.
Read more: TechCrunch
Google tightening control of Android, insisting licensees abide by 'non-fragmentation clauses'?
Posted by
jasper22
at
12:18
|
A storm seems to be brewing over the realm of Android development. Bloomberg's Businessweek spies have received word from "a dozen executives working at key companies in the Android ecosystem" that Google is actively working to gain control and final say over customizations of its popular mobile OS. That might not sound unreasonable, and indeed Google's public position on the matter is that it's seeking to stabilize the platform and ensure quality control, but it does mark a major shift from where Android started -- an open source OS that was also open to manufacturers and carriers to customize as they wish. Not so anymore, we're told, as apparently Mountain View is now demanding that content partnerships and OS tweaks get the blessing of Andy Rubin before proceeding. The alternative, of course, is to not be inside Google's warm and fuzzy early access program, but then, as evidenced by the company recently withholding the Honeycomb source code, you end up far behind those among your competitors who do dance to Google's pipe.
Read more: Engadget
How to send SMS in Windows Phone 7 using C# ?
Posted by
jasper22
at
11:19
|
The developers can make use of the SMSComposeTask launcher to send the SMS in Windows Phone 7 .
To send the SMS in Windows Phone 7 using SMSComposeTask by including the namespace Microsoft.Phone.Tasks
using Microsoft.Phone.Tasks;
The Microsoft.Phone.Tasks namespace includes the classes necessary for all the Launcher and Chooser tasks .
Now , create the instance of the SMSComposerTask and set its “To” field to a valid number and the “Body” property to a text to be sent .
private void button1_Click(object sender, RoutedEventArgs e)
{
SmsComposeTask composeSMS = new SmsComposeTask();
composeSMS.Body = " This is a Test SMS sent using SmsComposeTask launcher";
composeSMS.To = "1765432548";
composeSMS.Show();
}
The Show method will display the SMSComposer screen which requires the user interaction to send the SMS .
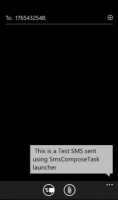
Read more: Ginktage
Itsy-OS Kernel: Premptive Switcher & Memory Manager
Posted by
jasper22
at
11:17
|
Itsy-OS v0.1 is a small 8086 operating system kernel providing two basic services: a simple preemptive task switcher and memory management. If necessary, simple IPC and task management can be added to provide the features of a traditional microkernel.
Weighing in at about 380 bytes, Itsy should port well to tiny architectures.
Memory Control Blocks
Each block of memory is preceded by a memory control block which is 32 bytes long. The blocks of memory are arranged as a circular linked list. The first memory control block resides in segment 050h.
org 0h
; -----------------------------
; [Master Memory Control Block]
; -----------------------------
M_NEXT:dw 0 ; address of next MCB
M_LAST:dw 0 ; address of previous MCB
M_PARA:dw 0 ; size of this block in paragraphs including MCB
M_FLAG:db 0 ; flags
db 0 ;
M_NPRO:dw 0 ; next MCB containing process, zero if not in queue
M_LPRO:dw 0 ; previous MCB containing process, or zero
M_STKP:dw 0 ; stack pointer
M_STKS:dw 0 ; stack segment
M_TIME:dw 0 ; time allocated / de-allocated
M_DATE:dw 0 ; date allocated / de-allocated
M_OWNR:dw 0 ; user number who owns this memory
M_NAME:db ' ' ; name of this memory block
; ---------------------------------------------------------------------------
; flag bits
F_FREE equ 01h ; zero if this is free memory
F_ACTI equ 02h ; zero if no task ready for CPU
; ---------------------------------------------------------------------------
jmp E_INIT ; setup kernel
; ---------------------------------------------------------------------------
; -------------------
; [Nucleus Data Area]
; -------------------
D_PCP:dw 0 ; segment of current process's MCB
D_SWEN:db 0 ; set to zero when switching disabled
Task Switcher
Read more: Retro Programming
Android HTTP Camera Live Preview Tutorial
Posted by
jasper22
at
11:15
|
The Android SDK includes a Camera class that is used to set image capture settings, start/stop preview, snap pictures, and retrieve frames for encoding for video. It is a handy abstraction of the real hardware device. However, the SDK doesn't provide any camera emulation, something that makes testing camera enabled applications quite difficult. In this tutorial, I am going to show you how to use a web camera in order to obtain a live camera preview.
What we are essentially going to do is setup a web camera to publish static images in a predefined URL and then grab these images from our application and present them as motion pictures. Note that this tutorial was inspired by a post named “Live Camera Previews in Android”.
Let's start by creating an Eclipse project under the name “AndroidHttpCameraProject” and an Activity named “MyCamAppActivity”. As a first step, I am going to show you how to use the SDK camera. You can find many resources on how to manipulate the camera, one of them being the CameraPreview class that is included in the SDK's samples. In short, we are going to extend the SurfaceView class and provide our implementation using the device's camera. Then, we use the setContentView method of our Activity in order to set the activity content to that specific View. Let's see what the two classes look like:
package com.javacodegeeks.android.camera;
import android.app.Activity;
import android.os.Bundle;
import android.view.SurfaceView;
import android.view.Window;
public class MyCamAppActivity extends Activity {
private SurfaceView cameraPreview;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
cameraPreview = new CameraPreview(this);
setContentView(cameraPreview);
}
}
Read more: Java code geeks
Why startups don't use .net
Posted by
jasper22
at
11:15
|
There have been several articles over the last couple of weeks about the usefulness of .net for startups. I will mention three:
* Did the Microsoft stack kill MySpace?
* Why we don't hire .net programmers
* Why Microsoft could kill your startup career
Even though our company is now several years old, our HQ is still located in an office facility that is mainly for startups. Well over the time we have had many companies, including Microsoft, Mozilla, IHTSDO and a few others, but most of the companies are startups. I recognize the same thing: I have not heard the word .net been used by any of the startups, yet. Would I hire a .net developer? I see no problem with .net, but also no significant value of having it on the resume. There are so many other parameters that are important.
I think the last article describes the problem best: Do large companies use their software engineers well enough? I think most have a problem here. A good software engineer is a serial decision maker, who writes down decisions about how things should be done in an automated way. Seen from the management POV, this is not a deterministic process, but a stochastic process, simply because the human mind is shaped by so many things, that influences the outcome. Many attempts have been made to improve the value generated by software developers, including agile software development processes, better ways to write specifications etc., but often these methods are confined within the software development department. If you want to get problems solved, for real, a larger part of the organization needs to be involved, thinking out-of-the-R&D-box must be allowed, and innovation must be rewarded.
Read more: Compas Pascal
How I store Enumerations in the Database
Posted by
jasper22
at
11:14
|
One of the things I come across in databases now and then is a collection of single tables with a name like "MessageType". You have a look in them and it turns out to have 6 or so rows with no foreign key relationships. Every single time it turns out to be someone had the idea to store an Enumeration (Enum) type in the database. Not a bad idea as it turns out since you can add sort options, soft deletes and the like, but the implementation of a single table for each one is flawed.
The following is how I deal with it (probably not ideal but works well for me). Essentially you define two tables in the database with names like Lookup and Value. Inside lookup you have something similar to the following.
+------+
|id |
|lookup|
|name |
+------+
This is basicly a representation of the enum name. Id is usually an autoincrementing id to make joins easy while lookup is the primary key. This is the definition of the enum, IE the name part in the database, or in our example "Message".
Then you add the enum values to your Values table which looks similar to the below,
+---------+
|id |
|lookupid |
|name |
|sortorder|
|deleted |
+---------+
Then through the power of a simple join you can get your enum values,
Read more: Search [co.de]
Use Python to write plug-ins for GIMP
Posted by
jasper22
at
11:06
|
This article is about using GIMP-Python, which is a set of Python modules that allow you to do programming in Python to automate commands in GNU Image Manipulation Program (GIMP). These Python modules are wrappers around the libgimp libraries. GIMP-Python is different from the Script-Fu extensions. In Script-Fu, a plug-in is used to execute scripts. In GIMP-Python, the Python script takes center stage and does the work. You can instantiate the GIMP-Python scripts from inside GIMP itself, or you can use GIMP's batch mode to start it from the command line.
In this article, you learn how to write Python code that allows you to automate two different tasks in GIMP: resizing images and saving them as different formats.
You can install and use both GIMP and Python on many different platforms, including Linux®, Mac OS® X, and Microsoft® Windows®. The cross-platform nature of both GIMP and Python means you can write complex plug-ins for GIMP using Python and be able to run them on a variety of platforms.
Overview of GIMP
GIMP is an open source image manipulation program that many people use as a viable alternative to some of the commercial offerings. GIMP handles complicated features such as layers and paths. GIMP supports a number of different image formats and comes with relatively complex filters. GIMP has strong community support and involvement, so it is usually easy to find information about how to use or extend GIMP.
See Resources for the link to download and install GIMP on your computer.
Overview of Python scripting
Python is an object-oriented scripting language that allows you to write code that runs on many different platforms. Python has been ported to both the .NET and Java™ virtual machines, so there are many different ways that you can execute Python. See Resources to learn how to install Python on your computer.
Many modules exist for Python that provide functionality you can reuse without writing your own (the GIMP-Python modules are an example). An index of the Python modules lists many different pre-built modules that you can use to do a variety of tasks from dealing with Hypertext Markup Language (HTML) and Hypertext Transfer Protocol (HTTP) connections to working with Extensible Markup Language (XML) files (see Resources). You can also build your own Python modules, allowing you to reuse parts of code within your enterprise.
Similar to GIMP, Python also has significant community support. This means that you can find information, as well as download and use relatively mature tools that help you in your Python development.
Before proceeding to the rest of the article, install Python on your operating system according to the instructions on Python's site. Make sure you have Python correctly installed by opening a command prompt and typing python --version. The results should look something like those in Listing 1.
Listing 1. Verifying the installation of Python
$ python --version
Python 2.6.6
After you install the Python interpreter, you can create Python files in any text editor and run them with the interpreter. You can also use the PyDev plug-in for Eclipse, which offers syntax highlighting as well as some other features, such as catching syntax errors for you. Another option is to use the Python console directly in Eclipse, which is convenient for finding help.
Read more: IBM
OpenSSH-LPK
Posted by
jasper22
at
09:54
|
The OpenSSH LDAP Public Key patch provides an easy way of centralizing strong user authentication by using an LDAP server for retrieving public keys instead of ~/.ssh/authorized_keys.
Read more: Google code
Building WPF and Silverlight Applications with a Single Code Base Using Prism
Posted by
jasper22
at
09:54
|
Contents
Introduction
Creating a Silverlight Version of Prism
Creating Project Linker
Test-Driven Development on Silverlight
Architecting Your Application for Multi-Targeting
Identifying What Can Easily be Multi-Targeted
Separated Presentation
Building Platform-Specific Services
Avoid Inconsistencies and Try to Keep a Single Code Base
Accommodate for Different Platform Capabilities
Conditional Compilation
Partial Classes
Caveats
Summary
Creating a Silverlight Version of Prism
Creating Project Linker
Test-Driven Development on Silverlight
Architecting Your Application for Multi-Targeting
Identifying What Can Easily be Multi-Targeted
Separated Presentation
Building Platform-Specific Services
Avoid Inconsistencies and Try to Keep a Single Code Base
Accommodate for Different Platform Capabilities
Conditional Compilation
Partial Classes
Caveats
Summary
Composite Application Guidance for WPF and Silverlight, also affectionately known as Prism v2, has been out for several months now. One of the areas Prism provides guidance on is the ability to target your application to both Windows Presentation Foundation (WPF) and Silverlight. It's interesting that, initially, this part of our guidance met quite a bit of resistance. Why were we focusing on multi-targeting for the first couple of iterations when we could be spending our time giving guidance on composition? But since the release of the Prism v2 project, we've found that a lot of our customers really love this part of the guidance. They especially like the Project Linker tool we built to help multi-targeting; as basic as it is, the tool has been well received and is being used in ways we couldn't have imagined.
So let's look at the approach we took for writing multi-targeted applications and how Prism can help you do the same.
Introduction
When we started work on Prism v2, in August 2008, we knew we wanted to support both WPF and Silverlight. Silverlight makes big strides toward closing the gap between the power of a rich-client application and the reach and ease of deployment of a Web application. The first version of Prism targets only WPF, but since WPF and Silverlight are very similar, we knew it wouldn't be too hard to create a Silverlight version. And since Prism helps in writing loosely coupled, modular applications, we felt that Prism could also help in allowing you to write applications that target both WPF and Silverlight from a single code base. The challenge is, of course, that although WPF and Silverlight are similar, they are not binary-compatible. The API and XAML language itself also has minor differences that make it harder to multi-target.
What Is Multi-Targeting and Why Should You Care?
Multi-targeting is the ability to target multiple platforms from a single code base. In this case, we are talking about targeting both the normal desktop version of the Microsoft .NET Framework version 3.5, which contains WPF and the Silverlight version of the .NET Framework.
So why should you care about this capability? The most obvious reason would be that you would like to take advantage of the strengths of WPF and Silverlight. In WPF, you can build applications that make full use of the client platform and interact with existing applications, such as Microsoft Office. It's also a lot easier to reuse existing assets, such as through COM interop or Windows Forms interop.
Whereas WPF gives you more capabilities, Silverlight gives you a much broader reach, because it runs on multiple platforms. It also runs in a protected sandbox, so end users can safely install Silverlight without needing administrative privileges. You don't need to completely multi-target your application to make it worthwhile. For example, you might expose small parts of internal applications over the Internet to give customers the ability to view and change some of their own information.
Creating a Silverlight Version of Prism
Even though we felt that multi-targeting would be a valuable capability for our customers, we also had a somewhat selfish reason for creating it. We wanted to build a Silverlight version of Prism, but we didn't want the overhead of maintaining two code bases. Because we didn't know how much code we would be able to reuse, we did a couple of spikes. A spike (in "agile" terminology) is a time-boxed exploration that allows you to learn more about a problem domain so that you can make a more valid estimation. So we did a couple of spikes to see how much of the Prism v1 code base we could migrate to Silverlight and how hard it would be to port our code to Silverlight. The conclusion was fascinating. We estimated that we could reuse around 80 percent of the code in the Prism library without modifying it.
Read more: MSDN
How can I debug WPF bindings?
Data Binding can be tricky to debug. In this post, I will share the techniques I use to debug WPF bindings, including the new debugging improvements we implemented in the latest 3.5 release. I will discuss the following four techniques:
Scanning Visual Studio’s Output window for errors.
Using Trace Sources for maximum control.
Using the new property introduced in WPF 3.5 PresentationTraceSources.TraceLevel.
Adding a breakpoint to a Converter.
The DataContext of this app is set to a new instance of Star, which is a class that contains two properties: Picture and Caption. Picture is of type BitmapImage and contains an image of the sun, which I included in the project. Caption is a string property that takes a while to be initialized (more details about this later).
Output Window
In the XAML of this application, I have an Image whose Source is data bound to an incorrect property name. This is a simple scenario that causes a Binding to fail.
<Image Source="{Binding Path=PictureWrong}" Width="300" Height="300" Grid.Row="0"/>
Every time a Binding fails, the binding engine prints an informative message in the Output window of Visual Studio. In this case, I get the following message:
System.Windows.Data Error: 35 : BindingExpression path error: ‘PictureWrong’ property not found on ‘object’ ”Star’ (HashCode=49535530)’. BindingExpression:Path=PictureWrong; DataItem=’Star’ (HashCode=49535530); target element is ‘Image’ (Name=”); target property is ‘Source’ (type ‘ImageSource’)
This message should give you enough information to quickly figure out the mistake in the property name.
Advantage of this technique:
It is very easy to look at the Output window, and in most cases it’s sufficient. It should be the first approach you consider when you have a problem with your bindings.
Disadvantage of this technique:
Most real world applications print so much information to the Output window that it may be hard to find the error you’re looking for.
Trace Sources
The Trace Sources solution was already around in WPF 3.0. It adds a lot more flexibility to the previous solution by allowing you to control the level of detail you care about, where you want that messages to be printed and the WPF feature you want to debug.
The Trace Sources solution relies on a config file for the configuration of its behavior – the App.config file. Here is a portion of the contents of that file:
<configuration>
<system.diagnostics>
<sources>
<source name="System.Windows.Data" switchName="SourceSwitch" >
<listeners>
<add name="textListener" />
</listeners>
</source>
…
<switches>
…
<add name="SourceSwitch" value="All" />
</switches>
<sharedListeners>
Read more: Bea Stollnitz
Thread safe observable collection
Posted by
jasper22
at
16:57
|
If you ever wrote something more, then standard UI input-output, you sure got into wide range of exceptions like “The calling thread cannot access this object because a different thread owns it” or “This type of CollectionView does not support changes to its SourceCollection from a thread different from the Dispatcher thread”. What the problem? The problem is old like condom in my wallet world-old, the try to get into resources from different threads. Let me show you some code. Very-very bad code. It’s unbelievable, that this code can ever work.
Let’s write Thread Safe Observable Collection, that knows, that it might be called from big number of threads and take care on itself. Sounds good? Let’s start.
First of all we’ll try to get current STAThread (UI) dispatcher. How to do it? Simple. The only place we’ll initialize our collection is in XAML, so default constructor will be called from the thread we need. Now, we save it
Dispatcher _dispatcher;
public ThreadSafeObservableCollection()
{
_dispatcher = Dispatcher.CurrentDispatcher;
}
The next step is to override all vital methods of our collection to be invoked from this thread.
protected override void ClearItems()
{
if (_dispatcher.CheckAccess())
{
base.ClearItems();
}
else
{
_dispatcher.Invoke(DispatcherPriority.DataBind, (SendOrPostCallback)delegate { Clear(); });
}
}
Let’s understand it. First we’re checking if the current thread can access the instance of the object, if it can – we’ll just do our work, but if not, we’ll invoke it in the context of the current thread by sending anonymous delegate with or without parameters.
Why I’m casting the anonymous delegate into SendOrPostCallback? The answer is simple – look into Reflector. You can not implement your delegate with parameter and without return value better
Read more: Tamir Khason – Just code
Why should I use ObjectDataProvider?
Posted by
jasper22
at
16:23
|
There are many ways to instantiate an object that will be used as the data source for bindings. I have seen many people create the object in code and set the DataContext of the Window to that instance, which is a good way to do this. You may have noticed that I have been adding the source object to the Window’s Resource Dictionary in most of my previous posts, which works well too. We have an ObjectDataProvider class in data binding that can also be used to instantiate your source object in XAML. I will explain in this post the differences between adding the source object directly to the resources and using ObjectDataProvider. This will hopefully give you guidance on how to evaluate your scenario and decide on the best solution.
As I describe these features, I will walk you through building a little application that allows people to type their weight on Earth and calculates their weight on Jupiter.
When adding the source object directly to the resources, the Avalon data binding engine calls the default constructor for that type. The instance is then added to a dictionary of resources, using the key specified by x:Key. Here is an example of the markup for this solution:
<Window.Resources>
<local:MySource x:Key="source" />
(…)
</Window.Resources>
As an alternative, you can add an ObjectDataProvider to the resources and use that as the source of your bindings. ObjectDataProvider is a class that wraps your source object to provide some extra functionality. I will discuss here the following distinguishing capabilities of ObjectDataProvider:
Passing parameters to the constructor
Binding to a method (which may take parameters)
Replacing the source object
Creating the source object asynchronously
Passing parameters to the constructor
When adding the source object to the resources directly, Avalon always calls the default constructor for that type. It may happen that you have no control over the source data, and the class you’re given has no default constructor. In that case, you can still create an instance in XAML by using ObjectDataProvider in the following way:
<ObjectDataProvider ObjectType="{x:Type local:MySource}" x:Key="odp1">
<ObjectDataProvider.ConstructorParameters>
<system:String>Jupiter</system:String>
</ObjectDataProvider.ConstructorParameters>
</ObjectDataProvider>
This markup creates a new instance of MySource by calling its constructor and passing the string “Jupiter”. It also creates an instance of ObjectDataProvider, which will wrap the MySource object.
MySource has a public property called “Planet” that exposes an actual Planet object – the one whose name matches the string passed in the constructor parameter (in this case, “Jupiter”). I want to have a title Label in my application that binds to the Planet’s Name property. Binding to subproperties can be done in Avalon by using “dot notation”. The syntax for this is Path=Property.SubProperty, as you can see in the following markup:
<Label Content="{Binding Source={StaticResource odp1}, Path=Planet.Name}" Grid.ColumnSpan="2" HorizontalAlignment="Center" FontWeight="Bold" Foreground="IndianRed" FontSize="13" Margin="5,5,5,15"/>
You may be looking at this binding statement and thinking that it makes no sense. It seems like we are binding to the Name subproperty of the Planet property of ObjectDataProvider. But I just mentioned that MySource is the one that has a Planet property, not ObjectDataProvider. The reason this markup works is that the binding engine treats ObjectDataProvider specially, by applying the Path to the source object that is being wrapped. Note that this special treatment is also used when binding to XmlDataProvider and CollectionViewSource.
Binding to a method
There is a method in MySource that takes as a parameter a person’s weight on Earth and calculates that person’s weight on the planet passed to the constructor. I want to pass some weight to this method in XAML and bind to its result. This can also be done with ObjectDataProvider:
<ObjectDataProvider ObjectInstance="{StaticResource odp1}" MethodName="WeightOnPlanet" x:Key="odp2">
<ObjectDataProvider.MethodParameters>
<system:Double>95</system:Double>
</ObjectDataProvider.MethodParameters>
</ObjectDataProvider>
Read more: Bea Stollnitz
StaticResource, DynamicResource, x:Static – what’s all this about?
Posted by
jasper22
at
12:42
|
So, you know to write very complicated things in XAML code, but, really, do you know what’s the difference between static and dynamic resource? Do you know what’s really x:Static means? We’ll try today to understand differences and things we can do with this stuff. All those are markup extensions. Let’s start from the very beginning – MSDN
A markup extension can be implemented to provide values for properties in attribute syntax, properties in property element syntax, or both.
StaticResource – Provides a value for a XAML property by substituting the value of an already defined resource
DynamicResource - Provides a value for a XAML property by deferring that value to be a runtime reference to a resource. A dynamic resource reference forces a new lookup each time that such a resource is accessed.
I can not tell more clear then this statement. If you want to reuse something already exists – use StaticResource, if it’s changing – DynamicResource. So StaticResource is restrictive resource, when DynamicResource is not. So, other words, if you need only properties to be changed, use StaticResource, if whole object changing – use DynamicResource . The best example for this is following code
<TextBlock Text="This is Active caption color" Background="{DynamicResource {x:Static SystemColors.HighlightBrushKey}}"/>
<TextBlock Text="This is not Active caption color" Background="{DynamicResource {x:Static SystemColors.HighlightBrushKey}}">
<TextBlock.Resources>
<SolidColorBrush x:Key="{x:Static SystemColors.HighlightBrushKey}" Color="Yellow" />
</TextBlock.Resources>
</TextBlock>
<TextBlock Text="This is Active caption color" Background="{StaticResource {x:Static SystemColors.HighlightBrushKey}}"/>
<TextBlock Text="This is also Active caption color" Background="{StaticResource {x:Static SystemColors.HighlightBrushKey}}">
<TextBlock.Resources>
<SolidColorBrush x:Key="{x:Static SystemColors.HighlightBrushKey}" Color="Yellow" />
</TextBlock.Resources>
</TextBlock>
The result is following
..
As you can see, I completely change HighlightBrush object by using local resources. This works if the resource set as dynamic, but wont if static.
That’s the reason, that you can not bind to DynamicResource, only to StaticResource properties or method results. Data binding engine can not be in peace with changing objects.
So, what is x:Static? It’s XAML-defined Markup extension. There are some of them x:Type, x:Static x:Null and x:Array.
x:Static – Produces static values from value-type code entities that are not directly the type of a property’s value, but can be evaluated to that type
Read more: Tamir Khason – Just code
15 Must Know Java Interview Questions After 2 Years of Experience
Posted by
jasper22
at
10:32
|
Interview Questions that every developer should have the answer
Enterprise Java Application development is growing every day and new features being introduced but the the beginners have always start from the basics. The questions listed below are what in general a Java developer should be able to answer after 2 years of experience. (Assuming no prior exposure to Java)
Core Java
1) What is the purpose of serialization?
2) What is the difference between JDK and JRE?
3) What is the difference between equals and ==?
4) When will you use Comparator and Comparable interfaces?
5) What is the wait/notify mechanism?
6) What is the difference between checked and unchecked exceptions?
7) What is the difference between final, finally and finalize?
JEE
8) What is the difference between web server and app server?
9) Explain the Struts1/Struts2/MVC application architecture?
10) What is the difference between forward and sendredirect?
General
11) How does a 3 tier application differ from a 2 tier one?
12) How does the version control process works?
13) What is the difference between JAR and WAR files?
Read more: Extreme Java
Advanced Animation: Animating 15,000 Visuals in Silverlight
Posted by
jasper22
at
10:29
|
In my last post I showed a quick summary of a graphic-intensive dashboard that I helped out with. I also mentioned that there are a lot of posts out there dogging SL and WPF rendering performance. But if we get creative with some of the built-in controls SL has to offer we can get some pretty good drawing perf. I wanted to elaborate on some of the techniques I used to eek-out every bit of perf I could. The main concepts I want to discuss are:
Procedural Animations
Writeable Bitmaps
Image Blitting
Out of the box, Silverlight and WPF have Storyboards and Timelines to animate objects on the screen. Storyboards, however, quickly break down once you need to draw more than ~50 items on a screen at once. Storyboards are great for quick animation (slide-in, slide-out, etc.), but for intense data visualizations we’ll have to get a little closer to the metal.
Procedural Animations
Procedural animations are most commonly found in video games and are used to create complex visual effects. Things like simulating particle systems, physics-based animations, and sprite animations. But that doesn’t mean we can’t spicy up our everyday-software with some of the same concepts.
They way most procedural animations are implemented are with a simple a Setup and Update, Draw loop.
In Silverlight we can setup our animations by hooking in to the CompositionTarget.Rendering event and then call ‘Update’ and ‘Draw’ each time it ticks. If you’ve ever done any game programming for SL or WPF this should look very familiar as it resembles a typical game ticker.
Here is a sample project you can download to wrap your head around how procedural animations in Silverlight.
Read more: Clarity Consulting
How to create your first SQL Server Integration Services (SSIS) package part-1
Posted by
jasper22
at
10:20
|
Table of Contents
Introduction
What is SSIS package?
Features
SSIS Import/Export Wizard
Creating or maintaining SSIS packages
Features of the data flow task
How to create a simple SSIS package
References
Conclusion
History
Introduction
SQL Server Integration Services (SSIS) is an excellent component with some great features of Microsoft SQL Server 2005 & 2008 edition as well. Today’s business are completely depends on the data which are comes thru user / customers. Business analyst analysis the data to figure out the market. So not only in business sector but also with the other areas; data are very much important.
So, if we think for a small single domain business, it could be easy to collect the business transactional data by using any data migration tools. But if it’s in a large domain or even multiple domains then how you centralized all the data’s; data centralization is very common and essential requirement for the business people’s where business become globalizing day by day.
In this article, we will discuss about Microsoft SSIS package, how to create SSIS package for smooth data migration, design SSIS package for single domain, design SSIS package for multiple domains and some other features i.e., SSIS Import/Export etc.
What is SSIS Package?
By definition, SQL Server Integration Services (SSIS) is a component of the Microsoft SQL Server database software which can be used to perform a broad range of data migration tasks.
SSIS is a platform for data integration and workflow applications. It features a fast and flexible data warehousing tool used for data extraction, transformation, and loading (ETL). The tool may also be used to automate maintenance of SQL Server databases and updates to multidimensional cube data.
Features
SQL Server Integration Services (SSIS) has various useful features, for example SSIS import/export wizard, creating / maintaining SSIS package using Microsoft visual development IDE /tools etc. Let’s try to understand few more on the above features:
SSIS Import/Export Wizard:
This wizard helps you to create SSIS package very quickly. For example we want to move data from a single data source to another destination, note that we can do this without transformations and wizard almost seems to Microsoft SQL Server Import / Export wizard.
Creating or maintaining SSIS packages:
SQL Server Business Intelligence Development Studio (BIDS). allow users to create / edit SSIS packages using a drag-and-drop user interface.. BIDS is very user friendly and allow you to drag-and-drop functionalities. Variety of elements that define a workflow in a single package. Upon package execution, the tool provides color-coded, real-time monitoring.
Read more: Codeproject
Fallout Mod Studio - A new mod manager for Fallout 3 and Fallout: New Vegas
Posted by
jasper22
at
10:19
|
Project Description
FOMS provides a simple, graphical, and highly useful approach to installing and managing modifications for Fallout 3 and Fallout New Vegas.
It utilizes XML templates to quickly sort mods, and offer solutions to missing dependencies, conflicting mods, missing patches, and more.
Read more: Codeplex
Subscribe to:
Posts (Atom)