Microsoft Patents OS Shutdown
You would think that shutting down software could be fairly simple from an end user's view. If I ask you to shut it down, would you mind shutting it actually down, please? Well, it's a bit more complicated than that, because you need to ask the user if they really want to shut down and if unsaved documents should be saved. And that warrants a patent that also covers Mac OS X. Next time you shut down Windows, remember how complicated it is for Windows to shut down. Perhaps that is the reason why this procedure can take minutes in some cases. Read more: Slashdot
Collect your C++ Garbage with Boost
Posted by
jasper22
at
10:16
|
C++ do not have automatic garbage collection built in like Java, .NET languages as well as many others. This has its pros and cons since the gained freedom will lead to potential memory leaks and a lot of extra code to deal with clean up.
Using the boost library, specifically the smart pointers provided, most of the memory handeling burden can be lift of the programmes shoulders.
In the boost library, there is an object, shared_ptr, that you can use to wrap all your pointers to which you have allocated memory. The shared pointer will keep track of all references to the very object, and once the final pointer to the objects gets out of scope or deleted, then your object will be freed automatically.
Here is some code that shows how to use boost::shared_ptr together with STL containers. Note that no "delete" keyword is used in the code.
#include <boost/shared_ptr.hpp>
#include <iostream>
#include <vector> // Just a simple class
class Foo
Using the boost library, specifically the smart pointers provided, most of the memory handeling burden can be lift of the programmes shoulders.
In the boost library, there is an object, shared_ptr, that you can use to wrap all your pointers to which you have allocated memory. The shared pointer will keep track of all references to the very object, and once the final pointer to the objects gets out of scope or deleted, then your object will be freed automatically.
Here is some code that shows how to use boost::shared_ptr together with STL containers. Note that no "delete" keyword is used in the code.
#include <boost/shared_ptr.hpp>
#include <iostream>
#include <vector> // Just a simple class
class Foo
{
public:
Foo(){ }~Foo(){
std::cout << "Destructing. " << (int)this << std::endl;
}
}; // Make shorthand to cut some typing
typedef boost::shared_ptr<Foo> FooPtr; // this functions allocates memory for an object, return a shared_ptr to it and forgets about it.
FooPtr CreateFoo()
typedef boost::shared_ptr<Foo> FooPtr; // this functions allocates memory for an object, return a shared_ptr to it and forgets about it.
FooPtr CreateFoo()
{
FooPtr fp(new Foo);
return fp;
} int main(int argc, char** argv)
FooPtr fp(new Foo);
return fp;
} int main(int argc, char** argv)
{
std::vector<FooPtr> foos; foos.push_back(CreateFoo());
foos.push_back(CreateFoo()); FooPtr temp = foos[1]; // extract the second object
std::cout << "clearing vector.." << std::endl;
foos.clear(); // the first object will be deleted here, but not the second, since it has a reference still in scope.
std::cout << "ending program.." << std::endl;
// main returns and the final object goes out of scope and is automatically destroyed.
return 0;
std::vector<FooPtr> foos; foos.push_back(CreateFoo());
foos.push_back(CreateFoo()); FooPtr temp = foos[1]; // extract the second object
std::cout << "clearing vector.." << std::endl;
foos.clear(); // the first object will be deleted here, but not the second, since it has a reference still in scope.
std::cout << "ending program.." << std::endl;
// main returns and the final object goes out of scope and is automatically destroyed.
return 0;
}Read more: Libzter
Linux boot process : code commentary
Posted by
jasper22
at
10:14
|
Abstract:
Booting an operating system has always been considered a challenging task. In this document we will take a look at the different aspects of the boot process. Such as the BIOS which is the first code which runs, the boot loaders that can load different operating systems, pass arguments to the kernel, load it from different sources like a hard drive, a flash, and network & finally the kernel itself. Though loading the kernel & setting it up to execute is not all that is to be done, we need to bring the system up with different user specific configurations. We will look at the scripts, which deal with this.
Linux has grown from a system that used to boot from a floppy providing no luxurious features to the user, to the current jazzy Linux systems. It is important to have an insight of the Linux boot procedure. Say for Linux to serve the purpose on embedded systems, the generic boot procedure must almost always be modified to meet the needs of the target application. Introduction:The moment after a computer is powered on, the systems memory is empty, the processor has no clue as to where it is & what it is supposed to execute. To see us through this situation a special hardware circuit raises the logical value of the RESET pin of the CPU. After RESET is thus asserted, some registers of the processor are set to fixed values, and the code found at physical address 0xFFFF FFF0, which is system BIOS, is executed. The BIOS does the POST ,some initialization & loads the boot sector from the boot device. The code that sits in the MBR is the boot loader; it gives us the ability to load multiple operating systems. The next in this process is the kernel itself, but its not as straight as it looks there are a few critical steps, which we will see in details a bit later. After the kernel is up and running it needs to mount the root file system. Say if the file system driver compiled as module then we won’t be able to directly mount the real root file system. To get over this we use an initrd images, which has the modules & a “linuxrc “ which can be a script or a binary. This will mount the real root file system & run init process. The init process reads the scripts & starts all the services according to the configuration. This is the final step in the boot process, now our system is all set to go..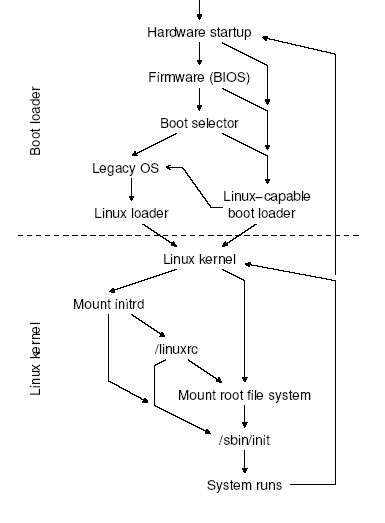
Read more: Milind Arun Choudhary
Booting an operating system has always been considered a challenging task. In this document we will take a look at the different aspects of the boot process. Such as the BIOS which is the first code which runs, the boot loaders that can load different operating systems, pass arguments to the kernel, load it from different sources like a hard drive, a flash, and network & finally the kernel itself. Though loading the kernel & setting it up to execute is not all that is to be done, we need to bring the system up with different user specific configurations. We will look at the scripts, which deal with this.
Linux has grown from a system that used to boot from a floppy providing no luxurious features to the user, to the current jazzy Linux systems. It is important to have an insight of the Linux boot procedure. Say for Linux to serve the purpose on embedded systems, the generic boot procedure must almost always be modified to meet the needs of the target application. Introduction:The moment after a computer is powered on, the systems memory is empty, the processor has no clue as to where it is & what it is supposed to execute. To see us through this situation a special hardware circuit raises the logical value of the RESET pin of the CPU. After RESET is thus asserted, some registers of the processor are set to fixed values, and the code found at physical address 0xFFFF FFF0, which is system BIOS, is executed. The BIOS does the POST ,some initialization & loads the boot sector from the boot device. The code that sits in the MBR is the boot loader; it gives us the ability to load multiple operating systems. The next in this process is the kernel itself, but its not as straight as it looks there are a few critical steps, which we will see in details a bit later. After the kernel is up and running it needs to mount the root file system. Say if the file system driver compiled as module then we won’t be able to directly mount the real root file system. To get over this we use an initrd images, which has the modules & a “linuxrc “ which can be a script or a binary. This will mount the real root file system & run init process. The init process reads the scripts & starts all the services according to the configuration. This is the final step in the boot process, now our system is all set to go..
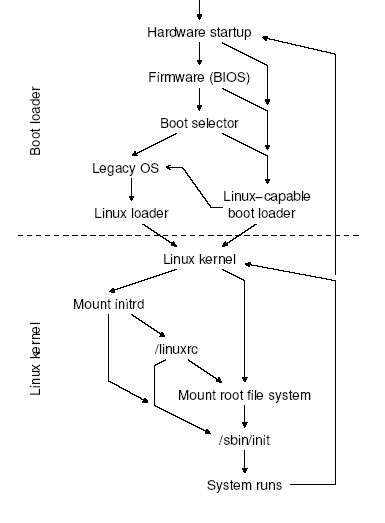
Read more: Milind Arun Choudhary
How to set up your own private Git server on Linux
Posted by
jasper22
at
10:13
|
Update 2: as pointed out by Tim Huegdon, several comments on a Hacker News thread pointing here, and the excellent Pro Git book, Gitolite seems to be a better solution for multi-user hosted Git than Gitosis. I particularly like the branch–level permissions aspect, and what that means for business teams. I’ve left the original article intact. Update: the ever–vigilant Mike West has pointed out that my instructions for permissions and git checkout were slightly askew. These errors have been rectified.One of the things I’m attempting to achieve this year is simplifying my life somewhat. Given how much of my life revolves around technology, a large part of this will be consolidating the various services I consume (and often pay for). The mention of payment is important, as up until now I’ve been paying the awesome GitHub for their basic plan. I don’t have many private repositories with them, and all of them are strictly private code (this blog; Amanda’s blog templates and styles; and some other bits) which don’t require collaborators. For this reason, paying money to GitHub (awesome though they may be) seemed wasteful. So I decided to move all my private repositories to my own server. This is how I did it.Set up the serverThese instructions were performed on a Debian 5 “Lenny” box, so assume them to be the same on Ubuntu. Substitute the package installation commands as required if you’re on an alternative distribution. First, if you haven’t done so already, add your public key to the server:ssh myuser@server.com mkdir .ssh
scp ~/.ssh/id_rsa.pub myuser@server.com:.ssh/authorized_keys Read more: Bradley Wright
scp ~/.ssh/id_rsa.pub myuser@server.com:.ssh/authorized_keys Read more: Bradley Wright
SELECT, Deconstructed
Posted by
jasper22
at
10:13
|
Today let’s expand on the logical processing order of SELECT that I mentioned in last week’s N Things Worth Knowing About SELECT blog.We’re looking at the SELECT statement clauses in the order that the SQL engine logically processes them…we’ll even write it that way – it’ll look weird, but we’ll be reading it like the SQL engine does. You can find more on SELECT’s logical processing order in BOL, and in Itzik Ben-Gan’s T-SQL Fundamentals and T-SQL Programming books.Here’s our basic T-SQL query (using AdventureWorks):SELECT P.Name ,
P.ProductNumber ,
P.Color ,
P.StandardCost ,
SC.Name [Category]
FROM Production.Product P
LEFT OUTER JOIN Production.ProductSubCategory SC ON P.ProductSubCategoryID = SC.ProductSubCategoryID
WHERE P.ProductSubCategoryID IS NOT NULL If we get all pseudo-mathematical on this, we can say that there’s some theoretical set of rows that we’ll get back out of this query; each logical step – in order – further refines that set of rows until we get back the actual rowset we want. FROMThe SQL engine starts with the FROM clause first, to see where the data’s coming from. In this case we’re pulling from the Product table…easy. So, the theoretical rowset right now is everything in the Product table. (You can think about each step passing on that theoretical rowset to the next step for further refinement.) -- Logical order:FROM Production.Product PON/JOIN
(more..)Read more: SQL Server Pedia
P.ProductNumber ,
P.Color ,
P.StandardCost ,
SC.Name [Category]
FROM Production.Product P
LEFT OUTER JOIN Production.ProductSubCategory SC ON P.ProductSubCategoryID = SC.ProductSubCategoryID
WHERE P.ProductSubCategoryID IS NOT NULL If we get all pseudo-mathematical on this, we can say that there’s some theoretical set of rows that we’ll get back out of this query; each logical step – in order – further refines that set of rows until we get back the actual rowset we want. FROMThe SQL engine starts with the FROM clause first, to see where the data’s coming from. In this case we’re pulling from the Product table…easy. So, the theoretical rowset right now is everything in the Product table. (You can think about each step passing on that theoretical rowset to the next step for further refinement.) -- Logical order:FROM Production.Product PON/JOIN
(more..)Read more: SQL Server Pedia
Enterprise Library 5 developer guide
Posted by
jasper22
at
10:12
|
Enterprise Library is a great addition to any .NET development project. The package contains hands-on labs and technical documentation. Now there is additional documentation available in several formats. The developer guide contains text, code snippets and diagrams, which makes it a great learning resource. Check it out here: http://msdn.microsoft.com/en-us/library/ff953181(PandP.50).aspx (more..)Read more: Pieter's Blog on Enterprise Development
Chapter 1 - Welcome to the Library
Chapter 2 - Much ADO about Data Access
Chapter 3 - Error Management Made Exceptionally Easy
Chapter 4 - As Easy As Falling Off a Log
Chapter 5 - A Cache Advance for your Applications
NHibernate Mapping Generator
Posted by
jasper22
at
10:10
|
Release Notes
Before installation this version you must uninstall your previous version of NMG.- Added partial support for composite id's. Only thing that is missing is the override for Equals() and GetHashCode().
- From what I can see, SQL Server 2000, 2005 and 2008 are supported. Although, this could use a little more testing.
- Models will now always be singular.
- Added support for one-to-many (HasMany) for both Oracle and SQL Server.
- One-to-many relationships will now have the property name pluralized (for example: public virtual IList<Order> Orders { get; set; } )
- Nullable fields will now have a nullable type if one is available.
- Added experimental support for Castle Active Record mappings.
- For Fluent NH, if the primary key is a string, we will use GeneratedBy.Assigned() instead of GeneratedBy.Identity() (only works for numbers).
- Lastly, from my testing, the application generates models far faster on large databases. I tested it on a legacy database with 300 tables with several (100) tables having 60 fields. The generation took nothing more than what I recall 1-2 minutes.
- Should now work with .NET 3.5For the most part it should generate correct HBM and Fluent mappings. Please let us know of any bugs you encounter.Read more: Codeplex
Before installation this version you must uninstall your previous version of NMG.- Added partial support for composite id's. Only thing that is missing is the override for Equals() and GetHashCode().
- From what I can see, SQL Server 2000, 2005 and 2008 are supported. Although, this could use a little more testing.
- Models will now always be singular.
- Added support for one-to-many (HasMany) for both Oracle and SQL Server.
- One-to-many relationships will now have the property name pluralized (for example: public virtual IList<Order> Orders { get; set; } )
- Nullable fields will now have a nullable type if one is available.
- Added experimental support for Castle Active Record mappings.
- For Fluent NH, if the primary key is a string, we will use GeneratedBy.Assigned() instead of GeneratedBy.Identity() (only works for numbers).
- Lastly, from my testing, the application generates models far faster on large databases. I tested it on a legacy database with 300 tables with several (100) tables having 60 fields. The generation took nothing more than what I recall 1-2 minutes.
- Should now work with .NET 3.5For the most part it should generate correct HBM and Fluent mappings. Please let us know of any bugs you encounter.Read more: Codeplex
NHibernate Query Analyzer
Posted by
jasper22
at
10:09
|
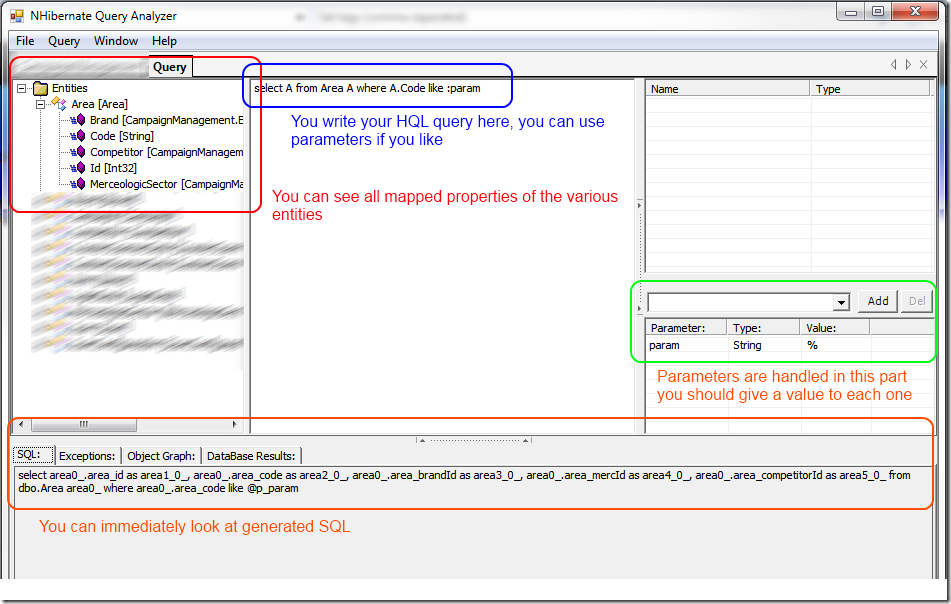
NHQueryAnalyzer is a tool originally written by Ayende, and now it is supported at this link. I think that it is really a good tool, very useful even to learn HQL query because gives you an immediate idea of what the query will looks like. Here is a screenshot. (‘I’ve hide some sensitive part because this is part of a project of a company I worked with). Read more: Alkampfer's Place
VoIP SIP SDK 3.4 released
Posted by
jasper22
at
10:08
|
VoIP SIP SDK provides a powerful and highly customizable solution to quickly add SIP based dial and receive phone calls features in your software applications. It accelerates the development of SIP compliant soft phone with a fully-customizable user interface and brand name. VoIP SIP SDK contains a high performance VoIP conferencing client capable of delivering crystal clear sound even for both low and high-bandwidth users and SIP compatible devices (hardware and software). It enables a worldwide communication over the internet or intern networks either by speaking and delivers superior voice quality. It supports DTMF, adaptive silence detection, adaptive jitter buffer! VoIP SIP SDK is based on IETF standards (SIP, STUN, etc.), so it should be compatible with other standard based products such as Asterisk, OpenSER other. Read more: Forum ASP
T-SQL: Disconnect Users from a Database
Posted by
jasper22
at
10:02
|
ניתן ל"נתק" משתמשים מבסיס נתונים של SQL Server באמצעות T-SQL:USE master
goDECLARE @dbname varchar(30), @spid varchar(10), @start datetimeSELECT @start = current_timestamp, @dbname = 'DataBase Name' while(exists(Select * FROM sysprocesses WHERE dbid = db_id(@dbname)) AND
datediff(mi, @start, current_timestamp) < 5)
begin
DECLARE spids CURSOR FOR
SELECT convert(varchar, spid) FROM sysprocesses
WHERE dbid = db_id(@dbname)
OPEN spids
while(1=1)
BEGIN
FETCH spids INTO @spid
IF @@fetch_status < 0 BREAK
exec('kill ' + @spid)
END DEALLOCATE spids
endIF NOT exists(Select * FROM sysprocesses WHERE dbid = db_id(@dbname))
EXEC sp_dboption @dbname, offline, true
else
begin
PRINT 'The following processes are still using the database:'
SELECT spid, cmd, status, last_batch, open_tran, program_name, hostname
FROM sysprocesses
WHERE dbid = db_id(@dbname)Read more: Dudi Nissan's Blog
goDECLARE @dbname varchar(30), @spid varchar(10), @start datetimeSELECT @start = current_timestamp, @dbname = 'DataBase Name' while(exists(Select * FROM sysprocesses WHERE dbid = db_id(@dbname)) AND
datediff(mi, @start, current_timestamp) < 5)
begin
DECLARE spids CURSOR FOR
SELECT convert(varchar, spid) FROM sysprocesses
WHERE dbid = db_id(@dbname)
OPEN spids
while(1=1)
BEGIN
FETCH spids INTO @spid
IF @@fetch_status < 0 BREAK
exec('kill ' + @spid)
END DEALLOCATE spids
endIF NOT exists(Select * FROM sysprocesses WHERE dbid = db_id(@dbname))
EXEC sp_dboption @dbname, offline, true
else
begin
PRINT 'The following processes are still using the database:'
SELECT spid, cmd, status, last_batch, open_tran, program_name, hostname
FROM sysprocesses
WHERE dbid = db_id(@dbname)Read more: Dudi Nissan's Blog
Login failed for user 'sa' while installing SQL Server 2005
Posted by
jasper22
at
10:01
|
I was working in a SQL Server 2005 setup case which failed while starting SQL Service with error:
SQL Server Setup could not connect to the database service for server configuration. The error was: [Microsoft][SQL Native Client][SQL Server]Login failed for user 'sa'. Refer to server error logs and setup logs for more information.
Then I reviewed the SQL Server error log and noted that
DateTime Logon Error: 18456, Severity: 14, State: 10DateTime Logon Login failed for user 'sa'.As per http://support.microsoft.com/kb/925744 State 10 corresponds to PASSWORD POLICY CHECK failure. The box I was working was in a workgroup. So we went ahead and used trace flag 4606 to byepass password policy check.
How to do that? Create a reg key by name “SQLArg3” of type REG_SZ in the registry location HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Microsoft SQL Server\<Instance ID Created by the install>\MSSQLServer\Parameters and add the value “-T4606”Note: SQLArg3 should be replaced with a value higher than existing keys. So if you already have SQLArg0, SQLArg1, SQLArg2, SQLArg3 then add the value above with SQLArg4Read more: Sakthi's SQL Server Channel
SQL Server Setup could not connect to the database service for server configuration. The error was: [Microsoft][SQL Native Client][SQL Server]Login failed for user 'sa'. Refer to server error logs and setup logs for more information.
Then I reviewed the SQL Server error log and noted that
DateTime Logon Error: 18456, Severity: 14, State: 10DateTime Logon Login failed for user 'sa'.As per http://support.microsoft.com/kb/925744 State 10 corresponds to PASSWORD POLICY CHECK failure. The box I was working was in a workgroup. So we went ahead and used trace flag 4606 to byepass password policy check.
How to do that? Create a reg key by name “SQLArg3” of type REG_SZ in the registry location HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Microsoft SQL Server\<Instance ID Created by the install>\MSSQLServer\Parameters and add the value “-T4606”Note: SQLArg3 should be replaced with a value higher than existing keys. So if you already have SQLArg0, SQLArg1, SQLArg2, SQLArg3 then add the value above with SQLArg4Read more: Sakthi's SQL Server Channel
Microsoft Releases 'Fix it' Help for DLL Security Flaw
Posted by
jasper22
at
09:59
|
Microsoft updated its security advisory today concerning a dynamic link library (DLL) issue and published a "Fix it" solution to help address the problem.The issue potentially involves hundreds of applications that may fail to specify a direct path to DLL files when accessing a remote server. These poorly written applications could be subject to a hacking method called "DLL preloading attacks" or "binary planting," Microsoft explained last week. In essence, applications that reference DLL files without a specified path could pick up a planted malware files instead.
The new Fix it solution, which is buried in a Knowledge Base support article linked to the revised security advisory, is designed to simplify matters for IT pros. It's supposed to be a one-click solution to the DLL security issue. However, Microsoft added some caveats before using the Fix it solution. IT pros should first download and install update 2264107 (the workaround), which is available in a series of links below the Fix it description in the Knowledge Base article. The next step is to configure the workaround by clicking the Fix it button. Alternatively, users can manually configure the workaround through the Windows registry. Either way, this fix will "block nonsecure DLL loads from WebDAV and SMB locations," according to the article. The DLL problem is either associated with remote servers using WebDAV (or "Web-based Distributed Authoring and Versioning"), which is used with Internet Information Services component in Windows, or with remote servers using the Server Message Block (SMB) protocol. Read more: Microsoft Online
The new Fix it solution, which is buried in a Knowledge Base support article linked to the revised security advisory, is designed to simplify matters for IT pros. It's supposed to be a one-click solution to the DLL security issue. However, Microsoft added some caveats before using the Fix it solution. IT pros should first download and install update 2264107 (the workaround), which is available in a series of links below the Fix it description in the Knowledge Base article. The next step is to configure the workaround by clicking the Fix it button. Alternatively, users can manually configure the workaround through the Windows registry. Either way, this fix will "block nonsecure DLL loads from WebDAV and SMB locations," according to the article. The DLL problem is either associated with remote servers using WebDAV (or "Web-based Distributed Authoring and Versioning"), which is used with Internet Information Services component in Windows, or with remote servers using the Server Message Block (SMB) protocol. Read more: Microsoft Online
Unlimited Query string - ASP.NET
Posted by
jasper22
at
09:54
|
IntroductionQuery string is one of the primary parameter passing techniques for Web Application.Query string has some limitation in terms of query string length; this length depends on the browser. Opera supports ~4050 characters.
IE 4.0+ supports exactly 2083 characters.
Netscape 3 -> 4.78 supports up to 8192 characters.
Netscape 6 supports ~2000
[http://classicasp.aspfaq.com/forms/what-is-the-limit-on-querystring/get/url-parameters.html]
To handle the query string length limitation, there are few solutions –using POST instead of GET,
passing by Cookies
Sending query string to the server by AJAX and adding to the session in the first step and fetching the query string from the session using some GUID concepts.
Here I am going to explain a best and stable solution for all the cases.BackgroundWe have three situations when we need to pass query string to the web page.Opening a new window (http://msdn.microsoft.com/en-us/library/ms536651(VS.85).aspx)
Showing modal dialog (http://msdn.microsoft.com/en-us/library/ms536759(VS.85).aspx)
Showing the URL in iFrame (http://msdn.microsoft.com/en-us/library/ms537627(v=VS.85).aspx)
Request Maximum Size can be configured on IIS Server configuration for Server side.
http://www.iis.net/ConfigReference/system.webServer/security/requestFiltering/requestLimits
[ Thanks : daveauld - http://www.codeproject.com/Members/daveauld ]Read more: Codeproject
IE 4.0+ supports exactly 2083 characters.
Netscape 3 -> 4.78 supports up to 8192 characters.
Netscape 6 supports ~2000
[http://classicasp.aspfaq.com/forms/what-is-the-limit-on-querystring/get/url-parameters.html]
To handle the query string length limitation, there are few solutions –using POST instead of GET,
passing by Cookies
Sending query string to the server by AJAX and adding to the session in the first step and fetching the query string from the session using some GUID concepts.
Here I am going to explain a best and stable solution for all the cases.BackgroundWe have three situations when we need to pass query string to the web page.Opening a new window (http://msdn.microsoft.com/en-us/library/ms536651(VS.85).aspx)
Showing modal dialog (http://msdn.microsoft.com/en-us/library/ms536759(VS.85).aspx)
Showing the URL in iFrame (http://msdn.microsoft.com/en-us/library/ms537627(v=VS.85).aspx)
Request Maximum Size can be configured on IIS Server configuration for Server side.
http://www.iis.net/ConfigReference/system.webServer/security/requestFiltering/requestLimits
[ Thanks : daveauld - http://www.codeproject.com/Members/daveauld ]Read more: Codeproject
Microsoft(R) Silverlight™ 4 SDK
Posted by
jasper22
at
09:52
|
The Microsoft® Silverlight™ 4 SDK contains online documentation, online samples, libraries and tools for developing Silverlight 4 applications.
Usage of the SDK is subject to the SDK License (included in the package). Read more: MS Download
Usage of the SDK is subject to the SDK License (included in the package). Read more: MS Download
Quick Time WPF Lib
Posted by
jasper22
at
09:52
|
Library for use Quick Time in WPF ApplicationsWhen I'm created project in .NET 4.0 and tried to reference "Apple QuickTime Control 2.0" I'v got an error. But against 3.5 SP1 Runtime it is added normal. But 3.5 SP1 doesn't support embedded interop types. I've created this project to help people, if they want to use QuickTime in WPF Applications Read more: Codeplex
Реверсинг .Net приложений. Часть 0
Posted by
jasper22
at
09:50
|
Перед вами первая статья из цикла «Реверсинг .Net приложений», в которой мы не будем работать с MSIL, не будем снимать пакеры и протекторы, и не встретимся с обфусцированным кодом. Всем этим мы займемся в будущих статьях, а пока мы пощупаем несколько основных инструментов и по традиции решим несложный крэкми.
Платформа .Net в текущем состоянии крайне уязвима:1. Программы, написанные для .Net, компилируются не в native, а в байт-код платформы .Net, названный MSIL (MicroSoft Intermediate Language). IL код компилируется непосредственно перед запуском. Такая технология получила название Just-in-time compilation (JIT, компиляция «на лету»). 2. Все программы содержат метаданные.
«Метаданные (metadata) — это данные, описывающие другие данные. В нашем контексте — это набор программных элементов ЕХЕ-файла, таких как типы и реализации методов.»Именно благодаря метаданным, а точнее их особым свойствам в среде .Net мы можем с легкостью получить исходники программ. 2.1 Метаданные в .Net обязательны и универсальны.
«Каждая программа в среде .Net, помимо кода на языке MSIL обязательно содержит метаданные, описывающие как её в целом (манифест), так и каждый тип, содержащийся в ней.» 2.2 Метаданные в .Net общедоступны.
«Доступ к метаданным могут получить любые программные компоненты и любые инструменты программирования.»2.3 Метаданные в .Net исчерпывающи.
«Метаданные содержат подробнейшую информацию о каждом типе: его имя, типы и имена его полей, описание свойств и методов со всеми их параметрами и возвращаемыми значениями. Здесь же хранится информация о доступности (видимости) всех членов класса и об их атрибутах. Метаданные хранят не только информацию о интерфейсе экспортируемых классов. Такие детали реализации, как структура защищённых полей, описания защищённых методов и других компонентов, также могут быть извлечены из метаданных.» Подробнее о структуре метаданных в .Net можно почитать здесь, а мы тем временем приступим непосредственно к практике. Для работы нам понадобитсяRead more: Habrahabr.ru
Платформа .Net в текущем состоянии крайне уязвима:1. Программы, написанные для .Net, компилируются не в native, а в байт-код платформы .Net, названный MSIL (MicroSoft Intermediate Language). IL код компилируется непосредственно перед запуском. Такая технология получила название Just-in-time compilation (JIT, компиляция «на лету»). 2. Все программы содержат метаданные.
«Метаданные (metadata) — это данные, описывающие другие данные. В нашем контексте — это набор программных элементов ЕХЕ-файла, таких как типы и реализации методов.»Именно благодаря метаданным, а точнее их особым свойствам в среде .Net мы можем с легкостью получить исходники программ. 2.1 Метаданные в .Net обязательны и универсальны.
«Каждая программа в среде .Net, помимо кода на языке MSIL обязательно содержит метаданные, описывающие как её в целом (манифест), так и каждый тип, содержащийся в ней.» 2.2 Метаданные в .Net общедоступны.
«Доступ к метаданным могут получить любые программные компоненты и любые инструменты программирования.»2.3 Метаданные в .Net исчерпывающи.
«Метаданные содержат подробнейшую информацию о каждом типе: его имя, типы и имена его полей, описание свойств и методов со всеми их параметрами и возвращаемыми значениями. Здесь же хранится информация о доступности (видимости) всех членов класса и об их атрибутах. Метаданные хранят не только информацию о интерфейсе экспортируемых классов. Такие детали реализации, как структура защищённых полей, описания защищённых методов и других компонентов, также могут быть извлечены из метаданных.» Подробнее о структуре метаданных в .Net можно почитать здесь, а мы тем временем приступим непосредственно к практике. Для работы нам понадобитсяRead more: Habrahabr.ru
Making WCF RIA Services work in a DMZ/Multitier architecture using Application Request Routing
Posted by
jasper22
at
09:49
|
IntroductionIn large companies / governments / ... most of the time the application architecture needs to follow a set of rules (focused on maintainability and security).
These could be rules like the following: The applications need to be developed following a Multitier architecture
Each tier should be physically separated for security.
The business logic tier is the only one that can connect to the data tier.
The presentation tier only connects to the business logic tier.
The presentation tier may not directly connect to the data tier.
The presentation tier is located in a DMZ, other tiers are heavily secured.
...
Each project / company will have its own rules but the concept stays the same.
Here is an example of how this could be achieved in ASP.NET:
A. Preparing the server(s)Make sure you have IIS 7 or IIS 7.5
Download the Application Request Routing extension: x86 / x64
Install the extension (it might install other extensions first)
For testing purposes we'll simulate 2 servers on one machine.
To do this, open c:\windows\system32\drivers\etc\hosts with notepad.127.0.0.1 presentationtier
127.0.0.1 logictierWe'll link these hostnames using host headers in IIS.If you have 2 servers you can use for testing, please do (and you can skip this step).
But don't forget to install the WCF RIA Services Toolkit!
Finally we'll configure the Application Request Routing.Read more: Codeproject
These could be rules like the following: The applications need to be developed following a Multitier architecture
Each tier should be physically separated for security.
The business logic tier is the only one that can connect to the data tier.
The presentation tier only connects to the business logic tier.
The presentation tier may not directly connect to the data tier.
The presentation tier is located in a DMZ, other tiers are heavily secured.
...
Each project / company will have its own rules but the concept stays the same.
Here is an example of how this could be achieved in ASP.NET:
A. Preparing the server(s)Make sure you have IIS 7 or IIS 7.5
Download the Application Request Routing extension: x86 / x64
Install the extension (it might install other extensions first)
For testing purposes we'll simulate 2 servers on one machine.
To do this, open c:\windows\system32\drivers\etc\hosts with notepad.127.0.0.1 presentationtier
127.0.0.1 logictierWe'll link these hostnames using host headers in IIS.If you have 2 servers you can use for testing, please do (and you can skip this step).
But don't forget to install the WCF RIA Services Toolkit!
Finally we'll configure the Application Request Routing.Read more: Codeproject
Application Extension Services
Application extension services enable you to extend the Silverlight application model. You will typically use extension services to encapsulate functionality used by multiple applications in a particular feature domain. For example, you can use extension services to implement specialized media handling or a custom data access layer. The Application class provides services common to most applications. For more information, see Application Services. You can provide additional services by creating a custom Application subclass, but this could lead to unnecessary complexity. For example, creating a subclass would require that applications derive from your subclass, which prevents the use of multiple extension frameworks at the same time. Instead of creating a custom Application subclass, you can add application extension services through the Application.ApplicationLifetimeObjects property. This enables you to combine multiple services and implement complex service dependencies. Application extension services must implement the IApplicationService interface. This interface provides support for service initialization and cleanup. Services can also implement the IApplicationLifetimeAware interface if they require deeper integration with application lifetime events. This topic contains the following sections:
Read more: MSDN
- Registering Extension Services
- Accessing Extension Services from Application Code
- Implementing the IApplicationService Interface
- Implementing the IApplicationLifetimeAware Interface
Read more: MSDN
SQL Server - Understanding the behavior of @@TRANCOUNT when querying from a client application
Posted by
jasper22
at
10:06
|
As you know, @@TRANCOUNT is used to inform you about the number of live transactions active at point. If you want to retrieve it's value using an application, you should be careful about the behaviour of the returned value. Let us consider the procedurecreate procedure get_count
as
select @@TRANCOUNT as trancountConsider the VB code that calls the procedurePrivate Sub Form_Load()
as
begin transaction
select @@TRANCOUNT
rollback transaction Read more: Beyond Relational
as
select @@TRANCOUNT as trancountConsider the VB code that calls the procedurePrivate Sub Form_Load()
Dim con As New ADODB.ConnectionEnd SubThe result is 0 eventhough there is transaction activated by application Alter the procedure like belowalter procedure get_count
Dim rs As New ADODB.Recordset
con.Open "connection string"
con.BeginTrans
Set rs = con.Execute("exec get_count")
MsgBox rs("trancount")
con.CommitTrans
as
begin transaction
select @@TRANCOUNT
rollback transaction Read more: Beyond Relational
FormsAuthentication and ClientHttpStack with mixed applications
Posted by
jasper22
at
10:03
|
if you ever tried to have mixed applications, with some regular ASP.NET pages and a bunch of Silverlight applications, where the forms authentication is made with a traditional ASP.NET login page, you have for sure found that you are forced to use the BrowserHttp stack into the Silverlight applications because the FormsAuthentication cookie is not shared between the ASP.NET page and the new ClientHttp stack that is available since Silverlight 3.0.
Since the new ClientHttp stack for many reasons is a huge improvement, is would be a beautiful thing being able to use this stack every time there is the need to place HTTP calls. Finally I found a way to share this cookie across the two stacks, but I want to warn you that the sole way to accomplish this task require a little "dirty hack", that someone might consider unacceptable while it opens some minor security concerns I will detail at the end of the post.
Before continue reading this post please have in mind that my suggestion is to always use the BrowserHttp stack in these scenario, until you do not really need the new stack, e.g. if you have to use some special verb or for some other particular reason. The dirty hack explained The first thing you have to know is that when you are using the ClientHttp stack you are free to access the cookies issued by the server and it is also possible to add some cookies to attach to a call. The task we would have to accomplish is simply to read a cookie (often called .ASPXAUTH) from the BrowserHttp stack and add it to the ClientHttp stack and it may seems and easy task.
Unfortunately the BrowserHttp Stack prevent us from accessing the cookies. The cookies management is one of the features that has been added by the new ClientHttp stack and there is not any way to pump out them from the old one.
So my first try was to use the HtmlPage.Document.Cookies property, as I found in some examples on the Internet. Perhaps this may work when the authentication cookie is issued by a custom authentication system, but with FormsAuthentication it is another failed try because the cookies issued by the ASP.NET engine are HttpOnly. This attribute, added since IE6 SP1, prevents the browser scripts - and Silverlight is considered a client script - from accessing these cookies that remain hidden.
So, how can we share these cookies? The only thing you can do is to add the cookie to the InitParams collection when you initialize the plugin in the html page. You have to change this page to be an aspx page and then you can create two keys into the InitParams collection like the following: public string GetFormsAuthenticationCookie()
{
Since the new ClientHttp stack for many reasons is a huge improvement, is would be a beautiful thing being able to use this stack every time there is the need to place HTTP calls. Finally I found a way to share this cookie across the two stacks, but I want to warn you that the sole way to accomplish this task require a little "dirty hack", that someone might consider unacceptable while it opens some minor security concerns I will detail at the end of the post.
Before continue reading this post please have in mind that my suggestion is to always use the BrowserHttp stack in these scenario, until you do not really need the new stack, e.g. if you have to use some special verb or for some other particular reason. The dirty hack explained The first thing you have to know is that when you are using the ClientHttp stack you are free to access the cookies issued by the server and it is also possible to add some cookies to attach to a call. The task we would have to accomplish is simply to read a cookie (often called .ASPXAUTH) from the BrowserHttp stack and add it to the ClientHttp stack and it may seems and easy task.
Unfortunately the BrowserHttp Stack prevent us from accessing the cookies. The cookies management is one of the features that has been added by the new ClientHttp stack and there is not any way to pump out them from the old one.
So my first try was to use the HtmlPage.Document.Cookies property, as I found in some examples on the Internet. Perhaps this may work when the authentication cookie is issued by a custom authentication system, but with FormsAuthentication it is another failed try because the cookies issued by the ASP.NET engine are HttpOnly. This attribute, added since IE6 SP1, prevents the browser scripts - and Silverlight is considered a client script - from accessing these cookies that remain hidden.
So, how can we share these cookies? The only thing you can do is to add the cookie to the InitParams collection when you initialize the plugin in the html page. You have to change this page to be an aspx page and then you can create two keys into the InitParams collection like the following: public string GetFormsAuthenticationCookie()
{
return string.Format(
}Read more: Silverlight Playground"FormsCookieName={0},FormsCookieValue={1}",FormsAuthentication.FormsCookieName,this.Request.Cookies[FormsAuthentication.FormsCookieName].Value);
Shutdown reason codes are reason codes, not error codes or HRESULTs
Posted by
jasper22
at
10:01
|
A customer liaison asked the following question on behalf of his customer:My customer is finding that their Windows Server 2003 system has restarted, and they want to find out why. I've found some event log similar to the ones below, but I don't know what error code 0x84020004 is. I've searched the Knowledge Base but couldn't find anything relevant. Please let me know why the system restarted. Event Type: Information
Event Source: USER32
Event Category: None
Event ID: 1074
Date: 3/20/2009
Time: 11:51:30 AM
User: GROUP1\infraadmin
Computer: DATA2
Description:
The process Explorer.EXE has initiated the shutdown of computer SYSP1 on behalf
of user GROUP1\infraadmin for the following reason: Operating System:
Reconfiguration (Planned)
Reason Code: 0x84020004
Shutdown Type: restart
Comment:
The value 0x84020004 is not an error code. It says right there that it's a reason code: Reason Code: 0x84020004
The system shutdown reason codes are documented in MSDN under the devious heading System Shutdown Reason Codes. In this case, the value 0x84020004 is a combination ofSHTDN_REASON_FLAG_PLANNED 0x80000000
SHTDN_REASON_FLAG_CLEAN_UI 0x04000000 // reason.h
SHTDN_REASON_MAJOR_OPERATINGSYSTEM 0x00020000
SHTDN_REASON_MINOR_RECONFIG 0x00000004That value for SHTDN_REASON_FLAG_CLEAN_UI is missing from the MSDN documentation for some reason, but's listed in reason.h. The flag means that the system was shut down in a controlled manner, as opposed to SHDTN_REASON_FLAG_DIRTY_UI which means that the system lost power and did not go through a clean shutdown. Read more: The old new thing
Event Source: USER32
Event Category: None
Event ID: 1074
Date: 3/20/2009
Time: 11:51:30 AM
User: GROUP1\infraadmin
Computer: DATA2
Description:
The process Explorer.EXE has initiated the shutdown of computer SYSP1 on behalf
of user GROUP1\infraadmin for the following reason: Operating System:
Reconfiguration (Planned)
Reason Code: 0x84020004
Shutdown Type: restart
Comment:
The value 0x84020004 is not an error code. It says right there that it's a reason code: Reason Code: 0x84020004
The system shutdown reason codes are documented in MSDN under the devious heading System Shutdown Reason Codes. In this case, the value 0x84020004 is a combination ofSHTDN_REASON_FLAG_PLANNED 0x80000000
SHTDN_REASON_FLAG_CLEAN_UI 0x04000000 // reason.h
SHTDN_REASON_MAJOR_OPERATINGSYSTEM 0x00020000
SHTDN_REASON_MINOR_RECONFIG 0x00000004That value for SHTDN_REASON_FLAG_CLEAN_UI is missing from the MSDN documentation for some reason, but's listed in reason.h. The flag means that the system was shut down in a controlled manner, as opposed to SHDTN_REASON_FLAG_DIRTY_UI which means that the system lost power and did not go through a clean shutdown. Read more: The old new thing
Create RESTful WCF Service API : Step By Step Guide
Posted by
jasper22
at
10:01
|
IntroductionWindows Communication Foundation (WCF) is an SDK for developing and deploying services on Windows. WCF provides a runtime environment for your services, enabling you to expose CLR types as services, and to consume other services as CLR types. In this article I am going to explain you how to implement restful service API using WCF 4.0 . The Created API returns XML and JSON data using WCF attributes. What is REST? Based on Roy Fielding theory "Representational State Transfer (REST), attempts to codify the architectural style and design constraints that make the Web what it is. REST emphasizes things like separation of concerns and layers, statelessness, and caching, which are common in many distributed architectures because of the benefits they provide. These benefits include interoperability, independent evolution, interception, improved scalability, efficiency, and overall performance." Actually only the difference is how clients access our service. Normally, a WCF service will use SOAP, but if you build a REST service clients will be accessing your service with a different architectural style (calls, serialization like JSON, etc). REST uses some common HTTP methods to insert/delete/update/retrive infromation which is below: a) GET - Requests a specific representation of a resourceb) PUT - Create or update a resource with the supplied representation c) DELETE - Deletes the specified resourced) POST - Submits data to be processed by the identified resource Why and where to use REST? Few days back i was writing a service which was suppose to access by heterogeneous language/platform/system. It can be used by iPhone, Android, Windows Mobile, .NET web application, JAVA or PHP. Using web service it was bit complex for me to expose it to everyone using uniform system. Then we decided to use REST, which was easily espoused over cloud. This was a great example which shows the capability of SIMPLE RESTful SERVICE :) . Below is some point which will help you to understand why to use the RESTful services. Read more: Codeproject
Microsoft Easy Assist
Posted by
jasper22
at
10:00
|
Did you know about Microsoft Easy Assist?“You can use Microsoft Easy Assist to allow a Microsoft support professional to remotely connect to your computer and help you solve a problem. Using a secure connection, the support professional can view your desktop and perform diagnostics and troubleshooting steps. Before using Easy Assist, you'll need to speak to a support professional and obtain a session ID. For contact information, go to the Microsoft Support website. Once you have a session ID, go to the Easy Assist webpage” Read more: I'm PC
FUSE(file system in userspace) for MS-SQL using C#
Posted by
jasper22
at
10:00
|
IntroductionEvery time I use Linux, I feel a piece of jealousy to see its filesystems. Not only NTFS, FAT32, but huge palette of data storages included GDocs etc. Now I can raise head up! Dokan rocks! What is Dokan?What Dokan is? Simply device driver encapsulated filesystem calls. By using proxy functions gives programatically create response for ReadFile, WriteFile, FindFiles and other core I/O operations. Even on .NET! There are also Dokan.NET interface for using C#.
Now you are enable to write your own filesystems. You can create your own filesystem or you can be inspired by another applications using Dokan . What about filesystem using memory as ramdisk, system used encrypted file as data storage? Mirroring of some disc or directories, access to registry via filesystem, list of processes as files on attached disk? Open your mind and try to find another use for Dokan. This article will help you. You will be able to store your files on MS SQL database, copy, rename, update, delete etc. Simply file versioning is also included. This was reason why I started play with Dokan. Create external storage with some versioning, mounted as disk, easy to use for end users. To create version of file, just add extension ".version" to the end of filename and copy to disk. File will be renamed to previous extension and previous version of file receive version number. You can choose if you want to see all versions or only actual version. PrerequisitiesInstalled Dokan.
Download actual version (0.53) from Dokan main pages and install. (code was tested with version 0.52)Prepared MS-SQL
For testing purposes is suitable MS-SQL Express edition on your PC, but I used SQL server located on virtual machine to simulate some network traffic and network accidents. For production be carefull and create special user account and allow to run stored procedures for this account. Do not miss this step. It is good security strategy. Read more: Codeproject
Now you are enable to write your own filesystems. You can create your own filesystem or you can be inspired by another applications using Dokan . What about filesystem using memory as ramdisk, system used encrypted file as data storage? Mirroring of some disc or directories, access to registry via filesystem, list of processes as files on attached disk? Open your mind and try to find another use for Dokan. This article will help you. You will be able to store your files on MS SQL database, copy, rename, update, delete etc. Simply file versioning is also included. This was reason why I started play with Dokan. Create external storage with some versioning, mounted as disk, easy to use for end users. To create version of file, just add extension ".version" to the end of filename and copy to disk. File will be renamed to previous extension and previous version of file receive version number. You can choose if you want to see all versions or only actual version. PrerequisitiesInstalled Dokan.
Download actual version (0.53) from Dokan main pages and install. (code was tested with version 0.52)Prepared MS-SQL
For testing purposes is suitable MS-SQL Express edition on your PC, but I used SQL server located on virtual machine to simulate some network traffic and network accidents. For production be carefull and create special user account and allow to run stored procedures for this account. Do not miss this step. It is good security strategy. Read more: Codeproject
Why GUIDs aren't so Good as SQL Server Primary Key
Posted by
jasper22
at
09:59
|
My informal poll of fellow developers reveals that by about a 4 to 1 margin, we are all using UNIQUEIDENTIFIER primary keys. Very Bad Idea.The GUID (UNIQUEIDENTIFIER) datatype is a wide column (16 bytes) and contains a unique combination of 33 uppercase and numeric characters. As the primary key it will be be stored in a clustered index (unless specified otherwise). it will be the page pointer for each leaf page in any non-clustered index. About the only thing you can do to improve the performance of this is to increase the Fill Factor to 100%. When a GUID is used instead of an integer identity column then the 33 characters need to be matched for each row that is returned using that column in the where clause. If a high volume of inserts are done on these tables then the GUIDs, being large, will contribute to page splits, as will the fact that NEWID() generates a random value, which could place a new record on any of the data pages. The result? Performance problems. A good clustering key should be sequential. But, a GUID that is not sequential - like one that has it's value generated in the client or generated by the newid() function in SQL Server can be a very bad choice - mostly because of the fragmentation that it creates in the base table but also because of its size. It's unnecessarily wide -- 4 times wider than an int-based identity - which can give you 2 billion unique rows. If you need more than 2 billion you can always go with a bigint (8-byte int) and get 2^63-1 rows. You could use the new NEWSEQUENTIALID() function to improve things, but you're still dealing with 16 bytes of glop. Here are some estimates from Kimberly Tripp on database size:
Base Table with 1,000,000 rows (3.8MB for int vs. 15.26MB with UNIQUEIDENTIFIER)
6 nonclustered indexes (22.89MB for int vs. 91.55MB with UNIQUEIDENTIFIER) Read more: PETER BROMBERG UnBlog
Base Table with 1,000,000 rows (3.8MB for int vs. 15.26MB with UNIQUEIDENTIFIER)
6 nonclustered indexes (22.89MB for int vs. 91.55MB with UNIQUEIDENTIFIER) Read more: PETER BROMBERG UnBlog
How to Drag Out Files Like Gmail
Posted by
jasper22
at
09:58
|
Ryan Seddon, aka the CSS Ninja, has a nice blog post up where he reverse engineers the new feature in Gmail where you can drag attachments from an email on to your desktop. Note that the feature only currently works in Chrome. Ryan begins with the following code:JAVASCRIPT:
Describing the code Ryan says: From the code above you attach an ondragstart event listener to something you want to “drag out” and save to your file system. On the dragstart event you set the data using the new “DownloadURL” type and pass file information to it. Note though that in order to pass the correct data to the dragstart event you need to provide a download URL that can be passed to the setData("DownloadURL" call. Read more: ajaxian
var file = document.getElementById( "dragout" );
file.addEventListener( "dragstart", function( evt ) {
evt.dataTransfer.setData( "DownloadURL", fileDetails );
}, false);
Describing the code Ryan says: From the code above you attach an ondragstart event listener to something you want to “drag out” and save to your file system. On the dragstart event you set the data using the new “DownloadURL” type and pass file information to it. Note though that in order to pass the correct data to the dragstart event you need to provide a download URL that can be passed to the setData("DownloadURL" call. Read more: ajaxian
Microsoft Office Protocol Documentation
Posted by
jasper22
at
09:55
|
The Office protocol documentation provides detailed technical specifications for Microsoft proprietary protocols (including extensions to industry-standard or other published protocols) that are implemented and used in Microsoft Office client programs to interoperate or communicate with Microsoft products. The documentation includes a set of companion overview and reference documents that supplement the technical specifications with conceptual background, overviews of inter-protocol relationships and interactions, and technical reference information. Read more: MS Download
Burning Man Goes Open Source For Cell Phones
Today I bring you a story that has it all: a solar-powered, low-cost, open source cellular network that's revolutionizing coverage in underprivileged and off-grid spots. It uses VoIP yet works with existing cell phones. It has pedigreed founders. Best of all, it is part of the sex, drugs and art collectively known as Burning Man. ... The technology starts with the 'they-said-it-couldn't-be-done' open source software, OpenBTS. OpenBTS is built on Linux and distributed via the AGPLv3 license. When used with a software-defined radio such as the Universal Software Radio Peripheral (USRP), it presents a GSM air interface ("Um") to any standard GSM cell phone, with no modification whatsoever required of the phone. It uses open source Asterisk VoIP software as the PBX to connect calls, though it can be used with other soft switches, too. ... This is the third year its founders have decided to trial-by-fire the system by offering free cell phone service to the 50,000-ish attendees at Burning Man, which begins today in Black Rock City, Nevada Read more: Slashdot
VLC 1.1.4 is released! PPA Ubuntu
Posted by
jasper22
at
10:20
|
VLC 1.1.4 is released, this is the fourth bugfix release of the VLC 1.1.x branch. This release comes especially to fix important security issue that was discovered in most windows application, Linux in general is not affected by this security bug. So this new release is targeted for the windows platform. What`s new in VLC 1.1.4?
Read more: Unixmen
Avformat fps displaying fix-TS,
Fix an issue where some programs would get dropped (fixes DVB issues too)
-Audio filters: · fix timestamps handling on some filters that provoked issues when playback of mono streams, especially on Windows
-Interface and extensions: · Youtube and other scripts updates
Read more: Unixmen
Apertus, the Open Source HD Movie Camera
Posted by
jasper22
at
10:18
|
This article takes a tour of the hardware and software behind the innovative Apertus, a real world open source project. Led by Oscar Spierenburg and a team of international developers, the project aims to produce 'an affordable community driven free software and open hardware cinematic HD camera for a professional production environment' Read more: Slashdot
Silverlight Media Framework 2.1
Posted by
jasper22
at
10:16
|
Release Notes
This is the official Release To Web (RTW) of the Microsoft Silverlight Media Framework 2.1. Notes:
To use SMF with IIS Smooth Streaming you must download the IIS Smooth Streaming Client here: http://www.iis.net/download/SmoothClient
The .CHM file in the API DOCUMENTATION download may need to be unblocked. Just right click on the file, select "Properties", and choose "Unblock".Fixes/Updates:
Read more: Codeplex
This is the official Release To Web (RTW) of the Microsoft Silverlight Media Framework 2.1. Notes:
To use SMF with IIS Smooth Streaming you must download the IIS Smooth Streaming Client here: http://www.iis.net/download/SmoothClient
The .CHM file in the API DOCUMENTATION download may need to be unblocked. Just right click on the file, select "Properties", and choose "Unblock".Fixes/Updates:
Fix JavaScript error message (missing Silverlight.js) in SmoothStreamingPlayer.html & ProgressiveDownloadPlayer.html
http://smf.codeplex.com/Thread/View.aspx?ThreadId=218806 SMF Bitrate graph does not reload:
http://smf.codeplex.com/Thread/View.aspx?ThreadId=217902Added support for PlaylistItem.StreamSource
http://smf.codeplex.com/workitem/17760PlaySpeedState.Normal renamed to PlaySpeedState.NormalPlayback
http://smf.codeplex.com/workitem/18384 SlowMotionElement wrong behavior
http://smf.codeplex.com/workitem/18245Added PlaylistItem.StreamSource
http://smf.codeplex.com/workitem/17760 Calling Play() before OnApplyTemplate() causes Player to stop working
http://smf.codeplex.com/workitem/18194(more..)
Read more: Codeplex
Analyzing Silverlight Memory Usage: Part 1 – Obtaining Measurements
Posted by
jasper22
at
10:15
|
A View from the Top
So you’ve decided that it’s time to optimize the memory footprint of your Silverlight application. Before you’ll be able to do this analysis and optimization, you’ll need to understand a little bit about the way that Silverlight is structured.
Though the Silverlight programming model that you’ve come to know and love is accessed via managed code, much of Silverlight’s internals are written in native code (C++). The actions that you take in managed code can cause Silverlight to allocate large amounts of native memory on your behalf, be it for layout, rendering, image allocations, you name it.
Especially important to remember is that your managed Silverlight elements will have a native memory counterpart. Reducing the element count in your visual tree will have a direct and favorable impact in native working set. Because of these native allocations, this article will go over analyzing native memory in addition to managed memory.
Where to start?
One of the most intimidating parts of performing memory analysis can be deciding where to start. Often developers don’t focus on memory usage until it becomes a problem, at which point the project has already grown to a rather complex scale. The good news is that there is already a great free tool that can help you divide the problem into more manageable pieces, this tool is VMMap. Read more: Silverlight Performance Blog
So you’ve decided that it’s time to optimize the memory footprint of your Silverlight application. Before you’ll be able to do this analysis and optimization, you’ll need to understand a little bit about the way that Silverlight is structured.
Though the Silverlight programming model that you’ve come to know and love is accessed via managed code, much of Silverlight’s internals are written in native code (C++). The actions that you take in managed code can cause Silverlight to allocate large amounts of native memory on your behalf, be it for layout, rendering, image allocations, you name it.
Especially important to remember is that your managed Silverlight elements will have a native memory counterpart. Reducing the element count in your visual tree will have a direct and favorable impact in native working set. Because of these native allocations, this article will go over analyzing native memory in addition to managed memory.
Where to start?
One of the most intimidating parts of performing memory analysis can be deciding where to start. Often developers don’t focus on memory usage until it becomes a problem, at which point the project has already grown to a rather complex scale. The good news is that there is already a great free tool that can help you divide the problem into more manageable pieces, this tool is VMMap. Read more: Silverlight Performance Blog
Gmail Priority Inbox Sorts Your Email For You. And It’s Fantastic.
Posted by
jasper22
at
10:14
|

Email overload has finally met its match. Tomorrow, Gmail is rolling out a new feature called Priority Inbox that is going to be a Godsend for those of you who dread opening your email. In short, Google has built a system that figures out which of your messages are important, and presents them at the top of the screen so you don’t miss them. The rest of your messages are still there, but you don’t have to dig through dozens of newsletters and confirmations to find the diamonds in rough. The beauty of the system lies in its simplicity — it’s nearly as easy as Gmail’s one click spam filter. There’s almost no setup: once it’s activated on your account, you’ll see a prompt asking you if you want to enable Priority Inbox. You can choose from a few options (the order of your various inboxes and if there are any contacts you’d like to always mark ‘Important’) but don’t have to setup any rules or ‘teach’ Gmail what you want it to mark important. It just works, at least most of the time. Read more: Techcrunch
Send and Read SMS through a GSM Modem using AT Commands
Posted by
jasper22
at
10:13
|
IntroductionThere are many different kinds of applications SMS applications in the market today, and many others are being developed. Applications in which SMS messaging can be utilized are virtually unlimited. Some common examples of these are given below: Person-to-person text messaging is the most commonly used SMS application, and it is what the SMS technology was originally designed for.
Many content providers make use of SMS text messages to send information such as news, weather report, and financial data to their subscribers.
SMS messages can carry binary data, and so SMS can be used as the transport medium of wireless downloads. Objects such as ringtones, wallpapers, pictures, and operator logos can be encoded in SMS messages.
SMS is a very suitable technology for delivering alerts and notifications of important events.
SMS messaging can be used as a marketing tool.
In general, there are two ways to send SMS messages from a computer / PC to a mobile phone:Connect a mobile phone or GSM/GPRS modem to a computer / PC. Then use the computer / PC and AT commands to instruct the mobile phone or GSM/GPRS modem to send SMS messages.
Connect the computer / PC to the SMS center (SMSC) or SMS gateway of a wireless carrier or SMS service provider. Then send SMS messages using a protocol / interface supported by the SMSC or SMS gateway.
In this article, I will explain the first way to send, read, and delete SMS using AT commands. But before starting, I would like to explain a little bit about AT commands. Read more: Codeproject
Many content providers make use of SMS text messages to send information such as news, weather report, and financial data to their subscribers.
SMS messages can carry binary data, and so SMS can be used as the transport medium of wireless downloads. Objects such as ringtones, wallpapers, pictures, and operator logos can be encoded in SMS messages.
SMS is a very suitable technology for delivering alerts and notifications of important events.
SMS messaging can be used as a marketing tool.
In general, there are two ways to send SMS messages from a computer / PC to a mobile phone:Connect a mobile phone or GSM/GPRS modem to a computer / PC. Then use the computer / PC and AT commands to instruct the mobile phone or GSM/GPRS modem to send SMS messages.
Connect the computer / PC to the SMS center (SMSC) or SMS gateway of a wireless carrier or SMS service provider. Then send SMS messages using a protocol / interface supported by the SMSC or SMS gateway.
In this article, I will explain the first way to send, read, and delete SMS using AT commands. But before starting, I would like to explain a little bit about AT commands. Read more: Codeproject
BITS Logging
Posted by
jasper22
at
10:12
|
The attached ZIP file contains scripts to both enable and disable logging for the Background Intelligent Transfer Service, or BITS. Both scripts must be run elevated so on Windows Vista or newer open an elevated command prompt and run these scripts. You may also right-click on the scripts and choose to Run as administrator. Read more: Heath Stewart's Blog
Silverlight CRUD Operations Using WCF Service
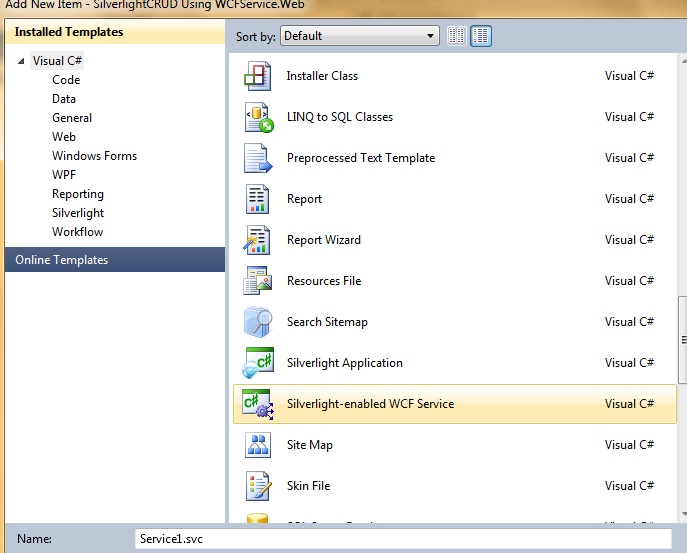
You will see project layout like this. Web project works like server project all database related classes and services, .aspx test pages, configuration file and other project has all .xaml pages controls. Now add a new Silverlight enabled wcf service.In this sample I am using NORTHWND database which is available in App_Data folder. First add a new class in web project.Customers.csusing System;
usingSystem.Collections.Generic;
usingSystem.Linq;
usingSystem.Web;namespace SilverlightCRUD_Using_WCFService.Web
{
public class Customers
{
public string CustomerID { get; set; }public string CompanyName { get; set; }public string ContactName { get; set; }public string ContactTitle { get; set; }
}}
Now add functions in service class. This is connection string.string conn = "Data Source=.\\SQLEXPRESS;AttachDbFilename=|DataDirectory|\\NORTHWND.MDF;Integrated Security=True;User Instance=True"; Read more: C# Corner
New SIlverilght Video Tutorial: How to Create a ListBox with a Static Background
Posted by
jasper22
at
18:02
|
Someone from my Silverlight Email List emailed me and told me that he had been struggling trying to create a ListBox with a static background image. So, I made this short, 3 mintue tutorial I show you how to do it all in Blend. So, if you need a ListBox with a background image then watch the video located here: http://tinyurl.com/2g84vd8\Download the Source HereEnjoy! VictorRead more: Victor Gaudioso's Silverlight Blog
Google Realtime Search Gets New Name, Its Own URL, And Kick In The Pants
Posted by
jasper22
at
17:58
|

In a move that emphasizes the increasing importance of realtime search, Google has just given their realtime search function a kick in the pants, moving it from the lowly “Updates” sidebar on regular Google search to it’s own URL http://google.com/realtime, which was broken this morning but now seems to be redirecting to http://www.google.com/realtime?esrch=RealtimeLaunch::Experiment. In addition, Google has added some supplementary tools to help you sift through news stories, blog posts, Twitter, Facebook, and Buzz updates including …Read more: Techcrunch
WPF DataGrid Control - Performing Update and Delete Operations
Posted by
jasper22
at
17:53
|
I was recently working on a requirement to perform Update and Delete operations on a WPF DataGrid control (similar to what developers do in a ASP.NET Grid control). The WPF DataGrid is an excellent control to bind with collections and provides various events to work with.
In this article, I have developed a small application using ADO.NET EF (Entity Framework) and WPF DataGrid. The script for creating the Database table is in the db.sql file in the source code of this article.
Note: If you are new to creating applications using WPF and ADO.NET EF then read my previous article Creating Applications using Windows Presentation Foundation (WPF) Commanding and ADO.NET Entity Framework Creating ADO.NET EF and WPF Project Step 1: Open VS2010 and create a WPF project and name it as ‘WPF_DataGridEvents’.
Step 2: To this project, add a new ADO.NET Entity Model. Name it as ‘CompanyEDMX’. From the wizard for ADO.NET EF, select ‘Employee’ table created in SQL Server using the db.sql script. After the completion of the wizard, the following model will be generated: Read more: dot net curry.com
In this article, I have developed a small application using ADO.NET EF (Entity Framework) and WPF DataGrid. The script for creating the Database table is in the db.sql file in the source code of this article.
Note: If you are new to creating applications using WPF and ADO.NET EF then read my previous article Creating Applications using Windows Presentation Foundation (WPF) Commanding and ADO.NET Entity Framework Creating ADO.NET EF and WPF Project Step 1: Open VS2010 and create a WPF project and name it as ‘WPF_DataGridEvents’.
Step 2: To this project, add a new ADO.NET Entity Model. Name it as ‘CompanyEDMX’. From the wizard for ADO.NET EF, select ‘Employee’ table created in SQL Server using the db.sql script. After the completion of the wizard, the following model will be generated: Read more: dot net curry.com
Making Ubuntu look like Windows 7
Posted by
jasper22
at
17:53
|

LG putting 9.7-inch color, 19-inch flexible e-paper displays into production
Posted by
jasper22
at
17:51
|

Создание изображений из элементов управления WPF
Posted by
jasper22
at
17:37
|
Существует достаточно распространенная задача, когда окно приложения или его часть необходимо сохранить как изображение. В WPF есть класс, который очень упрощает ее решение – RenderTargetBitmap. Он позволяет получить изображение любого элемента управления WPF (включая его дочерние элементы) в растровом формате. Рассмотрим пример использования данного класса и некоторые особенности. Сразу перейдем к коду примера. После я дам краткое его описание и расскажу про пару особенностей./// <summary>Renders the Visual object and store it to file.</summary>
/// <param name="baseElement">The Visual object to be used as a bitmap. </param>
/// <param name="imageWidth">The height of the bitmap.</param>
/// <param name="imageHeight">The width of the bitmap.</param>
/// <param name="pathToOutputFile">Full path to the output file.</param>
private void SaveControlImage(Visual baseElement,
int imageWidth, int imageHeight, string pathToOutputFile)
{
// 1) get current dpi
PresentationSource pSource = PresentationSource.FromVisual(Application.Current.MainWindow);
Matrix m = pSource.CompositionTarget.TransformToDevice;
double dpiX = m.M11 * 96;
double dpiY = m.M22 * 96; // 2) create RenderTargetBitmap
RenderTargetBitmap elementBitmap =
new RenderTargetBitmap(imageWidth, imageHeight, dpiX, dpiY, PixelFormats.Default); // 3) undo element transformation
DrawingVisual drawingVisual = new DrawingVisual();
using (DrawingContext drawingContext = drawingVisual.RenderOpen()) {
VisualBrush visualBrush = new VisualBrush(baseElement);
drawingContext.DrawRectangle(visualBrush, null,
new Rect(new Point(0, 0), new Size(imageWidth, imageHeight)));
} // 4) draw element
elementBitmap.Render(drawingVisual); // 5) create PNG image
BitmapEncoder encoder = new PngBitmapEncoder();
encoder.Frames.Add(BitmapFrame.Create(elementBitmap)); // 6) save image to file
using (FileStream imageFile =
new FileStream(pathToOutputFile, FileMode.Create, FileAccess.Write)) {
encoder.Save(imageFile);
imageFile.Flush();
imageFile.Close();
}
}Read more: devblog.NET
/// <param name="baseElement">The Visual object to be used as a bitmap. </param>
/// <param name="imageWidth">The height of the bitmap.</param>
/// <param name="imageHeight">The width of the bitmap.</param>
/// <param name="pathToOutputFile">Full path to the output file.</param>
private void SaveControlImage(Visual baseElement,
int imageWidth, int imageHeight, string pathToOutputFile)
{
// 1) get current dpi
PresentationSource pSource = PresentationSource.FromVisual(Application.Current.MainWindow);
Matrix m = pSource.CompositionTarget.TransformToDevice;
double dpiX = m.M11 * 96;
double dpiY = m.M22 * 96; // 2) create RenderTargetBitmap
RenderTargetBitmap elementBitmap =
new RenderTargetBitmap(imageWidth, imageHeight, dpiX, dpiY, PixelFormats.Default); // 3) undo element transformation
DrawingVisual drawingVisual = new DrawingVisual();
using (DrawingContext drawingContext = drawingVisual.RenderOpen()) {
VisualBrush visualBrush = new VisualBrush(baseElement);
drawingContext.DrawRectangle(visualBrush, null,
new Rect(new Point(0, 0), new Size(imageWidth, imageHeight)));
} // 4) draw element
elementBitmap.Render(drawingVisual); // 5) create PNG image
BitmapEncoder encoder = new PngBitmapEncoder();
encoder.Frames.Add(BitmapFrame.Create(elementBitmap)); // 6) save image to file
using (FileStream imageFile =
new FileStream(pathToOutputFile, FileMode.Create, FileAccess.Write)) {
encoder.Save(imageFile);
imageFile.Flush();
imageFile.Close();
}
}Read more: devblog.NET
Tips & Tricks: How to get the Screen Resolution in Silverlight ?
Posted by
jasper22
at
17:24
|
In this Tips & Tricks, I will show you the steps by which you can get the Screen Resolution of Client’s PC in Silverlight Application. It is quite simple. You have to just call the HTML DOM object to receive the handle of the screen and from that you can easily get the Screen Resolution.
To get the Screen Resolution, you need to get the handle of the Window and you can get it from:System.Windows.Browser.HtmlPage.Window;Read more: Kunal's Blog
To get the Screen Resolution, you need to get the handle of the Window and you can get it from:System.Windows.Browser.HtmlPage.Window;Read more: Kunal's Blog
6 new Windows 7 Themes for you to enjoy
Posted by
jasper22
at
17:23
|

Gears of War 3
26Creative
An Hsin Pu Tzu
(more..)Read more: I'm PC
Styling tips for common Silverlight controls
Posted by
jasper22
at
16:57
|
Microsoft Expression Blend comes with many Microsoft Silverlight controls that you can use to create a great user experience. You can also download controls from trusted sources.For more information, see Import a custom control by adding a reference. If you find a control that functionally fits your needs but doesn't look the way that you want it to, and if that control supports templates, you can modify the templates of the control to change its appearance. To make things easier, you can focus on your design by drawing everything on the artboard first, by using drawing tools, and by importing art or other assets. When you are done, you can use the Make Into Control command to convert your objects into a template for any control. Template-bindingControls have properties to which objects in a template can be bound. This is called template-binding. By binding parts of the template to a control, you effectively create parameters for the template. For example, instead of saying, "This Rectangle is blue," you say, "This Rectangle is the same color as the Background of the control." Thus, when you apply the template to a control with different Background properties, the result is a differently colored template. Read more: Microsoft Expression
Calling an asp.net web service from jQuery
Posted by
jasper22
at
16:10
|
As I have post it in earlier post that jQuery is one of most popular JavaScript library in the world amongst web developers Lets take a example calling ASP.NET web service with jQuery . You will see at the end of the example that how easy it is to call a web service from the jQuery. Let’s create a simple Hello World web service. Go to your project right click->Add –> New Item and select web service and add a web service like following. [WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class HelloWorld : System.Web.Services.WebService
{
[WebMethod]
public string PrintMessage()
{
return "Hello World..This is from webservice";
}
}...Now let’s add some JavaScript code to call web service methods like following. <script language="javascript" type="text/javascript">
function CallWebServiceFromJquery() {
$.ajax({
type: "POST",
url: "HelloWorld.asmx/PrintMessage",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
error: OnError
});
} function OnSuccess(data, status)
{
alert(data.d);
} function OnError(request, status, error)
{
alert(request.statusText);
}
</script>Read more: DOTNETJAPS ALL ABOUT .NET
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class HelloWorld : System.Web.Services.WebService
{
[WebMethod]
public string PrintMessage()
{
return "Hello World..This is from webservice";
}
}...Now let’s add some JavaScript code to call web service methods like following. <script language="javascript" type="text/javascript">
function CallWebServiceFromJquery() {
$.ajax({
type: "POST",
url: "HelloWorld.asmx/PrintMessage",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
error: OnError
});
} function OnSuccess(data, status)
{
alert(data.d);
} function OnError(request, status, error)
{
alert(request.statusText);
}
</script>Read more: DOTNETJAPS ALL ABOUT .NET
Smooth Streaming Primer
Posted by
jasper22
at
15:58
|
IIS Media Services, an integrated HTTP-based media delivery platform, delivers true HD (720p+) live and on-demand streaming, DVR functionality, and real-time analytics support to computers, TVs, and mobile devices. By offering a complete multi-format media delivery platform and a proven Web server, highly immersive websites can now be managed from a single Web platform: IIS. Smooth Streaming, an IIS Media Services extension, enables adaptive streaming of media to Silverlight and other clients over HTTP. Smooth Streaming provides a high-quality viewing experience that scales massively on content distribution networks (CDNs), making true HD 1080p media experiences a reality. Smooth Streaming is the productized version of technology first used by Microsoft to deliver on-demand video of the 2008 Summer Olympics for NBCOlympics.com. By dynamically monitoring both local bandwidth and video-rendering performance, Smooth Streaming optimizes content playback by switching video quality in real-time. Contents
Read more: IIS
- About IIS Smooth Streaming
- About the Protected Interoperable File Format (PIFF)
- Creating PIFF Content
- Enabling PlayReady DRM with Smooth Streaming
- Setting Up IIS Origin Servers for Live & On-Demand Smooth Streaming
- Enabling Server & Encoder Failover for Live Smooth Streaming
- Building a Smooth Streaming Client with Silverlight
- Building a Smooth Streaming Client for Embedded Platforms without Silverlight
- Getting Started with Expression Encoder, IIS Media Services, and Smooth Streaming to Silverlight Clients
- Setting Up Smooth Streaming to Apple Devices
- About the End-to-End Setup for Sunday Night Football & Winter Olympics Events
- Comparing Smooth Streaming to Other Adaptive Streaming Technologies
- FAQs (Coming Soon!)
Read more: IIS
Создание документации в .NET
Posted by
jasper22
at
15:57
|
Качественная документация – неотъемлемая часть успешного программного продукта. Создание полного и понятного описания всех функций и возможностей программы и программного компонента требует немало сил и терпения. В данной статье я рассмотрю некоторые практические аспекты создания документации для .NET компонентов. Предположим, что у нас готова или почти готова некоторая .NET библиотека для разработчиков (они же конечные пользователи). API библиотеки безупречен, количество багов впечатляюще мало, да и вообще это не библиотека, а просто кладезь совершенного кода. Дело за малым – объяснить пользователям, как работать с этим замечательным продуктом. Есть разные подходы к написанию документации. Некоторые команды предпочитают начинать создание документации в момент начала создания продукта. Другие откладывают написание мануалов на окончание работ. В некоторых командах документацию пишут специальные люди, которые ходят от разработчика к разработчику и от менеджера к менеджеру, аккумулируя знания о продукте. Во многих небольших командах таких специальных людей нет, а потому документацию часто пишет разработчик или разработчики. Кто-то использует сторонние средства вроде Help & Manual, в которых, как в заправском текстовом редакторе, можно создавать очень сложную верстку и на выходе получать документацию в многообразии форматов. Многие используют другой подход, широко пропагандируемый в последнее время – написание документации прямо в коде программы/библиотеки. Генерируем файл документацииПосле того, как xml-описание вашего компонента готово, можно сгенерировать файл документации. Я предпочитаю для этого использовать связку Sandcastle + Sandcastle Help File Builder (SHFB). Замечу, что некоторым больше по душе DocProject. Для этого требуется: Скачать и установить Sandcastle
sandcastle.codeplex.com/Скачать и установить Sandcastle Help File Builder
shfb.codeplex.com/ Скачать и применить патч для стилей, используемых Sandcastle
sandcastlestyles.codeplex.com/Если у вас возникнут проблемы со сборкой документации в формате HTML Help, то нужно проверить, что itircl.dll присутствует в системе и зарегистрирована. Обычно эта dll лежит в System32, регистрировать ее нужно через regsvr32. Подробнее написано тут:
frogleg.mvps.org/helptechnologies/htmlhelp/hhtips.html#hhc6003Read more: Habrahabr
sandcastle.codeplex.com/Скачать и установить Sandcastle Help File Builder
shfb.codeplex.com/ Скачать и применить патч для стилей, используемых Sandcastle
sandcastlestyles.codeplex.com/Если у вас возникнут проблемы со сборкой документации в формате HTML Help, то нужно проверить, что itircl.dll присутствует в системе и зарегистрирована. Обычно эта dll лежит в System32, регистрировать ее нужно через regsvr32. Подробнее написано тут:
frogleg.mvps.org/helptechnologies/htmlhelp/hhtips.html#hhc6003Read more: Habrahabr
Interesting T-SQL problems
Posted by
jasper22
at
15:55
|
With this blog post I am hoping to start a new series of blogs devoted to the interesting T-SQL problems I encounter in forums during the week.The idea of this blog series came to me on Wednesday night when I thought I solved a complex problem... First ProblemThe first problem, I'd like to discuss, is found in this MSDN thread:Given this tableSalesDateTime SalesAmount
2010-08-10 00:05:12 58.22
2010-08-10 00:08:22 21.10
2010-08-10 00:09:38 8.45
2010-08-10 00:18:04 9.52
2010-08-10 00:19:56 45.20
2010-08-10 11:35:15 47.12
2010-08-10 11:36:12 88.55
2010-08-10 11:40:31 45.12find the Average Sale amount for the 15 minutes time interval.Second Problem The second problem is really a gem and it is presented in How can I find overlapped ranges? threadName Chromosome Start End
N1 chr3 17443613 17443685
N2 chr3 17443521 17443685
N3 chr2 162180459 162180499
N343 chr2 131865573 131865687
N34 chr16 34623393 34623610
N2456 chr3 17443512 17443685
N43243 chr3 17443608 17443685
The whole table is about 31,000 records (includes 22 chromosomes + X, Y chromosomes). The problem is to find the overlapped regions (or ranges) and these overlapped records have to be on the same Chromosome of this table.Read more: LessThanDot
2010-08-10 00:05:12 58.22
2010-08-10 00:08:22 21.10
2010-08-10 00:09:38 8.45
2010-08-10 00:18:04 9.52
2010-08-10 00:19:56 45.20
2010-08-10 11:35:15 47.12
2010-08-10 11:36:12 88.55
2010-08-10 11:40:31 45.12find the Average Sale amount for the 15 minutes time interval.Second Problem The second problem is really a gem and it is presented in How can I find overlapped ranges? threadName Chromosome Start End
N1 chr3 17443613 17443685
N2 chr3 17443521 17443685
N3 chr2 162180459 162180499
N343 chr2 131865573 131865687
N34 chr16 34623393 34623610
N2456 chr3 17443512 17443685
N43243 chr3 17443608 17443685
The whole table is about 31,000 records (includes 22 chromosomes + X, Y chromosomes). The problem is to find the overlapped regions (or ranges) and these overlapped records have to be on the same Chromosome of this table.Read more: LessThanDot
LINQ To XML Tutorials with Examples
Posted by
jasper22
at
15:55
|
A lot of developers over the past few months have requested us for tutorials focusing on LINQToXML. Although I have written a couple of them in the past, I decided to republish these tips in the form of a single post. In this article, we will explore 24 ‘How Do I’ kind of examples using LINQ to XML. I assume you are familiar with LINQ. If not, you can start off with LINQ by checking some tutorials over here and here.
For this article, we will be using a sample file called ‘Employees.xml’ for all our samples which is available with the source code. So make sure you keep it handy with you while are practicing these examples. The mark up for Employees.xml is as follows: <?xml version="1.0" encoding="utf-8" ?>
<Employees>
<Employee>
<EmpId>1</EmpId>
<Name>Sam</Name>
<Sex>Male</Sex>
<Phone Type="Home">423-555-0124</Phone>
<Phone Type="Work">424-555-0545</Phone>
<Address>
<Street>7A Cox Street</Street>
<City>Acampo</City>
<State>CA</State>
<Zip>95220</Zip>
<Country>USA</Country>
</Address>
</Employee>
<Employee>
<EmpId>2</EmpId>
<Name>Lucy</Name>
<Sex>Female</Sex>
<Phone Type="Home">143-555-0763</Phone>
<Phone Type="Work">434-555-0567</Phone>
<Address> Read more: dot net curry.com
<Employees>
<Employee>
<EmpId>1</EmpId>
<Name>Sam</Name>
<Sex>Male</Sex>
<Phone Type="Home">423-555-0124</Phone>
<Phone Type="Work">424-555-0545</Phone>
<Address>
<Street>7A Cox Street</Street>
<City>Acampo</City>
<State>CA</State>
<Zip>95220</Zip>
<Country>USA</Country>
</Address>
</Employee>
<Employee>
<EmpId>2</EmpId>
<Name>Lucy</Name>
<Sex>Female</Sex>
<Phone Type="Home">143-555-0763</Phone>
<Phone Type="Work">434-555-0567</Phone>
<Address> Read more: dot net curry.com
HelpProvider in C#
Posted by
jasper22
at
15:45
|
HelpProvider HelpProvider control provides popup or online help for a control. In this article, we will discuss how to use a HelpProvider control to implement help for controls in a Windows Forms application. Here are some of the useful methods of HelpProvider.SetShowHelp method specifies whether Help is displayed for the specified control.SetHelpString method specifies the Help string associated with the specified control. SetHelpNavigator method specifies the Help command to use when retrieving Help from the Help file for the specified control.SetHelpKeyword method specifies the keyword used to retrieve Help when the user invokes Help for the specified control. HelpNamspace property gets or sets a value specifying the name of the Help file associated with this HelpProvider.Here is an example of how to use a HelpProvider component and load a help (.chm) file and apply on the controls. C# Code:private HelpProvider hlpProvider; private void CreateHelpProvider()
{
hlpProvider = new System.Windows.Forms.HelpProvider();
hlpProvider.SetShowHelp(textBox1, true);
hlpProvider.SetHelpString(textBox1, "Enter a valid text here."); hlpProvider.SetShowHelp(button1, true);
hlpProvider.SetHelpString(button1, "Click this button."); // Help file
hlpProvider.HelpNamespace = "helpFile.chm"; hlpProvider.SetHelpNavigator(textBox1, HelpNavigator.TableOfContents);
}Read more: C# Corner
{
hlpProvider = new System.Windows.Forms.HelpProvider();
hlpProvider.SetShowHelp(textBox1, true);
hlpProvider.SetHelpString(textBox1, "Enter a valid text here."); hlpProvider.SetShowHelp(button1, true);
hlpProvider.SetHelpString(button1, "Click this button."); // Help file
hlpProvider.HelpNamespace = "helpFile.chm"; hlpProvider.SetHelpNavigator(textBox1, HelpNavigator.TableOfContents);
}Read more: C# Corner
Debug Your .NET Web Project With IIS Express [Tips & Tricks]
Posted by
jasper22
at
12:21
|
For those of us too impatient to wait for a hotfix for Visual Studio to natively support IIS Express, I've done some digging and found a way to [fairly] easily setup a debugging environment for IIS Express and VS 2010 (should work for VS 2008 also, though!). This assumes you are at least an intermediate user of .NET/IIS and Visual Studio. Prerequisites
Download and install WebMatrix beta. This download includes IIS Express (as of this posting, I did not find a standalone download of IIS Express).
Be using Visual Studio 2010 or 2008 and a web project to debug. Steps to Setup IIS ExpressIt's actually quite simple to setup IIS express. Once WebMatrix is done installing, go to "My Documents\IISExpress8\config".
Read more: InterPID
Download and install WebMatrix beta. This download includes IIS Express (as of this posting, I did not find a standalone download of IIS Express).
Be using Visual Studio 2010 or 2008 and a web project to debug. Steps to Setup IIS ExpressIt's actually quite simple to setup IIS express. Once WebMatrix is done installing, go to "My Documents\IISExpress8\config".

Read more: InterPID
Stepping into ASP.NET MVC source code with Visual Studio debugger
Posted by
jasper22
at
12:20
|
Using Visual Studio symbols and source files makes debugging much easier. I am specially happy about ASP.NET MVC 2 source files because I develop on ASP.NET MVC 2 almost every day. You may also find other useful symbols and source files. In this posting I will show you how to get ASP.NET MVC source to your computer and how to use it. 1. Debug options
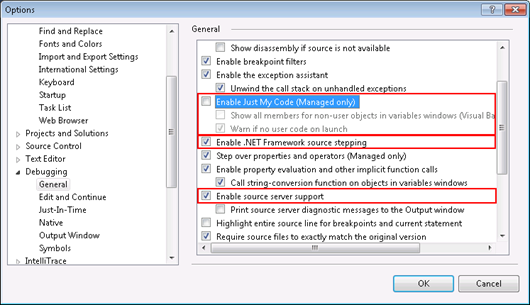
Read more: Gunnar Peipman's ASP.NET blog
Open options dialog and make sure you have check boxes set as on following image in red boxes.
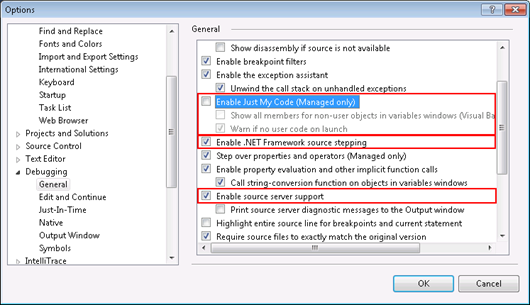
MvcContrib Template Gallery
Posted by
jasper22
at
12:19
|
Project Description
This project is the MVC Template Gallery which is part of the MvcContrib project.This gallery is filled with project styles that work with the out of the box markup from the ASP.Net MVC project template. These were origionally part of the www.asp.net website but went away after a site redesign. Since they were licensed under a Creative Commons licences, we put them up here and hope to have more submitted by great developers and designers like you! Read more: Codeplex
This project is the MVC Template Gallery which is part of the MvcContrib project.This gallery is filled with project styles that work with the out of the box markup from the ASP.Net MVC project template. These were origionally part of the www.asp.net website but went away after a site redesign. Since they were licensed under a Creative Commons licences, we put them up here and hope to have more submitted by great developers and designers like you! Read more: Codeplex
Managing View State in ASP.NET 4 Using the New ViewStateMode Property
Posted by
jasper22
at
12:18
|
Introduction
The ASP.NET Web Forms model strives to encapsulate the lower level complexities involved in building a web application. Features like server-side event handlers, the page lifecycle, and view state effectively blur the line between the client and the server, simplify state management, and free the developer from worrying about HTTP, requests and responses, and similar matters. While these facets of the Web Forms model allow for rapid application development and make ASP.NET more accessible to developers with a web application background, their behavior can impact your website's behavior and performance. View state is perhaps the most important - yet most misunderstood - feature of the Web Forms model. In a nutshell, view state is a technique that automatically persists programmatic changes to the Web controls on a page. By default, this state is serialized into a base-64 encoded string and included as a hidden <input> field in the Web Form. On postback, this state information is returned to the server as part of the POST request, at which point the server can deserialize it and reapply the persisted state to the controls in the control hierarchy. (If this last paragraph made crystal clear sense, great! If not, consider reading my article, Understanding ASP.NET View State, and Dave Reed's article, ViewStateMode in ASP.NET 4, before continuing.) One potential issue with view state is that it can greatly bloat the size of your web pages. Each new version of ASP.NET seems to include new techniques for managing view state's footprint. ASP.NET 4 adds a new property to all Web controls, ViewStateMode, which allows developers to disable view state for a page by default and then selectively enable it for specific controls. This article reviews existing view state-related properties and then delves into the new ViewStateMode property. Read on to learn more! Read more: 4 Guys From Rolla
The ASP.NET Web Forms model strives to encapsulate the lower level complexities involved in building a web application. Features like server-side event handlers, the page lifecycle, and view state effectively blur the line between the client and the server, simplify state management, and free the developer from worrying about HTTP, requests and responses, and similar matters. While these facets of the Web Forms model allow for rapid application development and make ASP.NET more accessible to developers with a web application background, their behavior can impact your website's behavior and performance. View state is perhaps the most important - yet most misunderstood - feature of the Web Forms model. In a nutshell, view state is a technique that automatically persists programmatic changes to the Web controls on a page. By default, this state is serialized into a base-64 encoded string and included as a hidden <input> field in the Web Form. On postback, this state information is returned to the server as part of the POST request, at which point the server can deserialize it and reapply the persisted state to the controls in the control hierarchy. (If this last paragraph made crystal clear sense, great! If not, consider reading my article, Understanding ASP.NET View State, and Dave Reed's article, ViewStateMode in ASP.NET 4, before continuing.) One potential issue with view state is that it can greatly bloat the size of your web pages. Each new version of ASP.NET seems to include new techniques for managing view state's footprint. ASP.NET 4 adds a new property to all Web controls, ViewStateMode, which allows developers to disable view state for a page by default and then selectively enable it for specific controls. This article reviews existing view state-related properties and then delves into the new ViewStateMode property. Read on to learn more! Read more: 4 Guys From Rolla
Listening to DependencyProperty changes in Silverlight
Posted by
jasper22
at
12:17
|
The dependency property system is a pretty nice concept. Receiving notifications for dependency property changes on an existing object is a very common scenario in order to update my view model or the UI. This is quite easy in WPF:
Unfortunately Silverlight has a limited set of meta-data functionality around the dependency property system, because the DependencyPropertyDescriptor does exist in Silverlight. In order to get a workaround I found a solution in which I get notified with help of the binding system. I simple use a relay object which value property is bound to the source property I want to get notified. The relay object contains a public event which raises when the value changes. Read more: Kiener's Blog
// get the property descriptor
DependencyPropertyDescriptor prop = DependencyPropertyDescriptor.FromProperty(TextBox.TextProperty, myTextBox.GetType());
// add change handler
prop.AddValueChanged(myTextBox, (sender, args) =>
{
});
Unfortunately Silverlight has a limited set of meta-data functionality around the dependency property system, because the DependencyPropertyDescriptor does exist in Silverlight. In order to get a workaround I found a solution in which I get notified with help of the binding system. I simple use a relay object which value property is bound to the source property I want to get notified. The relay object contains a public event which raises when the value changes. Read more: Kiener's Blog
Run Any Browser Directly from the Web
Posted by
jasper22
at
09:25
|
Want to quickly test your website in IE 6 or Firefox 2 or Google Chrome 5 or Safari 4 or Opera? Spoon is a cool website that lets you run specific versions of the popular web browsers directly in your web browser via a plugin. Great for geeks like me. Read more: Spoon Browser Sandbox
Hackers accidentally give Microsoft their code
Posted by
jasper22
at
09:24
|
When hackers crash their systems while developing viruses, the code is often sent directly to Microsoft, according to one of its senior security architects, Rocky Heckman.When the hacker's system crashes in Windows, as with all typical Windows crashes, Heckman said the user would be prompted to send the error details--including the malicious code--to Microsoft. The funny thing is that many say yes, according to Heckman. "People have sent us their virus code when they're trying to develop their virus and they keep crashing their systems," Heckman said. "It's amazing how much stuff we get."At a Microsoft Tech.Ed 2010 conference session on hacking today, Heckman detailed to the delegates the top five hacking methods and the best methods for developers to avoid falling victim to them. Heckman explained how to create malicious code that could be used in cross-site scripting or SQL injection attacks and, although he said it "wasn't anything you couldn't pick up on the Internet", he suggested delegates use the code responsibly to aid in their protection efforts. According to Heckman, based on the number of attacks on Microsoft's website, the company was only too familiar with what types of attacks were most popular."The first thing [script kiddies] do is fire off all these attacks at Microsoft.com," he said. "On average we get attacked between 7000 and 9000 times per second at Microsoft.com," said the senior security architect. "I think overall we've done pretty good, even when MafiaBoy took down half the Internet, you know, Amazon and eBay and that, we didn't go down, we were still up."Read more: ZDnet
Subscribe to:
Posts (Atom)