How to Implement MVVM, INotifyChanged and ICommand in a Silverlight Application
...
INotifyChanged
By implementing the INotifyChanged interface (or inheriting from a base class which implements it), events can be raised when a property value changes. For the calculator example events will be used to update the user interface when the values for the two input numbers or the calculated result change. The code below shows how the OnPropertyChanged method is called in the property setters to raise the PropertyChangedEventHandler.
private string _firstValue;
private string _secondValue;
private string _result;
public event PropertyChangedEventHandler PropertyChanged;
public string FirstValue
{
get { return _firstValue; }
set
{
_firstValue = value;
OnPropertyChanged("FirstValue");
}
}
public string SecondValue
{
get { return _secondValue; }
set
{
_secondValue = value;
OnPropertyChanged("SecondValue");
}
}
public string Result
{
get { return _result; }
private set
{
_result = value;
OnPropertyChanged("Result");
}
}
protected void OnPropertyChanged(string propertyName)
{
if (PropertyChanged != null)
{
PropertyChanged(this,
new PropertyChangedEventArgs(propertyName));
}
}
Read more: Arrange act assert
Create And Share Panoramic Photos With 360 For Android
Posted by
jasper22
at
10:32
|

New to the Android Market, 360 is yet another commendable tool for casual photography enthusiasts. The app lets you capture HD panoramic photographs with your Android device and share them with your social media (Facebook, Twitter) contacts and other 360 users, complete with geotagging. You can also view panoramas uploaded by 360 users from all around the globe or those in your vicinity from within the app.
In our brief test-run, we found the method for capturing panoramas to be taxing yet rewarding. It takes quite a lot of time and patience to capture a flawless panorama but the result is usually well worth the effort, quite satisfying and a treat to view.
Read more: Addictive tips
MiMedia Offers 7 GB Cloud Storage To Sync And Backup Data
Posted by
jasper22
at
10:03
|
Are you running short of Dropbox storage space and looking for a cloud-based service with Dropbox like features and capabilities? You can sign up for freshly released MiMdedia service, which unlike many other cloud based storage services, offers 7 GB for absolutely free with options to watch and stream saved media files, backup multiple PCs automatically, and categorize photos, documents, and music into groups. Just like Dropbox and many other cloud storages, it allows user to share their files with friends and family in simple yet intuitive manner.
Taking an offbeat approach to backup, it first asks you to specify all important data which you can’t afford to lose at any cost. You are presented with a list of drives from where folders are to be selected. Once backup folders are specified, it connects your PC with online server in order to backup selected folders to its server. Using Shuttle Drive is, however, recommended, but you can use broadband service prior to allowing high bandwidth usage to lessen the time required for backup process.
MiMedia is more like your online personal media manger. After you’ve uploaded favorite media files, you can stream them from anywhere and share them as well. Media buffs also have sorting options to quickly access required files without having to navigate through a big media file stack.
Read more: Addictive Tips
Read more: MiMedia
Google Announces Chromebooks For Business, At $28 Monthly Per User
Posted by
jasper22
at
09:41
|
Google Product VP Sundar Pichai announced today at Google I/O that the Chromebooks functionality for consumers will be expanded for businesses. According to Pichai, more than 50% of IT companies today are still running the ten-year-old Windows XP software despite the fact that it will be phased out in 2014. In addition, that fact that users are increasingly using their laptops to move in and out of the company firewalls has created problems for CIOs and IT admins.
Said Pichai, “Many companies have a hard time upgrading OS and most users use laptops today. They take them in and out of enterprise firewalls and this is challenging for IT admins. CIOs and IT admins are moving computing to the cloud.”
Because of these challenges Pichai announced that Google will be launching Chromebooks for Business, a software and hardware package tailored to the needs of businesses.
In preparation for this, Google has partnered with Citrix and VMWare to provide business apps for Chromebooks and has tested the service with businesses like Jason’s Deli and the City of Orlando.
Read more: Techcrunch
Keyboard Hooking In Kernel
Posted by
jasper22
at
09:05
|
Introduction
Please imagine that there's no input signal from the keyboard. It can be used to secure a computer from anybody. For example, if you leave the computer, you can lock the keyboard in this way. After returning to your computer, you can type specific keys to unlock the computer. So the other man who doesn't know your secret password can't access your computer. This can be implemented through Keyboard Hooking.
What is keyboard hooking?
We also called it keyboard filtering. And it can be explained in a simple manner. The user presses the key and from Keyboard device driver sends signals to User's application. And we hook the stream of that.
Keyboard hooking can be accomplished in several ways. In Userland and Kernelland.
You can see userland sample from Adam Roderick J's article.
He shows keyboard hooking skills in userland and few articles also explain keyboard hooking. But using this technique, you can't hook several times by several applications and it can also be uninstalled easily. So I'll introduce another way to hook keyboard in Kernelland. I focused on Driver Hooking in this article. You can not only stop key signals, but also modify signals from User by using this technique, then it can become a virus or malware. But I don't want that. Then how can it be implemented? Let's see together.
How To Use
Place Application.exe and HK_KBD.sys in the same folder, and execute Application.exe. Then press any key. You can see that there's no input from key. OK! That's it!
Using the Code
First, we should find keyboard device object handle. In order to do, you should list all the devices of your computer. And from them, you should find Keyboard Class Device.
You can implement that in the following way:
Collapse
NTSTATUS
KeyFlt_CreateClose(
IN PDEVICE_OBJECT DeviceObject,
IN PIRP Irp
)
{
...
switch (stack->MajorFunction)
{
case IRP_MJ_CREATE:
{
...
RtlInitUnicodeString(&uniOa, L"\\Device");
InitializeObjectAttributes(
&oa,
&uniOa,
OBJ_CASE_INSENSITIVE,
NULL,
NULL
);
status = ZwOpenDirectoryObject(
&hDir,
DIRECTORY_ALL_ACCESS,
&oa
);
if(!NT_SUCCESS(status))
{
break;
}
pBuffer = ExAllocatePoolWithTag
(PagedPool, ALLOC_SIZE, Tag);
Read more: Codeproject
Why double-null-terminated strings instead of an array of pointers to strings?
Posted by
jasper22
at
09:01
|
I mentioned this in passing in my description of the format of double-null-terminated strings, but I think it deserves calling out.
Double-null-terminated strings may be difficult to create and modify, but they are very easy to serialize: You just write out the bytes as a blob. This property is very convenient when you have to copy around the list of strings: Transferring the strings is a simply memory of transferring the memory block as-is. No conversion is necessary. This makes it easy to do things like wrap the memory inside another container that supports only flat blobs of memory.
As it turns out, a flat blob of memory is convenient in many ways. You can copy it around with memcpy. (This is important when doing capturing values across security boundaries.) You can save it to a file or into the registry as-is. It marshals very easily. It becomes possible to store it in an IDataObject. It can be freed with a single call. And in the cases where you can't allocate any memory at all (e.g., you're filling a buffer provided by the caller), it's one of the few options available. This is also why self-relative security descriptors are so popular in Windows: Unlike absolute security descriptors, self-relative security descriptors can be passed around as binary blobs, which makes them easy to marshal, especially if you need to pass one from kernel mode to user mode.
Read more: The old new thing
Silverlight Application with WCF Service and Child Windows as Popups - Part 1
Posted by
jasper22
at
08:57
|
Introduction
In this application, I create a meeting scheduler using which one can schedule a meeting and call other colleagues to attend the meeting. This application uses the WCF services to fetch and save records. I have also used Silverlight child window to get the saved contacts to send mail to them. Child window is used as popup for adding contacts in the scheduler forms. This application is very useful for all those to just started learning Silverlight and have confusion with the basics of its interaction with database only through WCF services or webservices.
Tools and Technologies Used to Develop this Application
Visual Studio 2010
C# 4.0
Silverlight 4
SQL Server 2008
WCF Services
Step by Step Description of this Application
Create a new Silverlight application in Visual Studio 2010 File->New->Project -> select Silverlight in Visual C# -> select Silverlight Application. Rename it to Meetingschedule and click on ok. A popup window is prompted, click ok for that too.
You can see two projects in the solution explorer; one is the Silverlight project and another is the web project which is used to consume the Silverlight application.
Now our main purpose is to design the form in MainPage.xaml in Silverlight application. Below, you can see the XAML code for the form design, also how this design should be seen in the browser.
Read more: Codeproject
Another decompiler comes online - dotPeek from JetBrains
Posted by
jasper22
at
08:56
|
What's Cool about dotPeek?
Decompiling .NET 1.0-4.0 assemblies to C#
Quick jump to a specific type, assembly, symbol, or type member
Effortless navigation to symbol declarations, implementations, derived and base symbols, and more
Accurate search for symbol usages
with advanced presentation of search results
Overview of inheritance chains
Support for downloading code from source servers
Syntax highlighting
Complete keyboard support
dotPeek is free!
..."
I think we, the .Net Development Community, owe a word of thanks to RedGate. Their moves with Reflector have kicked the .Net dev ant hill and have caused a wave of development that I've not seen for a while...
Read more: Greg's Cool [Insert Clever Name] of the Day
Read more: JetBrains dotPeek EAP is Open!
The command prompt has been disabled by your Administrator
שלום לכולם,
כאן דן ויזנפלד מצוות התמיכה של Microsoft.
בעת ניסיון טעינת ממשק שורת הפקודה (CMD), ייתכן ונתקלתם בהודעת השגיאה:
"The command prompt has been disabled by your Administrator"

אם אינכם יודעים מיהו מנהל הרשת שלכם ( The Administrator) או אינכם יודעים אם יש לכם בכלל אחד כזה, אז מקור ההודעה הוא כפי שאמרה לכם תחושת הבטן שלכם: וירוס, או תוכנה זדונית אחרת.
התהליך לפתרון הבעיה יתבצע בשני רבדים:
1. הסרת המזיק
א. הורידו את הכלי של Microsoft להסרת תוכנות זדוניות, שנקרא MSERT, ובצעו סריקה מקיפה למחשב שלכם. שימו לב! במידה והמזיק חסם את הגישה של המחשב לאינטרנט, ניתן להוריד את הכלי במחשב אחר ולהעבירו באמצעות דיסק אחרון למחשב הנגוע.
ב. לאחר הסרת כלל הקבצים הנגועים, אמליץ לכם להפעיל מחדש את המחשב ולאחר מכן להתקין את האנטי-וירוס החינמי של Microsoft אשר נקרא Microsoft Security Essentials.
2. טיפול נקודתי בבעיה
ייתכן והסרת המזיק לא בהכרח תפתור את הבעיה אשר הוא יצר (אמנם המקור לבעיה טופל, אך הנזק כבר בוצע). לשם כך, יש לבצע טיפול נקודתי לבעיה. הטיפול הנקודתי יכול להתבצע בשתי דרכים – באמצעות עורך הרישום, או באמצעות ממשק המדיניות הקבוצתית של Windows.
שימו לב שעליכם להיות בעלי חשבון עם הרשאות מנהל לשם ביצוע הפעולות.
א. שימוש בעורך הרישום
(1) לחצו בו-זמנית במקלדת על המקשים Start (מקש התחל, הידוע גם בכינויים Winkey ומקש Windows) ו-R.
(2) בחלון שנפתח הזינו את הפקודה regedit ולאחר מכן לחצו על אישור (OK).
Read more: MS Support blog
Google Storage for Developers open to all, with new features
Posted by
jasper22
at
10:46
|
For those of you who have been waiting to use Google Storage, we’re happy to announce that effective immediately, you can get a Google Storage for Developers account without needing to request an invitation.
We’ve also launched several significant enhancements to the service, including more flexible and powerful security features, simplified sharing, the ability to store data in Europe, support for larger objects (up to 5 TB), team-oriented accounts and a completely free promotional tier.
OAuth 2.0 Support
OAuth 2.0 is the new recommended Google Storage authentication and authorization scheme. OAuth 2.0 is an industry standard that we’ve adopted across Google, offering many benefits:
Simpler - never sign a request again! OAuth 2.0 uses access tokens for authentication, which obviate the need for complicated signature schemes. We recommend that all OAuth 2.0-authenticated requests be made over SSL.
More flexible and powerful - OAuth 2.0 allows for three-legged authentication, where a user can grant an application permission to access Google Storage on their behalf (and revoke the grant at any time if necessary).
Read more: Google code blog
Streamline your web font requests: introducing “text=”
Posted by
jasper22
at
10:44
|
Last week, the Google Web Fonts team announced a new feature on the Google Web Fonts Blog. Since we’re discussing this feature today at Google I/O, we’d like to share this news with Google Code Blog readers as well.
Oftentimes, when you want to use a web font on your website or application, you know in advance which letters you’ll need. This often occurs when you’re using a web font in a logo or heading.
That’s why we’re introducing a new beta feature to the Google Web Fonts API. The feature is called “text=”, and allows you to specify which characters you’ll need. To use it, simply add “text=” to your Google Web Fonts API requests. Here’s an example:
<link href='http://fonts.googleapis.com/css?family=Special+Elite &text=MyText' rel='stylesheet' type='text/css'>
Google will optimize the web font served based on the contents of this parameter. For example, if you only require a few letters for a logo, such as “MyText”, Google will return a font file that is optimized to those letters. Typically, that means Google will return a font file that contains only the letters you requested. Other times, Google might return a more complete font file, especially when that will lead to better caching performance.
Read more: Google code blog
FTP directly in SQL (Using SQL CLR)
Posted by
jasper22
at
10:43
|
Introduction
Working with a complex SQL environment which used a multitude of technologies, one of the problems we encountered was using SQL Jobs to transfer files via FTP.
The existing SQL Jobs in our environment used an external application (the command line application ftp.exe) called via the xp_cmdshell extended stored procedure, to transfer the files to the FTP servers.
It worked most of the time but when it didn't there was no easy way to trace the failures directly within SQL.
Transact-SQL can be powerful and fun to use, but it has some limitations and lacks the advantages of managed code.
The solution I've provided allows for better handling of code... Drum roll for CLR Stored Procedures...
CLR Stored Procedures
Defining a CLR Stored Procedure allows one to utilise managed code from within SQL. This gives almost infinite possibilities in extending the functionality of SQL Stored Procedures by leveraging the .NET Framework with your favorite language such as C# or VB.NET.
By using a CLR Stored Procedure which calls the function defined in the pre-compiled .NET DLL, we were able to transfer files via FTP to and from FTP Servers directly from a SQL stored procedure and catch and handle exceptions and finally return the results to SQL variables.
Leveraging this, we used a trace table to record when a file was successfully uploaded or downloaded and if not we recorded the error to assist with debugging/troubleshooting for the SQL Database Administrators.
This solution was inspired by the article CLR Stored Procedure and Creating It Step by Step which was written by Virat Kothari.
Getting Started
This is a high-level overview of the steps taken to create the CLR Stored Procedure.
Create a Visual Studio Project as a Class Library.
Decorate functions to be exposed with [Microsoft.SqlServer.Server.SqlFunction].
Compile Project in and copy .DLL file to the appropriate folder.
Register Assembly from within SQL Server Management Studio:
CREATE ASSEMBLY clr_sqlFTP AUTHORIZATION dbo
FROM 'c:\Windows\System32\sqlFTP.dll'
WITH PERMISSION_SET = UNSAFE
Read more: Codeproject
Demystifying USB Selective Suspend
Posted by
jasper22
at
10:42
|
Run-Time Power Management
Before we talk about selective suspend, let’s understand a more generic concept: run-time power management.
One way of conserving power in a system is to send the whole system to a low-power state such as sleep or hibernate. Because this mechanism requires turning off the system, it is only possible when the whole system is not in use. Even when the whole system is in use and is in working state, it is quite likely that certain components of the system are not active. Those components are said to be in an idle state. Run-time power management refers to sending idle components to a lower power state, until they need to be used again. The components can be hardware such as processor, memory, and so on; however in this discussion, we are only interested in run time power management of devices.
Devices and device drivers should aggressively pursue run-time power management of devices because the mechanism can lead to significant power savings. Because a driver stack includes more than one driver, coordination between drivers is required while sending the device to a lower power state and bringing it back to working state. Both Windows Driver Model (WDM) and Kernel-Mode Driver Framework provide mechanisms for this coordination. One driver in the device stack, typically the function driver, is the power policy owner. This power policy owner is responsible for detecting that the device is idle and initiating the process of transitioning the device into a lower power state. The power policy owner is also responsible for bringing the device back to working state, (also referred to as waking up the device) when the user needs to use that device.
Host-Initiated and Device-Initiated Wake-up
How would the power policy owner driver know about the user’s intent to use a device, so that the driver can wake up the device? That depends on the kind of device. Let us say, a storage device that is in a low-power state, and a user needs to transfer a file from or to that device. When the user initiates the transfer process (by using a certain application), the power policy owner gets an I/O request from the application, and knows that it needs to wake up the device. However, if the device is a mouse, the device must send some sort of signal to initiate the wake-up process. The device-initiated power transition is known as remote wake-up feature in the USB world. Because the mouse needs to generate such a resume signal, it cannot completely turn itself off. Typically, the ability of the device to generate the resume signal is programmable. Therefore, before putting the device to sleep, the driver must instruct the device to turn on the remote wake-up feature when the device is suspended. This process is called arming the device for remote wake-up.
Read more: Microsoft Windows USB Core Team Blog
Setting up central Mercurial server in Ubuntu
Posted by
jasper22
at
10:39
|
It’s been a while since we’ve been thinking about moving to Mercurial from Subversion at our company. We were convinced about the benefits of DVCS and chose Mercurial over Git. However, due to pressure at work and some procrastination, the move was delayed a couple times. Finally, today we had the move and the team is already up to speed with it.
Although it’s quite simple to install it in a central server, the different articles on the web had me confused with too many options or with a different distro than Ubuntu. So, here is a dead simple list of things to do for installing Mercurial centrally for your dev team.
1. Log onto your server using SSH
2. Install mercurial from repository:
sudo apt-get update
sudo apt-get install mercurial
3. We need to have a directory to store our Mercurial configuration and repository files, so let’s create one and change it’s owner to the apache user so that apache can access them:
cd /var/
sudo mkdir hg
sudo mkdir hg/repos
sudo chown -R www-data:www-data hg/repos
Read more: Emran Hasan's Blog
Understanding the Content Controls in Silverlight
Posted by
jasper22
at
10:38
|
Once you get in touch with the Silverlight Templated controls it became evident that there are three kind of controls you can put in the XAML. Given that the structure of the page is a hierarchical tree - for this reason is is commonly called Visual Tree - you can expand this paradigm to understand the three types of controls.
"Leaf" controls are classes inherited from Control and they terminate the hierarchy because nothing can be inserted into them
"Content" controls are inherited from the ContentControl class. These controls can host other elements inside of them. They are nodes in the hierarchy
"Items" controls are usually inherited from ItemsControl and they can contain multiple instances of child controls so they are also a particular case of nodes with many branches starting from it.
The boundary between one kind and the other is not always immediately evident when you use a control. As an example, if you consider the Button control at the first sight is may seems a leaf control but instead it is a Content control and thanks to this you can have button that hosts images and texts and not only a label.
When you start creating a templated control the choice of the class from which inheriting is really important and you have to make the choice carefully because this choice can impact the effectiveness and reusability of the control. Many times the choice of inheriting from Control is good, but you have always to try to give a chance to the ContentControl, reasoning about the final structure you want to achieve. Content control can often give an additional gear to your work paying an often low price in when you first create the control layout.
Creating a ContentControl from scratch
Download the source code
The first time you create a control, it is really hard to deal with the concept of content. It seems there is nothing you can't do inheriting from the simple Control class, exposing a bunch of properties to set the various aspect of the control. For the purpose of this article we will create a Balloon control similar to the ones it is usual to find in chat clients, containing the messages exchanged between the parts. The control is basically a rectangle with a spike and at the first sight it may seem it suffice to inherit from Control and expose a property "Text" to set the content of the baloon. The solution can work fine but the first time we have to put an emoticon into the chat the things become immediately hard. Given that the "Text" property can contain a string it is very difficult to put the image of the emoticon inside of the balloon because you have to write a sort of parser to detect the emoticon inside of the text and change them to an image. The things become much more complicated if you want to support image attachments, different fonts, text styles and so on.
Read more: Silverlight Show
Using dynamic WCF service routes
Posted by
jasper22
at
10:35
|
For a demo I am working on, I’m creating an OData feed. This OData feed is in essence a WCF service which is activated using System.ServiceModel.Activation.ServiceRoute. The idea of using that technique is simple: map an incoming URL route, e.g. “http://example.com/MyService” to a WCF service. But there’s a catch in ServiceRoute: unlike ASP.NET routing, it does not support the usage of route data. This means that if I want to create a service which can exist multiple times but in different contexts, like, for example, a “private” instance of that service for a customer, the ServiceRoute will not be enough. No support for having http://example.com/MyService/Contoso/ and http://example.com/MyService/AdventureWorks to map to the same “MyService”. Unless you create multiple ServiceRoutes which require recompilation. Or… unless you sprinkle some route magic on top!
Implementing an MVC-style route for WCF
Let’s call this thing DynamicServiceRoute. The goal of it will be to achieve a working ServiceRoute which supports route data and which allows you to create service routes of the format “MyService/{customername}”, like you would do in ASP.NET MVC.
First of all, let’s inherit from RouteBase and IRouteHandler. No, not from ServiceRoute! The latter is so closed that it’s basically a no-go if you want to extend it. Instead, we’ll wrap it! Here’s the base code for our DynamicServiceRoute:
public class DynamicServiceRoute : RouteBase, IRouteHandler
{
private string virtualPath = null;private ServiceRoute innerServiceRoute = null;private Route innerRoute = null;public static RouteData GetCurrentRouteData(){}public DynamicServiceRoute(string pathPrefix, object defaults, ServiceHostFactoryBase serviceHostFactory, Type serviceType){}public override RouteData GetRouteData(HttpContextBase httpContext){}public override VirtualPathData GetVirtualPath(RequestContext requestContext, RouteValueDictionary values){}public System.Web.IHttpHandler GetHttpHandler(RequestContext requestContext){}
}
As you can see, we’re creating a new RouteBase implementation and wrap 2 routes: an inner ServiceRoute and and inner Route. The first one will hold all our WCF details and will, in one of the next code snippets, be used to dispatch and activate the WCF service (or an OData feed or …). The latter will be used for URL matching: no way I’m going to rewrite the URL matching logic if it’s already there for you in Route.
Read more: Maarten Balliauw {blog}
Optional argument corner cases, part one
Posted by
jasper22
at
10:32
|
In C# 4.0 we added "optional arguments"; that is, you can state in the declaration of a method's parameter that if certain arguments are omitted, then constants can be substituted for them:
void M(int x = 123, int y = 456) { }
can be called as M(), M(0) and M(0, 1). The first two cases are treated as though you'd said M(123, 456) and M(0, 456) respectively.
This was a controversial feature for the design team, which had resisted adding this feature for almost ten years despite numerous requests for it and the example of similar features in languages like C++ and Visual Basic. Though obviously convenient, the convenience comes at a pretty high price of bizarre corner cases that the compiler team definitely needs to deal with, and customers occasionally run into by accident. I thought I might talk a bit about a few of those bizarre corner cases.
First off, a couple having to do with interfaces. Let's take a look at how optional arguments interact with "implicit" interface implementations.
interface IFoo
{
void M(int x = 123);
}
class Foo : IFoo
{
public void M(int x){}
}
Here the method M is implicitly implemented by Foo's method M. What happens here?
Foo foo = new Foo();
foo.M();
Read more: Fabulous Adventures In Coding
SilverWii - That is connecting a WiiMote to a Silverlight OOB app via NESL (Native Extensions For Microsoft Silverlight)
Posted by
jasper22
at
10:32
|
Yeah, I know... Silverlight, Silverlight, Silverlight... I know I've been doing a good bit of Silverlight recently, and will back off it soon, but I saw this and well I just couldn't pass this up (I tried, but every time I re-org'ed my list of possible posts this one just kept bubbling all by itself to the top... lol).
Hooking up a Wiimote to a Silverlight app? Come on, you know that's officially "fun"...
NESL: Native Extension for Silverlight or No (Except Seven) Limits for Silverlight? Experimenting the Sensor API with a Wiimote
The first time I heard about the Native Extension for Silverlight, I wondered: well, in what context can I use this? In what kind of scenario? And overall, is it something really usable? In this article I will try to test one of the features included in NESL, i.e. the capability of interacting with motion sensors. At the end I used the popular wiimote controller since it has a built-in accelerometer. Read the rest of the story to find out the test result.
Read more: Channel9
Writing inline MSBuild tasks in C# for one-liners
Posted by
jasper22
at
10:30
|
Every now and then, when trying to do something in MSBuild, I hit a roadblock (or maybe just some unintuitive “feature”) and I’m left thinking “gosh, now I have to create a custom MSBuild task for this one-liner that is SO trivial in plain C#!”.
Well, no more with MSBuild 4.0. You can now write inline tasks in plain C# which can of course have input and output parameters.
For example, I needed to checkout a file from TFS before some task run and tried to write it. Checking out from TFS can easily be done with an Exec task:
<Exec Command=""$(VS100COMNTOOLS)..\IDE\tf.exe" checkout $(ExtenderNamesTargetFile)"
WorkingDirectory="$(MSBuildProjectDirectory)" />
(Note how I’m NOT using $(DevEnvDir))
That’s simple enough, but it has a problem: performance. The tf.exe command connects to the TFS server every time for the checkout, and this can take some time. Not something you want to do if the file is already checked out! So I needed a simple condition: just checkout if the file is readonly.
Of course there’s no built-in way in MSBuild to check if a file is readonly. Inline MSBuild task to the rescue!
<UsingTask TaskName="IsFileReadOnly" TaskFactory="CodeTaskFactory" AssemblyFile="$(MSBuildToolsPath)\Microsoft.Build.Tasks.v4.0.dll">
<ParameterGroup>
<FileName ParameterType="System.String" Required="true" />
<IsReadOnly ParameterType="System.Boolean" Output="true" />
</ParameterGroup>
<Task>
<Using Namespace="System.IO"/>
<Code Type="Fragment" Language="cs">
<![CDATA[
this.IsReadOnly = new FileInfo(this.FileName).IsReadOnly;
]]>
</Code>
Read more: Daniel Cazzulino's Blog
Custom Cursors in Silverlight
Posted by
jasper22
at
10:28
|
There has been quite a few blog posts on how to create custom cursors in Silverlight, but I felt some of them were very limited or not very re-useable, or required you to put the cursor in the layout and put restrictions on your layout (like for instance requiring you to use a Canvas as parent for your cursor). So I ended up writing my own class for it that doesn’t have any of these restrictions.
The basic idea is the same as other approaches out there: You “disable” the cursor on your element by setting myElement.Cursor = Cursors.None. Next you move a custom UIElement around on top of your layout which represents your custom cursor. My approach uses a Popup, and therefore never requires you to modify your layout, and you can easily change the cursor on the fly. The cursor look is defined using a DataTemplate and can contain animations and so on.
All you need to do is define a DataTemplate and use the Attached Property to define the template. Below is an example of this working on a border element:
<UserControl x:Class="CustomCursor.MainPage"
xmlns:local="clr-namespace:SharpGIS.CustomCursor">
<UserControl.Resources>
<DataTemplate x:Key="CircleCursor">
<Ellipse Fill="Red" Width="20" Height="20" />
</DataTemplate>
</UserControl.Resources>
<Grid>
<Border Background="Blue"
local:CustomCursor.CursorTemplate="{StaticResource CircleCursor}" />
</Grid>
</UserControl>
Read more: SharpGIS
Android Usb Port Forwarding
Posted by
jasper22
at
10:28
|
Introduction
The Android architecture does not allow to start communication from the Android to the host through the USB cable.The opposite is possible, using the Google "Android Debug Bridge" (ADB in short).
This tool will act as a tunnel between the Android client application and the host server. It is a software implementation of a router doing IP port forwarding.
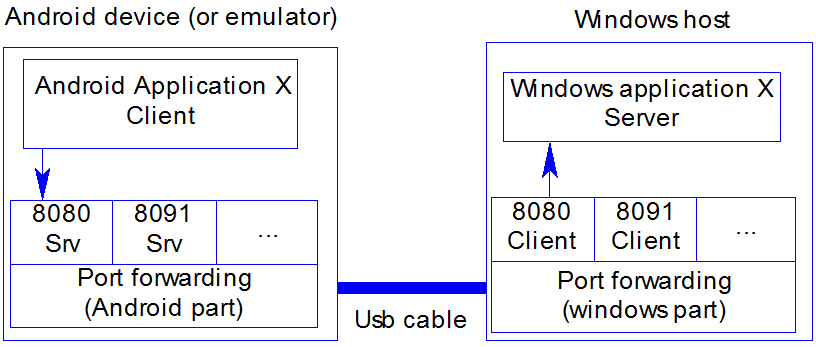
On the Host part, you have to declare the ports you want to be opened on the Android and the tunnel will transfer communications between the android and the host. The host can be any server on the network or your local host.
Prerequisits
Enable "Usb debuging" on your Android
Install "Usb Tunnel" on your Android (see donwnload link on top of the article) or from the market :
Read more: Codeproject
Using WinDbg to inspect native dump files
Posted by
jasper22
at
10:26
|
Just a very short instruction on how to inspect native dump files with WinDbg:
- Get and install and then start WinDbg
- File - Open Crash Dump
~*kb
- Lists all the threads and their call stacks.
- !locks
Will show you the critical sections. LockCount - RecursionCount - 1 = the amount of times the lock has been acquired.
- So if you have several locks that are taken you can check the call stacks and see where you have the deadlock.
- !runaway
Will show you time spent in each thread.
I find it useful to get a text dump of all the call stacks which I can search in my text editor, rather than clicking around using Visual studio when searching for a deadlock.
Read more: Jayway Team Blog
Getting Fiddler and the .NET Framework to Play (better) Together
Posted by
jasper22
at
10:25
|
I was working on a sample over this weekend and kept running into a wall related to Fiddler that was driving me crazy. Fortunately, I was able to find a solution thanks to Eric Lawrence and so I want to share that with you.
Here’s the scenario. I have a console application client which is using the HttpClient object model (from the Rest Starter Kit Preview 2) to call a WCF 4.0 service hosted on my local IIS Express at http://localhost:17697). As you can see, there’s nothing super fancy about how I’m calling my service:
static void AddNewProduct(ProductDto product) {
Console.WriteLine("Adding new product");
using(var client = new HttpClient("http://localhost:17697")) {
var content = HttpContentExtensions.CreateXmlSerializable(
product, Encoding.UTF8, "application/xml");
var response = client.Post("products", content);
Console.WriteLine("Product {0} now exists at location {1}",
product.id, response.Headers.Location);
}
}
The code works just fine by itself – the problems start when I want to insert Fiddler in the middle to monitor the HTTP traffic. Typically, this is done by either adding a “.” after localhost or using the special hostname “ipv4.fiddler” (in fact, in IE9, you no longer need the “.” anymore – Fiddler will pick up localhost just fine). However, when I try both of these (plus one more – using “127.0.0.1.”), every request yields the following (though on a positive note, it does show up in fiddler)
HTTP/1.1 400 Bad Request
Content-Type: text/html; charset=us-ascii
Server: Microsoft-HTTPAPI/2.0
Date: Sat, 07 May 2011 22:13:40 GMT
Connection: close
Content-Length: 334
Bad Request - Invalid Hostname
It turns out that the solution is to add a rule in Fiddler to allow me to use a custom hostname on my client (one that Fiddler can pick up) and then have that Fiddler rule rename the host so that the request can be handled appropriately by my server and service.
Read more: CodeBetter
Exposing WCF REST Service over HTTPS
Posted by
jasper22
at
10:20
|
In this post, I will explain; how could we expose a WCF REST Service over secure HTPP (HTTPS)? Essentially there are three steps involved
1. Create a certificate or use a third party provided certificate
2. Configure HTTPS in IIS
3. Configure webHttpBinding to use transport level security
Create Certificate
To expose a service over HTTPS, we need to have a certificate in store. Either you can create your own X.509 certificate or use certificate provided by 3rd parties.
I am going to create my own certificate. To create X.509 certificate Open Inetmgr

In center you will get an option of Server Certificates. Double click on that.

Read more: DEBUG MODE……
Remote Debugging in Visual Studio: Squashing Bugs in their Native Environment
Posted by
jasper22
at
10:18
|
your machine the way you like it, you’ve installed all manner of add-ins into Visual Studio, you’ve added a number of seemingly random assemblies to your global assembly cache. Then, you’re totally disappointed when you copy the executable to another machine and it crashes.
Of course, it’s not really quite like that. Build systems try to ensure that the hidden dependencies are brought to the surface, and large collections of untainted virtual machines allow you to quickly test an application in a clean room environment. Still, it’s impossible to test all possible configurations, and so all too often bugs turn up. In those scenarios, it would be nice get access to the customer’s machine. Using some of the modern support technologies such as NTRsupport, this is fairly easy to do, and by using WinDbg and the SOS Debugging Extension it is possible to install very little on the target machine while still being able to get enough data to diagnose the problem.
Recently, someone pointed out to me that Visual Studio has an alternative for debugging remote applications, which ought to make it a lot easier to debug problems in .NET applications. It would be nice if all that was necessary was to select Debug, Attach to Process in Visual Studio on the host machine, and then enter the name of the target machine.
Of course, it isn’t really quite that easy…
Making the target machine available for remote debugging
Before you can get started, the target machine has to make itself available for remote debugging.
The host machine talks to the target machine via DCOM in order to get the data it needs for debugging the application. The way to set this up is to run the appropriate version of msvsmon.exe, which you can find in the architecture-specific subdirectory:
\program files\microsoft Visual Studio 10.0\Common7\IDE\Remote Debugger
This executable is a DCOM server that handles the requests from remote clients, attaching to the relevant processes and getting data from them.
When the Debugging Monitor is running, it displays a view of the connections as they come in, as shown below. This is useful for debugging connection failures.
Read more: Simple-talk
AES Encryption Sample in C# (CSharp)
Posted by
jasper22
at
10:18
|
Recently I have written a post on Encryption and compression in Java. Now i had similar requirement to do in CSharp( I work on Distrubuted systems which are in Different Technology Stack). Here is the sample which does AES Encryption of files/text etc using a key
using System;
using System.IO;
using System.Security.Cryptography;
//
// Sample encrypt/decrypt functions
// Parameter checks and error handling
// are ommited for better readability
// @author:Ashwin Kumar
// Date : 12/3/2008
public class AESEncryptionUtility
{
static int Main(string[] args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: AESEncryptionUtility infile outFile");
return 1;
}
string infile = args[0];
string outfile = args[1];
//string keyfile = args[2];
//var key = File.ReadAllBytes(keyfile);
Encrypt(infile,outfile,"test");
Decrypt(outfile,"_decrypted"+infile,"test");
return 0;
}
// Encrypt a byte array into a byte array using a key and an IV
public static byte[] Encrypt(byte[] clearData, byte[] Key, byte[] IV)
{
// Create a MemoryStream to accept the encrypted bytes
MemoryStream ms = new MemoryStream();
// Create a symmetric algorithm.
// We are going to use Rijndael because it is strong and
// available on all platforms.
// You can use other algorithms, to do so substitute the
// next line with something like
// TripleDES alg = TripleDES.Create();
Rijndael alg = Rijndael.Create();
Read more: felicitas and beatitudo
Memory Forging Attempt by a Rootkit
Posted by
jasper22
at
10:13
|
Some time ago a new rootkit appeared that at first glance seemed more similar to initial variants of TDL3 than to the updated TDL4 variants we have seen this year. Like TDL3, it also parasitically infected a driver by inserting code in the resource directory of the PE file. In this case the name of the file it infected was hard-coded to volsnap.sys. Also similar to the early variants of TDL3, this rootkit also hooked some pointers in the dispatch table (IRP hook) of the driver below disk on the device stack of the hard disk.
But it was very interesting to see some of the anti-rootkit tools not showing the dispatch table hooks that are usually pretty straightforward to identify. Also this malware would not allow an external debugger (WinDbg) to break, which was annoying.
The reason for hooks not being reported was that the memory being read by the tools was not the actual memory! The dispatch table as “seen” by the tools appeared not to be hooked–whereas in reality it was hooked. The part that made it interesting was that the memory was being read at the correct address with a mov instruction and not using some system API that could be hooked. We know of some proof-of-concept ways to achieve this, but I had not seen this behavior before from a threat in the wild.
Obviously the motivation for malware authors to use such techniques is to prevent tools from showing their hooks so that administrators are not alarmed of suspicious activity.
So how does it fake memory on read access? This rootkit is using hardware breakpoints (DRX register setting) to monitor access to memory areas of the kernel that it patches. In addition to modifying the DR0 register, it hooks the KiDebugRoutine pointer so that it gets notification when the hardware breakpoint is encountered due to memory access. This rootkit installs IRP hooks and sets the DR0 register to the memory address where the IRP hook is installed. So when the memory of the dispatch table is read, a “fake” image with no hook in it is presented by the malware’s KiDebugRoutine hook. Here is a brief overview of the KiDebugRoutine hook code.
This is the beginning of the malware’s KiDebugRoutine handler code. If it encounters a breakpoint, then the malware simply increments the instruction pointer for the thread where the exception occurred and returns it as handled. Otherwise we take the jump.

Read more: McAfee
Smart Logic for Conditional WHERE Clauses
Posted by
jasper22
at
10:10
|
One of the key features of SQL databases is their support for ad-hoc queries—that is, dynamic SQL. That's possible because the query optimizer (query planner) works at runtime; each SQL statement is analyzed when received to generate a reasonable execution plan. The overhead introduced by runtime optimization can be minimized with bind parameters—Section 3, “Bind Parameter” covers that in detail.
The gist of that recap is that databases are optimized for dynamic SQL—so, use it.
There is, however, one widely used practice to avoid dynamic SQL if favour of static SQL—often because of the "Dynamic SQL is slow" myth. Unfortunately that practice is doing more harm than good.
So, let's imagine an application that queries the employees table (see Appendix C, Example Schema). It allows searching for subsidiary id, employee id and last name (case-insensitive) in any combination. The following statement support all these variants:
SELECT first_name, last_name, subsidiary_id, employee_id
FROM employees
WHERE ( subsidiary_id = :sub_id OR :sub_id IS NULL )
AND ( employee_id = :emp_id OR :emp_id IS NULL )
AND ( UPPER(last_name) = :name OR :name IS NULL )
The statement uses Oracle style named placeholder for better readability. All possible filter expressions are statically coded in the statement. Whenever a filter isn't needed, the respective search term is NULL so that the particular filter becomes ineffective.
It's a perfectly reasonable SQL statement. The use of NULL is even in line with its definition according to the three valued logic of SQL. Regardless of that, it is still amongst the worst things for performance.
Read more: Use the index, Luke
WCF Extensibility – Operation Selectors
Posted by
jasper22
at
10:05
|
We’re now entering the realm of the less used extensibility points for the WCF runtime. Operation selectors could well be left as an internal implementation detail for WCF, but for some reason the designers decided to make them public (I think the guideline back then was if anyone can come up with a scenario where a user would use it, then WCF would expose a hook for that). The server selector (IDispatchOperationSelector) is actually interesting to have as a public hook, as the whole UriTemplate model for REST services was built on top of it – basically, the selector knows about the HTTP method and URI templates for each service operation, and based on those properties of incoming messages it can call the appropriate operation. For “normal” (i.e., SOAP) endpoints, the operation selector implementation (which happens to be internal) is based on the addressing of the binding – in most of the cases it simply matches the Action header of the message with a map of action to the operation names.
The client operation selector (IClientOperationSelector) is another story. The client selector receives the method invoked by the client (the System.Reflection.MethodBase object) and it must return an operation name which will be used by the WCF runtime to retrieve the actual client operation from the client runtime. I’ve tried for a while to think of any scenario where one would actually want to call one method in the proxy and have another operation be executed (besides some practical joke scenario where one would swap Add for Subtract in a calculator), but I just can’t think of any. Even searching on Bing or Google doesn’t show any real scenarios where a client selector is used. But well, WCF is extensible, so let’s let people choose their client operations as well .
Public implementations in WCF
WebHttpDispatchOperationSelector (server): The dispatch implementation where the UriTemplate magic happens for WCF REST endpoints.
There are no public implementations for IClientOperationSelector, only an internal one which simply returns the operation name of the method being called.
Interface declaration
public interface IDispatchOperationSelector
{
string SelectOperation(ref Message message);
}
public interface IClientOperationSelector
{
bool AreParametersRequiredForSelection { get; }
string SelectOperation(MethodBase method, object[] parameters);
}
The dispatch operation selector is quite simple – on SelectOperation you get the message, you return the operation name. In most cases the operation can be selected based on information on the message header (e.g., the Action header for SOAP endpoints) or the message properties (e.g., the HTTP request property attached to the message). There are scenarios, however, where the routing to the operation needs to be done based on the message contents, so the message is passed by reference – if it needs to be consumed by the operation selector, it can recreated it to hand it back to the WCF pipeline.
On the client side the AreParametersRequiredForSelection is used to determine whether the response to SelectOperation can be cached or not – if the parameters are not required, the WCF runtime will only call SelectOperation once per method in the proxy, otherwise every time a proxy call is made the selector will be invoked again.
Read more: Carlos' blog
Microsoft to Pay $8.5 Billion Cash for Skype
Posted by
jasper22
at
10:04
|
Obviously born to pay retail, Microsoft is gonna ante up $8.5 billion cash money to scoff up Skype and turn it into its new Skype Division with CEO, Cisco veteran Tony Bates, as president. Bates will report to Microsoft CEO Steve Ballmer.
The price, more than Microsoft has ever paid for anything although it once offered $48 billion for Yahoo before it thought better of it, includes the assumption of Skype's roughly $700 million debt.
Although Skype's a freeware money loser - off $7 million on revenues of $859.8 million last year - Microsoft, which has had little luck with the Internet the past 15 years, said it expects the acquisition to generate significant new business and revenue opportunities.
Read more: .NET Journal
“MyEclipse G” Set to Revolutionize Cloud Deployments by Easing Business Users into Google Development
Posted by
jasper22
at
10:03
|
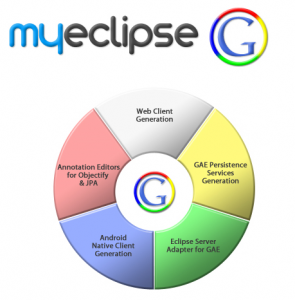
Live from Google I/O, we’re pleased to announce the newest IDE on the market; “MyEclipse G.” The “G” showcases our laser-focus on Google development technologies for the cloud. As with all our product lines, MyEclipse G is a robust solution designed for an easy user experience while creating multiple frameworks for Google App Engine. The simple-to-use cloud studio-type functionality automates project architecture and implementation, all with very little time investment from you, the developer or business user.
Read more: Genuitec blog
Read more: MyEclipse G website
IDisposable, and resource leaks: It could happen
Posted by
jasper22
at
10:02
|
I received a question about Item 15 in the second edition of Effective C#. I thought the question and answer would be of general interest, so I’m addressing it here. The question:
You stated in your book (Kindle page number 1359) that if you stack the using statements of IDisposable objects that it could have a potential resource leak if an exception is thrown. I however, have been unable to see this happen, I even wrote my own example to see if this is the case. Please can you elaborate on this particular scenario?
Here’s the sample he sent along:
class Program
{
static void Main(string[] args)
{
try
{
TestObject outer = new TestObject("outer");
TestObject inner = new TestObject("inner");
using (outer as IDisposable)
using (inner as IDisposable)
{
throw new NotImplementedException();
}
}
finally
{
Console.ReadLine();
}
}
class TestObject : IDisposable
{
public TestObject(string name)
{
Name = name;
}
public string Name { get; set; }
authors note: The rest of this blog post reads much better if you imagine it read by Henry Fonda as Juror #8 in 12 Angry Men.
The code, as written, does call Dispose() on both TestObjects created. If that’s what happened, multiple using statements like this work just fine.
But, suppose that’s not what happened. Suppose, just suppose, the constructor for TestObject throws an exception when creating the second TestObject.
In that case, the outer TestObject has been created, but neither using statement is reached. Dispose will not be called on the outer TestObject. Resources would leak. Maybe that’s what could happen. Just maybe. We should think about this more.
Maybe the constructor doesn’t throw an exception. Most developers do try to write constructors that minimize the chance of a constructor exiting by throwing an exception. Maybe that won’t happen. Maybe it’s safe as is.
Read more: Bill Wagner
Debugging Windows Service Startup with Service Isolation
Posted by
jasper22
at
09:59
|
A year and a half ago I touched on the subject of debugging process startup, such as the startup of Windows Services, using the GFlags utility (the ImageFileExecutionOptions registry key).
The general idea is to rely on the Windows loader to launch a debugger instead of the debugged process, and trace your way through the process startup code. Unfortunately, this relies on the debugged process to run in the same session as you—otherwise, you won’t be able to actually see the debugger.
Starting from Windows Vista, Windows services are isolated into a separate session to which you do not have access when you are logged onto the system. The debugger is launched within this session as well, which produces the undesired result of having the service stuck waiting for the debugger, and the debugger stuck waiting for your input which you cannot provide. (To learn more about Session 0 Isolation, check out the trusty Windows 7 Training Kit which covers several application compatibility topics with detailed code examples.)
What can you do to debug service startup on Windows Vista or newer OS versions? All you need is to fire a remote debugging server that debugs the service, and connect to its debugging session from a debugging client. Assuming that your Debugging Tools for Windows installation resides in C:\Debuggers, you can configure the following as the Debugger string in GFlags:
C:\Debuggers\ntsd.exe -server tcp:port=10000 -noio
Read more: All Your Base Are Belong To Us
May 2011 Security Release ISO Image
Posted by
jasper22
at
09:58
|
This DVD5 ISO image file contains the security updates for Windows released on Windows Update on May 10th, 2011.
Read more: MS Download
WCF Sessions - Brief Introduction
Posted by
jasper22
at
09:54
|
Introduction
Session is well understood term for all of us and as per our common understanding it is (well, less or more) some duration in which entities recognize each other. Some of us might have played with it in ASP.NET as well. Concept is almost similar in WCF though technique and usage are a bit different.
In WCF there is always a service class instance that handles incoming service requests. These instances may already be there (at server when request arrives) or may be created as needed. In WCF the concept of session is mainly to manage these service instances so that server can be utilized in optimized way. At server there is one special class named InstanceContext that creates/loads service class instance and dispatches requests to it. The correlation can be perceived as –
You can see here how stuff is engaged. When some request arrives it is routed to service instance via instance context. Suppose there is a hit of thousand requests then service will have to create thousand instance contexts (which in turn will create thousand service instances) to handle these requests. If requests are served in this way then service is called PERCALL service as each request is served by a new instance context and service instance object (call them as service objects onwards). Consider there is a client who made 100 requests. If service identifies this client and always serves it by a dedicated service object then this type of service will be known as PERSESSION service as it recognizes the client and serves it by a single instance of service object. On the other hand if all the requests irrespective of client are served by a single instance of service objects then the service will be known as SINGLETON service. Following pictures summarize the concept–
Read more: Codeproject
LateBindingApi.Office - The easiest way to use Office in .NET
Project Description
.NET Wrapper Assemblies für den Zugriff auf Microsoft Office, Excel, Word, Outlook, PowerPoint, Access
Features
Office Integration ohne Versionsbeschränkung
Alle Objekte, Methoden, Properties und Events der Office Versionen 2000, 2002, 2003, 2007, 2010 enthalten
Attribute Konzept und XML Source Doku zur Information welche Office Version(en) die jeweilige Methode oder Property anbietet(en)
Syntaktisch und semantisch identisch zu den Primary Interop Assemblies
Keine Einarbeitung wenn Sie das Office Objekt Modell bereits kennen, verwenden Sie Ihren bestehenden PIA Code
Reduzierter und besser lesbarer Code durch automatische Verwaltung von COM Proxies
Keine Deployment Hürden, keine problematische Registrierung, keine Abhängigkeiten, keine Interop Assemblies, kein VSTO notwendig
Mit jeder .NET Version ab 2.0 verwendbar
Problemlose Addin Entwicklung
Read more: Codeplex
על lsass.exe, Isass.exe, ומה שביניהם
Posted by
jasper22
at
09:04
|
שלום לכולם,
כאן דן ויזנפלד מצוות התמיכה של Microsoft.
אחד מלקוחותינו פנה אלינו עם שאלה מעניינת:
"מהו תהליך lsass.exe? במנהל המשימות?"
את התשובה לשאלה זו אחלק ל-2 חלקים.
תהליך lsass.exe הינו תהליך בסיסי וחיוני ביותר של מערכות ההפעלה מבית Microsoft. שם התהליך הינו בעצם ראשי תיבות למונח: “Local Security Authority Subsystem Service”.

התהליך lsass.exe ממוקם בתיקייה: %SystemRoot% \System32, והוא אחראי על מספר תחומי אחריות במערך האבטחה של המחשב:
1. אכיפת הגדרות מדיניות האבטחה שבמחשב (או השרת בארגונים גדולים), הידועות בכינוי Local Security Policies. הגדרות אלו מתכללות את הגדרות אבטחה במחשב שלכם, כפי שהוגדרו כברירת מחדל או על ידי מנהל המחשב.
2. אימות נתוני המשתמשים (Users) במחשב/ברשת – התהליך אחראי על בדיקת נתוני הזהות של המשתמשים המבקשים לבצע שימוש במחשב/להתחבר לשרת. רק לאחר ביצוע פעולה זו, מאפשרת מערכת ההפעלה גישה לקבצים ולתיקיות אליהן הוגדרו למשתמשים הרשאות גישה.
3. יצירת Access Tokens – יצירת פריטי מידע, מעין תעודות זהות, המצורפות לכל פעולה או תכנית, שהפעלתה דורשת הרשאות מנהל. כך יכולה מערכת ההפעלה לאכוף את הרשאות הנגישות לאותן תכניות.
4. אחריות על הפעלה ותקינות של שירותי אבטחה שונים ופרוטוקולים – כגון SSL ו-Kerberos.
את עבודת התהליך, ותיעוד הפעולות שהוא מבצע, תוכלו למצוא במציג האירועים (Event Viewer).

Read more: MS Support blog
Conference
Posted by
jasper22
at
09:02
|
Project Description
This is the web project based on Silverlight and WCF which provides the possibility of establishing sound- and video-connection between users directly on the web-page (in the Silverlight container). It's developed in C#.
Read more: Codeplex
New line in html tooltip
Posted by
jasper22
at
09:01
|
קורה לכם שאתם רוצים להציג tooltip פשוט של html ולהציג מידע בכמה שורות כשאתם מחליטים היכן לבור את השורה, תוכלו לכתוב קוד כזה:
הסימון ;13&# נותן ירידת שורה, וכעת כשעומדים על הלינק זה יראה כך:
Read more: שלמה גולדברג (הרב דוטנט)
ההבדל בין Unique Index ו-Unique Constraint
Posted by
jasper22
at
08:58
|
בפורום בסיסי נתונים בתפוז נשאלה שאלה כיצד להגדיר עמודה שאינה מפתח כ-Unique (כלומר- ללא ערכים כפולים).
עניתי- "בעזרת "Unique Index. זמן קצר לאחר מכן עדי הוסיף שאפשר להשתמש גם ב-Unique Constraint, ויש לי הרגשה שהוא ציין זאת לא בתור "גם לי יש מה לומר" אלא כי יש בהצעתו יתרון מסויים.
כדי לעמוד על ההבדלים בין שתי ההצעות (לא הרבה..), נתחיל מהדומה, וניצור לשם כך טבלה עם שתי עמודות זהות שלאחת נגדיר אינדקס ולשניה אילוץ:
If Object_Id('Try001','U') Is Not Null Drop Table Try001;
Go
Create Table Try001(ID Int Identity,
I1 Int Null,
I2 Int Null);
Go
Alter Table Try001 Add Constraint Try001_I1_Constraint Unique Nonclustered(I1)
Create Unique Index Try001_I2_Index ON Try001(I2);
Go
With T As
(Select 1 N
Union All
Select N+1 N
From T
Where N<100)
Insert
Into Try001
Select N,
N
From T;
Go
מתברר שהמערכת יצרה לאילוץ אינדקס מתאים, זהה לאינדקס שנוצר באופן ישיר (ובנוסף מציגה את האינדקס שנוצר ולא את האילוץ) וזה די הגיוני: לא סביר לצפות שבכל פעם שמכניסים או משנים ערך בעמודה - המערכת תבצע Scan על כל הטבלה כדי לבדוק שהחדש אינו קיים, ואינדקס הוא כלי עזר מתבקש במקרה זה.
ננסה להכניס ערכי Null לעמודות:
Insert
Into Try001
Values(Null,0);
Go
Insert
Into Try001
Values(0,Null);
Go
ה-Nulls נכנסו ללא בעיות שכן שתי העמודות הוגדרו כ-Nullable.
מה יקרה אם ננסה לבצע שליפות מפולטרות או ממויינות של העמודות?
Select I1 From Try001 Where I1=1;
Select I2 From Try001 Where I2=1;
Read more: גרי רשף
Peer-To-Peer Chat - Advanced!
Posted by
jasper22
at
08:56
|
Today we are going to work on a peer-to-peer, P2P, chat system. The approach that I'm taking requires that you have two computers on a local area network, or you could have one computer and use virtual machines. Basically, you can only run one peer on a single computer.
I've seen many P2P chat systems around the web that use a client/server approach using TCP (Transmission Control Protocol). I'm going to take a pure peer-to-peer approach using UDP (User Datagram Protocol). In this system there is no server, each of the peers is equal to any other peer. Just a quick background on TCP and UDP. TCP and UDP are part of the TCP/IP protocol suite for networking. TCP is a connection based protocol where you need a connection between hosts. UDP is a connectionless protocol where a sender just sends out data and doesn't care if it is received or not.
To get started create a new Windows Forms application called PeerToPeerChat. Right click the Form1.cs that was generated and select Rename. Change the name of this form to ChatForm.cs. When it asks if you want to change the instances of the form in code say yes. I also added in a very simple form to log in a user. Right click the PeerToPeerChat project in the Solution Explorer and select Add|Windows Form. Name this new form LoginForm. Onto the form I dragged a Label, Text Box, and Button. My finished form in the designer looked like this.
Read more: </dream-in-code>
AutoCompleteBox in Silverlight 4.0 with DataSource from SQL Server
Posted by
jasper22
at
08:56
|
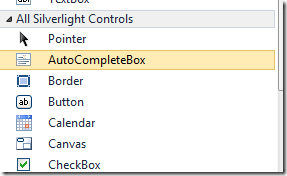
In my last article AutoCompleteBox in Silverlight 4.0 , Data Source for Autocomplete Box was an IEnumerable list. In this post we will work with a column value of table from SQL Server as Data Source for Autocomplete Box.
Our approach would be
1. Create WCF Service
2. Exposed data
3. Consume in Silverlight
4. Put data exposed by service as data source of Autocomplete Box.
I have a table called Course in School database. I am going to bind value of the Title column of Course table as source for AutoCompletebox.
Create WCF Service
Right click on web project hosting Silverlight application and add a new item by selecting WCF Service application from Web tab.
1. Very first let us create a Service Contract returning titles as list of string.
Read more: DEBUG MODE……
SlingUriffic
Posted by
jasper22
at
08:54
|
Project Description
When installed as the default browser on a Windows machine, SlingUriffic will redirect incoming URLs to the browser of choice based on Regex pattern matches. If you use different browsers to handle different sites, then this is the utility for you.
Read more: Codeplex
An Overview of Objective-C - Part 1
Posted by
jasper22
at
08:53
|
I’ve been blogging about our educational iPhone/iPod Touch RPN calculator application called CrunchTime, and so far have given an overview of the platform, talked about the vision of the project, discussed the deployment process of an iPhone application, introduced the Xcode IDE, and outlined the functional specifications of our project.
As I stated before, the main programming language for development on Apple platforms is Objective-C and in a few blog posts I’m going to give a quick overview of the main features of Objective-C that was the main thing I needed to get started with this language for our project. I’m going to use a simplified version of the code that I wrote for the calculation engine of CrunchTime to showcase the features in my examples.
Objective-C is an Object-Oriented programming language that adds a Smalltalk style of messaging to the C programming language. The syntax is a combination of original C syntax combined with Smalltalk message style even though in the newer versions some of the common syntax styles are included to make it easier for C developers to learn, use, and adapt the language.
Like C, you have multiple files for the implementation of a class in Objective-C: a header file that includes the declaration and abstractions of the class with .h extension, and an implementation file with definitions and .m extension. In this part I focus on header files.
The following code is the header file for the CalculationEngine class in CrunchTime named CalculationEngine.h.
#import <Foundation/Foundation.h>
#import "StackManager.h"
@interface ComputationEngine : NSObject
{
StackManager *mgr;
}
// Initialize the stack
-(id) init;
// Calculate the result of an operation by getting the operands from the stack
-(double) calculate: (NSString*) operator;
// Push an operand to the stack
-(void) pushOperand: (double) v;
// Pop an operand from the stack
-(double) popOperand;
// Get a pointer for the stack
-(NSArray*) getStack;
// Get the current size of the stack
-(int) getSize;
// Check to see if stack is empty
-(BOOL) isEmpty;
// Check to see if stack is full
-(BOOL) isFull;
// Clear the stack
-(void) clear;
Read more: .NET Zone
Merula shell
Posted by
jasper22
at
08:52
|
Project Description
Merula shell is a Windows shell replacement. Use the library of Merula Shell to create your own windows shell in WPF. Currently there is a DEMO avaible to show you wat Merula shell can do.
Read more: Codeplex
Inside Facebook
Posted by
jasper22
at
08:51
|
Tracking Facebook and the Facebook Platform for Developers and Marketers
Read more: Inside Facebook
Grapevine OS
Posted by
jasper22
at
08:51
|
You must read the documentation before editing the source code
Important things we need for first OS publish
Knowledge of how to get mouse coordinates in the x,y format not in VB in Cosmos (C#)
Public mouseposition as System.Drawing.Point
Contributor with knowledge of Cosmos (C#)
Project Description
Grapevine OS is a simple Cosmos experimental OS that has a GUI, it is written in VB.net however it is converted into C# for compilation. People with experience in C# and Cosmos are wanted, C# for error checking and Cosmos for the complicated stuff.
GUI Information
The GUI works by re printing the desktop every time the mouse is moved; in later versions this is hoped to be improved as more contributors join with more experience.
Read more: Codeplex
Android for .NET Developers - Building the User Interface
Posted by
jasper22
at
08:48
|
The Android for .NET Developers Series
Part 1 Starting with this article, I'll discuss what you need to know to approach Android programming without any aid from your .NET expertise.
Part 2 In this article, we'll go through an Android application that accepts input from the user and handles user's clicking.
Part 3 In this article, you will learn how to build the user interface.
Introduction
The user interface of native mobile applications often relies on a new family of widgets that do not exist - at least not in the same form - in Web and desktop user interfaces. Rounded panels, data tables, pick lists are fairly common, but I'd also call your attention on the use of bitmaps, icons and gradients that are probably more common than in other types of application.
Any Android project contains a folder of resources and resources are catalogued in subfolders by category - menu, layout, values, drawable. (See Figure 1.) You populate these folders with a bunch of files representing various visuals. In this article, I'll go through a bunch of topics that revolve around the use of resources and illustrate how to accomplish some common tasks such as displaying an image, a rounded panel, localized strings, menus, and modal dialogs. I'll start with the first type of resource you deal with even when your goal is simply writing a hello-world application - the layout resource.
Figure 1: Resources in an Android project.

Read more: dotNetSlackers
Problems with WCF Scaling
Every once in a while, people ask me about problems they have when trying to use a single WCF service with multiple concurrent calls.
The first think I tell them is to check their WCF throttling settings. The throttling behavior in WCF controls how many instances and session WCF can create and manage at once.
These settings also depend on the binding you use. For example if you have a single proxy on the client side that sends many async calls at once, and you use basicHttpBinding, WCF will by default create many instances on the service side, this is because basicHttpBinding does not support sessions. If you change the binding to wsHttpBinding which supports sessions, you will end up using a single instance (this of course depends on the instance context mode of your service).
In WCF 3.5 the throttling settings were quite low, but in .NET 4 they were increased and now they depend on the number of cores your machine has.
Of course this is the obvious reason, but after solving this issue, some people find another problem with concurrent calls, usually when making a lot of concurrent calls that span many service instances. The problem with creating a lot of service instances at once, even after increasing the throttling values, relies in the fact the WCF uses the ThreadPool to create to queue the work, and the ThreadPool controls how many threads are available for work and how to create new threads (this is the MinIOThreads setting). The ThreadPool has a minimum of threads which is set according to the machine’s CPU, and can be changed if needed (note the bug that exists in the CLR in .NET 3.5).
Read more: Ido Flatow
Your program loads libraries by their short name and you don't even realize it
Posted by
jasper22
at
10:40
|
In the discussion of the problems that occur if you load the same DLL by both its short and long names, Xepol asserted that any program which loads a DLL by its short name "would have ONLY itself to blame for making stupid, unpredictable, asinine assumptions" and that Windows should "change the loader to NOT load any dll with a short name where the short name and long name do not match."
The problem with this rule, well, okay, one problem is that you're changing the rules after the game has started. Programs have been allowed to load DLLs by their short name in the past; making it no longer possible creates an application compatibility hurdle.
Second, it may not be possible to obtain the long name for a DLL. If the user has access to the DLL but not to one of the directories in the path to the DLL, then the attempt to get its long name will fail. The "directory you can access hidden inside a directory you cannot access" directory structure is commonly used as a less expensive alternative to Access Based Enumeration. Maybe your answer to this is to say that this is not allowed; people who set up their systems this way deserve to lose.
Third, the application often does not control the path being passed to LoadLibrary, so it doesn't even know that it's making a stupid, unpredictable, asinine assumption. For example, the program may call GetModuleFileName to obtain the directory the application was installed to, then attempt to load satellite DLLs from that same directory. If the current directory was set by passing a short name to SetCurrentDirectory (try it: cd C:\Progra~1) then the program will unwittingly be making a stupid, unpredictable, asinine decision. (But since the decision is being made consistently, there was no problem up until now.)
When you call CoCreateInstance, there is nearly always a LoadLibrary happening behind the scenes, because the object you are trying to create is coming from a DLL that somebody else registered. If they registered it with a short name, then any application that wanted to use that object runs afoul of the new rule, even though the application did nothing wrong.
Now, you might argue that this just means that the component provider is the one who made a stupid, unpredicable, asinine assumption. But that may not have been a stupid assumption at the time: 16-bit applications see only short names, so it might have been that that's the only thing they can do. Or the component may have made a conscious decision to register under short names; this was common in the Windows 95 era because file names often passed through components that didn't understand long file names. (Examples: 16-bit applications, backup applications.)
Read more: The old new thing
Subscribe to:
Posts (Atom)