DotNetZip Library
DotNetZip - Zip and Unzip in C#, VB, any .NET language
DotNetZip is an easy-to-use, FAST, FREE class library and toolset for manipulating zip files or folders. Zip and Unzip is easy: with DotNetZip, .NET applications written in VB, C# - any .NET language - can easily create, read, extract, or update zip files. For Mono or MS .NET.
DotNetZip is the best ZIP library for .NET. Just look at the reviews!
DotNetZip works on PCs with the full .NET Framework, and also runs on mobile devices that use the .NET Compact Framework. Create and read zip files in VB, C#, or any .NET language, or any scripting environment. DotNetZip supports these scenarios:
- a Silverlight app that dynamically creates zip files.
- an ASP.NET app that dynamically creates ZIP files and allows a browser to download them
- a Windows Service that periodically zips up a directory for backup and archival purposes
- a WPF program that modifies existing archives - renaming entries, removing entries from an archive, or adding new entries to an archive
- a Windows Forms app that creates AES-encrypted zip archives for privacy of archived content.
- a SSIS script that unzips or zips
- An administrative script in PowerShell or VBScript that performs backup and archival.
- a WCF service that receives a zip file as an attachment, and dynamically unpacks the zip to a stream for analysis
- an old-school ASP (VBScript) application that produces a ZIP file via the COM interface for DotNetZIp
- a Windows Forms app that reads or updates ODS files
- creating zip files from stream content, saving to a stream, extracting to a stream, reading from a stream
- creation of self-extracting archives.
Read more: Codeplex
QR: 
DockPanel Suite
Posted by
jasper22
at
21:21
|
Description
The docking library for .Net Windows Forms development which mimics Visual Studio .Net.
Read more: SourceForge
QR: 
The HIGH cost of ConcurrentBag in .NET 4.0
Posted by
jasper22
at
16:54
|
I got some strange results when using concurrent collections, so I decided to try to track it down, and wrote the following code:
var count = ?;
var list = new List<object>(count);
var sp = Stopwatch.StartNew();
for (int i = 0; i < count; i++)
{
list.Add(new ConcurrentBag<int>());
}
sp.Stop();
Console.WriteLine("{0} {2} items in {1:#,#;;0}ms = {3:#,#;;0}ms per item",
sp.Elapsed, sp.ElapsedMilliseconds, count, sp.ElapsedMilliseconds / count);
And then I started to play with the numbers, and it is not good.
10 items in 2ms = 0ms per item
This is incredibly high number, you have to understand. Just to compare, List<int> takes 8 ms to create 100,000 items.
Read more: Ayende @ Rahien
QR: 
#575 – PropertyMetadata vs. FrameworkPropertyMetadata
Posted by
jasper22
at
16:50
|
When you implement a custom dependency property and you register the property by calling DependencyProperty.Register, you specify some metadata for the property by passing it an instance of PropertyMetadata. This can be an instance of the PropertyMetadata class or an instance of one of its subclasses. The differences are shown below.
PropertyMetadata – Basic metadata relating to dependency properties
CoerceValueCallback – coerce the value when being setDefaultValue – a default value for the propertyPropertyChangedCallback – respond to new effective value for the property
UIPropertyMetadata – derives from PropertyMetadata and adds:
IsAnimationProhibited – disable animations for this property?
FrameworkPropertyMetadata – derives from UIPropertyMetadata and adds:
AffectsArrange, AffectsMeasure, AffectsParentArrange, AffectsParentMeasure, AffectsRender – Should layout calculations be re-run after property value changes?
Read more: 2,000 Things You Should Know About WPF
QR: 
[Windows 8] How to create an awaitable component ?
Posted by
jasper22
at
16:48
|
C# 5, with it’s async/await pattern, is extremelly useful for asynchronous development. In Windows 8, the good point is that all the APIs which take more than 50ms must be run asynchronously !
But how do you create your own WinRT component ? This might be simple but there are some tricky points that I wanted to highlight in this blog post.
We know that each WinRT components need to be a sealed class so let’s take a look at the following code:
public sealed class Reader
{
public async static Task<IEnumerable<string>> GetItemsAsync()
{
var feedsTitle = new List<string>();
var client = new SyndicationClient();
var feeds = await client.RetrieveFeedAsync(new Uri(“http://channel9.msdn.com/coding4fun/articles/RSS”));
foreach (var item in feeds.Items)
{
feedsTitle.Add(item.Title.Text);
}
return feedsTitle.AsEnumerable();
}
}
Even if this code seems correct, it’ll fail to compile due to the following error (click on image to enlarge):

The problem here is that each type exposed via your component need to be projected using WinRT. And the compiler is not able to project the Task type.
So we need to create a wrapper that will return (and take in parameters) only types that can be projected. And, for asynchronous operations, the dedicated type is IAsyncOperation<T>, which can be obtained, from a Task object, using the AsAsyncOperation extension’s method:
public sealed class Reader
{
public static IAsyncOperation<IEnumerable<string>> GetItemsAsync()
{
return InternalGetItemsAsync().AsAsyncOperation();
}
internal async static Task<IEnumerable<string>> InternalGetItemsAsync()
{
var feedsTitle = new List<string>();
var client = new SyndicationClient();
var feeds = await client.RetrieveFeedAsync(new Uri(“http://channel9.msdn.com/coding4fun/articles/RSS”));
foreach (var item in feeds.Items)
{
feedsTitle.Add(item.Title.Text);
}
return feedsTitle.AsEnumerable();
}
}
Read more: blog.thomaslebrun.net
QR: 
Visual Studio 2012: Improving User Stories and Requirements with PowerPoint Storyboarding
Posted by
jasper22
at
12:07
|
For Visual Studio Premium and Ultimate users there is a great new add-in for PowerPoint that can really help you called the PowerPoint Storyboarding add-in. Using this feature you can quickly draft an interface design and get stakeholder feedback. Plus, since it’s PowerPoint, even stakeholders can change elements easily to show you what they really want. Let me show you how this works.
Getting Started
To get started just go to the Microsoft Visual Studio 2012 program folder and select PowerPoint Storyboarding:

This will open up PowerPoint, give you a blank slide, and take you to the Storyboarding tab:

It will also show the storyboard shapes to the right which are searchable via the search box (CTRL + G):
QR: 
HOW TO: Use SQLite in C# Metro style app
Posted by
jasper22
at
11:19
|
I got a few questions and comments about how to actually include SQLite in a C# Metro style app. Since perhaps it wasn’t clear in describing in my post, I thought a quick video might help demonstrate the steps to build and use SQLite in a C# Metro style app.
The video walks through actually building SQLite from the source (Visual Studio 2012 required…express is fine) and adding it to a C# Metro style app, create a database, populate with some data based on a class and databind the query to a ListView. The video references my OneNote notebook on the tools you need to download and build the SQLite source. It also demonstrates using the sqlite-net library from NuGet on interacting with SQLite in a C# application.
Read more: Tim Heuer
QR: 
Enabling Coded UI Test playback logs in Visual Studio 2012 Release Candidate
Posted by
jasper22
at
11:16
|
In Jason Zander’s blog post: Announcing the Release Candidate (RC) of Visual Studio 2012 and .NET Framework 4.5 he showed a new Coded UI feature -capturing playback logs.
Unfortunately CodedUI doesn’t do this by default and finding the logs isn’t always obvious.
To enable CodedUI Test Playback logs you need to set some configuration settings in the file QTAgent32.exe.config to make this work. This file can be found at:
C:\Program Files (x86)\Microsoft Visual Studio 11.0\Common7\IDE
The settings are:
<system.diagnostics>
<switches>
<!-- You must use integral values for "value".
Use 0 for off, 1 for error, 2 for warn, 3 for info, and 4 for verbose. -->
<add name="EqtTraceLevel" value="4" />
</switches>
</system.diagnostics>
and:
<appSettings>
<appSettings>
<add key="EnableHtmlLogger" value="true"/>
<add key="EnableSnapshotInfo" value="true"/>
QR: 
Facebook Open Sources Library of its Components
Posted by
jasper22
at
11:02
|
Even as Facebook continues making headlines in the wake of its IPO and a precipitous drop in its share price, the company has made a big contribution to open source. Not everyone realizes that Facebook is built on open source technology, and it has released many components to the open source community before. Now, as Jordan DeLong explains in an extensive post, the company has released a reusable C++ library of components called Folly.
Read more: Ostatic
QR: 
Loading half a billion rows into MySQL
Posted by
jasper22
at
10:19
|
Background
We have a legacy system in our production environment that keeps track of when a user takes an action on Causes.com (joins a Cause, recruits a friend, etc). I say legacy, but I really mean a prematurely-optimized system that I’d like to make less smart. This 500m record database is split across monthly sharded tables. Seems like a great solution to scaling (and it is) — except that we don’t need it. And based on our usage pattern (e.g. to count a user’s total number of actions, we need to do query N tables), this leads to pretty severe performance degradation issues. Even with memcache layer sitting in front of old month tables, new features keep discovering new N-query performance problems. Noticing that we have another database happily chugging along with 900 million records, I decided to migrate the existing system into a single table setup. The goals were:
reduce complexity. Querying one table is simpler than N tables.
push as much complexity as possible to the database. The wrappers around the month-sharding logic in Rails are slow and buggy.
increase performance. Also related to one table query being simpler than N.
Alternative Proposed Solutions
MySQL Partitioning: this was the most similar to our existing set up, since MySQL internally stores the data into different tables. We decided against it because it seemed likely that it wouldn’t be much faster than our current solution (although MySQL can internally do some optimizations to make sure you only look at tables that could possibly have data you want). And it’s still the same complexity we were looking to reduce (and would further be the only database set up in our system using partitioning).
Redis: Not really proposed as an alternative because the full dataset won’t fit into memory, but something we’re considering loading a subset of the data into to answer queries that we make a lot that MySQL isn’t particularly good at (e.g. ‘which of my friends have taken an action’ is quick using Redis’s built in SET UNION function). The new MySQL table might be performant enough that it doesn’t make sense to build a fast Redis version, so we’re avoiding this as possible premature optimization, especially with a technology we’re not as familiar with.
Dumping the old data
MySQL provides the `mysqldump’ utility to allow quick dumping to disk:
msyqldump -T /var/lib/mysql/database_data database_name
This will produce a TSV file for each table in the database, and this is the format that `LOAD INFILE’ will be able to quickly load later on.
Installing Percona 5.5
We’ll be building the new system with the latest-and-greatest in Percona databases on CentOS 6.2:
yum install Percona-Server-shared-compat Percona-Server-client-55 Percona-Server-server-55 -y
[ open bug with the compat package: https://bugs.launchpad.net/percona-server/+bug/908620]
Specify a directory for the InnoDB data
This isn’t exactly a performance tip, but I had to do some digging to get MySQL to store data on a different partition. The first step is to make use your my.cnf contains a
datadir = /path/to/data
directive. Make sure /path/to/data is owned by mysql:mysql (chown -R mysql.mysql /path/to/data) and run:
mysql_install_db --user=mysql --datadir=/path/to/data
Read more: derwiki
QR: 
40 великолепных QR-кодов
Posted by
jasper22
at
10:15
|


QR-коды — новый тип штрих-кодов, который содержит в себе информацию, но они выглядят смертельно скучно. Однако, благодаря талантливым дизайнерам, они могут превратиться в настоящее искусство.
Read more: Habrahabr.ru
QR: 
Microsoft To Run Linux On Azure
Posted by
jasper22
at
10:11
|
After years of battling Linux as a competitive threat, Microsoft is now offering Linux-based operating systems on its Windows Azure cloud service. The Linux services will go live on Azure at 4 a.m. EDT on Thursday. At that time, the Azure portal will offer a number of Linux distributions, including Suse Linux Enterprise Server 11 SP2, OpenSuse 12.01, CentOS 6.2 and Canonical Ubuntu 12.04. Azure users will be able to choose and deploy a Linux distribution from the Microsoft Windows Azure Image Gallery and be charged on an hourly pay-as-you-go basis.
Read more: Slashdot
QR: 
MSDN Magazine: June 2012
Posted by
jasper22
at
09:35
|
.jpg)
The June edition of MSDN Magazin was release online over the long weekend, with articles looking at the use of the Windows Azure Service Bus, .NET 4.5 changes, HTML5, Knockout.js, Windows Phone 7, and the usual collection of columns.
Read more: MSDN
QR: 
Solid-state revolution: in-depth on how SSDs really work
Posted by
jasper22
at
09:03
|
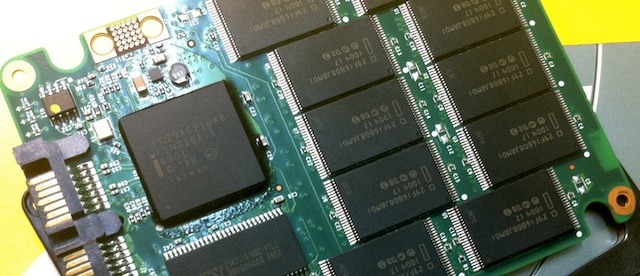
...
Solid-state drives are odd creatures. Though they sound simple in theory, they store some surprisingly complex secrets. For instance, compare an SSD to a traditional magnetic hard drive. A modern multi-terabyte spinning hard disk plays tricks with magnetism and quantum mechanics, results of decades of research and billions of dollars and multiple Nobel Prizes in physics. The drives contain complex moving parts manufactured to extremely tight tolerances, with drive heads moving around just thousandths of a millimeter above platters rotating at thousands of revolutions per minute. A modern solid-state drive performs much more quickly, but it's also a more mundane on the inside, as it's really a hard drive-shaped bundle of NAND flash memory. Simple, right?
However, the controller software powering an SSD does some remarkable things, and that little hard drive-shaped bundle of memory is more correctly viewed as a computer in its own right.
Given that SSDs transform the way computers "feel," every geek should know at least a bit about how these magical devices operate. We'll give you that level of knowledge. But because this is Ars, we're also going to go a lot deeper—10,000 words deep. Here's the only primer on SSD technology you'll ever need to read.
Varying degrees of fast
It's easy to say "SSDs make my computer fast," but understanding why they make your computer fast requires a look at the places inside a computer where data gets stored. These locations can collectively be referred to as the "memory hierarchy," and they are described in great detail in the classic Ars article "Understanding CPU Caching and Performance."
It's an axiom of the memory hierarchy that as one walks down the tiers from top to bottom, the storage in each tier becomes larger, slower, and cheaper. The primary measure of speed we're concerned with here is access latency, which is the amount of time it takes for a request to traverse the wires from the CPU to that storage tier. Latency plays a tremendous role in the effective speed of a given piece of storage, because latency is dead time; time the CPU spends waiting for a piece of data is time that the CPU isn't actively working on that piece of data.
The table below lays out the memory hierarchy:
LEVEL | ACCESS TIME | TYPICAL SIZE |
---|---|---|
Registers | "instantaneous" | under 1KB |
Level 1 Cache | 1-3 ns | 64KB per core |
Level 2 Cache | 3-10 ns | 256KB per core |
Level 3 Cache | 10-20 ns | 2-20 MB per chip |
Main Memory | 30-60 ns | 4-32 GB per system |
Hard Disk | 3,000,000-10,000,000 ns | over 1TB |
Read more: ArsTechnica
QR: 
Утекла база LinkedIn хэшей?

Вчера на форуме по подбору хэшей forum.insidepro.com пользователь под ником dwdm попросил помощи в подборе паролей к базе с SHA1 хэшами:
http://forum.insidepro.com/viewtopic.php?p=96122 (На текущий момент ссылка не работает)
Зато работает ссылка с теми самими хэшами. В базе около 6.5 миллионов SHA1 хэшей без соли.
Read more: Habrahabr.ru
QR: 
Goodbye Photoshop, Hello Cloudinary
Posted by
jasper22
at
18:01
|
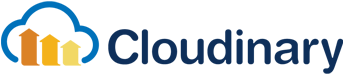
Manipulating images for your website is such a tedious chore. You need to open Photoshop, click your mouse about ten thousand times, then save the file and upload it. Then next month you redesign your site and suddenly need to re-size all your image elements again! Startup Cloudinary has a good alternative for you: use custom URLs to transform your images in the cloud! I was a bit skeptical when I first read about Cloudinary, but after five minutes of goofing around with it I’m sold.
Upload your images — either through the Cloudinary dashboard or from your own applications via their API — and then access those images using specially crafted URLs to apply a variety of transformations to your images. That’s the real magic: you don’t need to do anything other than request your image with the transformations you want. Your original photo is still available, if needed, and each new variant you request is cached and delivered through Amazon’s CDN.
You want that full-size image scaled to 100 pixels high? Here you go!
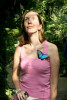
Oh, you just want the woman’s face from that photo in a nice 90×90 thumbnail? Cloudinary provides face detection, so no problem.

You need rounded corners?

Read more: Cloudinary
QR: 
Nmap 6 Released Featuring Improved Scripting, Full IPv6 Support
Posted by
jasper22
at
09:16
|
The Nmap Project is pleased to announce the immediate, free availability of the Nmap Security Scanner version 6.00 from http://nmap.org/. It is the product of almost three years of work, 3,924 code commits, and more than a dozen point releases since the big Nmap 5 release in July 2009. Nmap 6 includes a more powerful Nmap Scripting Engine, 289 new scripts, better web scanning, full IPv6 support, the Nping packet prober, faster scans, and much more!
Read more: Slashdot
Read more: NMap
QR: 
TPL Data Flow Debugger Visualizer

TPL Data Flow
I first encounter TPL data flow (part of .NET 4.5 - TPL DTF) during the build conference (I attended last year) but I must say I didn’t get it then. It sounded too much like RX which I was familiar with. Recently during one of my projects that required high performance CPU bounded – high throughput and low latency my colleague Alon the gave the idea – why not using TPL data flow and from then my world changed forever. It’s amazing to how many systems this technology can be suitable to,and for the project I was working on it was perfect. For those of you that are not yet familiar with this technology I suggest reading the article from here and see this amazing example of kinect and TPL DTF here – you’ll thank me later.
So I created some crazy TPL data flow networks on runtime (~100k different networks) and it worked getting 100% CPU most of the time, leveraging all the cores and getting really good performance – but it was really hard to debug and to understand the flow from the code. It was Alon again that suggested to write debug visualizer – so I did. This is my insights from the process.
Writing Debugger Visualizer
I don’t know how many of you had the chance to implement debugger visualizer but it is really straightforward if you need only view on a simple object just follow 5-6 steps:read here. Finally put your assembly in the visualizer folder (e.g. for VS 11 C:\Program Files (x86)\Microsoft Visual Studio 11.0\Common7\Packages\Debugger\Visualizers) and you’re done. This is very simple if your debugging target is simple and serializable. I from the other hand had few issues.
The Issues I encountered during the implementation
Debugger Visualizer Target cannot be interface
This really surprised me because I know the target I wanted was IDataFlow which is the common interface of TPL data flow which all the blocks need to implement and I discovered that it’s impossible,the target must be a type (can be common base class but not an interface). My solution to this issue was not perfect – I added multiple attributes with all the build in blocks as target. It’s not that bad because the build in blocks are a good start and as long as you start from build in block (what you normally do) then it will discover the rest of the linked block even if they are not part of the build in blocks. In addition it’s not recommended to implement IDataFlow block on your own – there is a lot to take care of: locking synchronization,buffering and more. If one of you Microsoft guys are listening I think adding support of Interface as target of debug visualizer can be very handy.
Read more: Offir Shvartz
QR: 
No more awaiting... ILSpy 2.1 now supports async/await
Posted by
jasper22
at
13:05
|
A few weeks ago, Daniel blogged about the details of how decompiling async / await is implemented. If you are only interested in the results, see the below screenshot for a proof of decompilation working (on Windows 8 RP):
Read more: Greg's Cool [Insert Clever Name] of the Day
Read more: ILSpy_Master_2.1.0.1603_RTW_Binaries.zip
QR: 
C/C++ EXEs and DLLs created by Visual Studio 2008 don't run on Windows 4.0 (ie, NT4 and Win9x)
Posted by
jasper22
at
12:16
|
Louis Solomon / SteelBytes
Written 22/Apr/08
Revised 23/Apr/08 typo's and comment about link.exe/editbin.exe, and the 'quick notes'
Added Link 25/Aug/08 My command line tool that patches compiled code ExeVersion
Added Link 27/Aug/08 other related work LegacyExtender
Here is the result of my exploring this problem ... YMMV :-)
Part 1 (NT4 and Win9x): the required OS version flags in the .exe header are set to 5.0. Can you use Link.exe's or EditBin.exe's /SUBSYSTEM switch to fix this? No, as they don't let you set a value less then 5.0 on both the OS and SubSystem fields (using older version of EditBin from some older version of VS/VC/SDK apparently works). Solution is to patch 'em back to 4.0, here is some code that will do that
HANDLE hfile = CreateFile(argv[1],GENERIC_READ|GENERIC_WRITE,0,0,OPEN_EXISTING,0,NULL);
if (hfile!=INVALID_HANDLE_VALUE)
{
HANDLE hmapping = CreateFileMapping(hfile,0,PAGE_READWRITE,0,0,0);
if (hmapping)
{
BYTE *file_base = (BYTE *)MapViewOfFileEx(hmapping,FILE_MAP_ALL_ACCESS,0,0,0,0);
if (file_base)
{
IMAGE_OPTIONAL_HEADER *ioh = (IMAGE_OPTIONAL_HEADER *)(file_base + ((IMAGE_DOS_HEADER *)file_base)->e_lfanew + 4 + sizeof(IMAGE_FILE_HEADER));
ioh->MajorOperatingSystemVersion = 4;
ioh->MinorOperatingSystemVersion = 0;
ioh->MajorSubsystemVersion = 4;
ioh->MinorSubsystemVersion = 0;
UnmapViewOfFile(file_base);
}
CloseHandle(hmapping);
}
CloseHandle(hfile);
}
Part 2 (Win9x): the CRT uses the Unicode / Widechar version of GetModuleHandle() in it's internal _crt_waiting_on_module_handle(). Here is a replacement that doesn't
extern "C" __declspec(noinline) HMODULE __cdecl _crt_waiting_on_module_handle(LPCWSTR szModuleName)
{
#define INCR_WAIT 1000
#define _MAX_WAIT_MALLOC_CRT 60000
char szModuleNameA[MAX_PATH];
WideCharToMultiByte(CP_ACP,0,szModuleName,-1,szModuleNameA,_countof(szModuleNameA),NULL,NULL);
unsigned long nWaitTime = INCR_WAIT;
HMODULE hMod = GetModuleHandleA(szModuleNameA);
Read more: louis.steelbytes.com
QR: 
How to get Visual C++ 2012 (VC 11 Beta) statically linked CRT and MFC applications to run on Windows XP
Posted by
jasper22
at
11:42
|
XPSupport wrappers version 1.09
One of the major limitations of Visual C++ 2012 is that it does not create applications that can run on Windows XP. As most of you already know, there is a connect item at Microsoft on this. See: http://connect.microsoft.com/VisualStudio/feedback/details/690617
Note that the connect item was closed as “by design” and it was stated that only Vista SP2 and above is supported. So this leaves out XP and Server 2003.
As you can see from the comments, many users simply will not adopt VC 11 because of its lack of XP targeting support. Whether the Visual Studio 11 tools run on XP is a whole other story. I don’t need the IDE to run on XP. What I need is the ability to use VC 11 (and all of its powerful new language features) AND still be able to target XP as a platform to deploy my apps to.
If you’re a long time reader of my blog, you know that I have written another article in the past on how to target Windows 2000 when using Visual Studio 2010. In that article I explained that there is a simple trick that we can use to “wrap” missing API functions. This involves making a small assembly language forwarding directive that calls our own C++ wrapper function. If we use this trick we can substitute our own versions of any API symbol that is linked into our app, without causing any multiple symbol errors in the linker. This means we do not have to use /FORCE:MULTIPLE.
To summarize, if we run dependency walker (available from www.dependencywalker.com) on a built executable that uses CRT and/or MFC 11 on Windows XP SP3, we’ll see a bunch of “red” icons beside the functions that are missing on XP (mostly in kernel32.dll). Our goal is to get rid of all of this red before it will run correctly on XP.
An aside: If it were truly a technical limitation to drop XP, then it would be impossible for us to “wrap” every instance of these functions without touching the original CRT and/or MFC static lib source code. I’m about to show you a simple, elegant way to give your VC 11 apps the gift of XP support, while allowing the exact same binary to run on higher versions as well (e.g Vista, 7, Server 2008, etc)
There is another new roadblock that Microsoft has put in our way. Previous to VC 11, we could override both the minimum operating system and subsystem version numbers using a linker command line option. However in VC 11, they only allow specification of 6.0 (Vista) as a minimum. That means there is NO way to write the necessary operating and subsystem versions to the built binary without a separate post-build tool.
Turns out that writing the operating system and subsystem version numbers can be acheived using EDITBIN.EXE (a tool that is included with VC11) This means we can use this as post-build step just after linking occurs.
All that needs to be done is to run editbin.exe on the built executable itself, e.g.
EDITBIN.EXE testapp.exe /SUBSYSTEM:WINDOWS,5.01 /OSVERSION:5.1
It still throws a warning that the subsystem supplied is invalid but writes it anyway (unlike linking which refuses to write it when it’s invalid).
Note: if you’re trying these instructions with a console application, use
EDITBIN.EXE testapp.exe /SUBSYSTEM:CONSOLE,5.01 /OSVERSION:5.1
instead.
Notice that in the title of this blog post, I say “statically linked”. There is good reason for that. Microsoft no longer provides makefiles for their DLLs (since VC2010). The last version to provide makefiles was VC2008. That is unfortunate, as I could have provided a way to make dynamically linked apps run on XP as well. But for now, we’ll have to do with a solution for statically linked apps only. I suggest you put pressure on Microsoft using the avenue of your choice to have them make available steps or makefiles that will allow us to rebuild the CRT and MFC DLLs. If they agree, then I can provide a follow-up to this blog entry.
Read more: Teds blog
QR: 
Using the DPAPI through ProtectedData Class in .Net Framework 2.0
Introduction:
The objective of this tutorial is to show how the DPAPI can be used to encrypt and decrypt data:
Encrypt some data using ProtectedData Class in System.Security.Cryptography namespace and save it to a file.
Show that the data can be decrypted using the same class but deferent method.
Login as a different user, and show that the data cannot be decrypted.
Encrypting Data:
Here we will encrypt some data, and write it to a file.
Open the attached project WriteSecretData which you will find in the WriteSecretData folder. This is a skeleton C# console application that you will use to encrypt data using the DPAPI support in .NET 2.0.
Add using directives for System.Security.Cryptography and System.IO to the other usings at the top of the file:
// Needed for encryption
using System.Security.Cryptography;
// Needed for file I/O
using System.IO;
Note you may need to add a reference to System.Security.dll, do that if needed.
Note how the code asks for some code to encrypt, and a string to use as an extra encryption key (the 'entropy').
If an entropy string isn't used, anyone logging in with the same user ID will be able to decrypt the secret data.
At the first TODO comment, insert code to turn the strings into byte arrays:
byte[] plainBytes = Encoding.Unicode.GetBytes(plainText);
byte[] entropyBytes = Encoding.Unicode.GetBytes(entropyText);
At the second TODO comment in the code, add a call to encrypt the data so that it can only be retrieved by the current user:
byte[] encryptedBytes = ProtectedData.Protect(plainBytes, entropyBytes, DataProtectionScope.CurrentUser);
Read more: C# Corner
QR: 
“Invalid License Data” after VS 11 Beta to VS 2012 RC Upgrade
Posted by
jasper22
at
10:41
|
We’ve been seeing reports of some users hitting an “Invalid License Data” error on VS startup after upgrading from VS 11 Beta to VS 2012 RC. This could be due to upgrading from a “higher” Beta SKU (e.g. Ultimate) to a “lower” RC SKU (e.g. Professional).
Read more: Aaron Marten
QR: 
Save Your Gmail Attachments to Google Drive Directly from Your Inbox [Google Chrome Extension]
Posted by
jasper22
at
10:40
|

Are you tired of having to manually download attachments from Gmail before you can add them to your Google Drive account? Then cut through all the hassle and add those attachments directly from your Inbox to your Google Drive account with the Gmail Attachments To Drive extension.
Once you have the extension installed and have refreshed your Gmail Tab (if you had it open before installation), simply click on the Save To Drive Link and within moments you will see the following message confirming the upload to your Google Drive (Docs) account.
Read more: How-to geek
QR: 
Building High Performance WPF/E Enterprise Class Application in C# 4.5
Posted by
jasper22
at
10:38
|
Preface
This article provides an in-depth reference of how to build performance-oriented applications in WPF for both Windows and the Web. This article talks about all major aspects with examples, including design pattern trade-offs, multi-threading, WCF Service calls (client server architecture or /smart Client Model), managing memory, parallelism, etc., which are a must to start building an enterprise class application from scratch.
Contents
Overview.
Introduction.
WPF/E or Silverlight
Factors need to considered for enterprise class application with WPF/Silverlight
WPF Programming Model.
Initial Architecture.
KSmart pattern for WPF.
Model Layer implementation.
Advance Memory Management.
Caching objects.
Weak References.
Garbage Collection.
Unmanaged Objects.
Asynchronous Programming.
Dispatcher.
Using the Dispatcher.
Updating the UI.
Updating the UI Asynchronously.
2. Background Worker.
Using Background Worker in WPF.
Binding.
Direction of Data Flow.
What Triggers Source Updates.
Page and Navigation.
Refreshing the current Page.
Navigation Lifetime.
Programming Guidelines for Improving Performance.
Conclusion.
Overview
This article provides in-depth reference on how to build WPF applications which is targeted for high performance and still will work similar to Silverlight or any other third party plug-ins for browser. This article also targets on the common mistake developer or architects do in choosing design patterns, implementing multi-threading or making WCF Service calls in building smart client applications. This also discuss key design issues which end in large memory consumptions, decreasing application performance, resolution issue, Managing user sessions, Parallelism etc.
This concept applies to WPF, WPF running in client (XBAP), Silverlight, all applications which sticks onto the concept of WPF.
Read more: Codeproject
QR: 
5 Cool Things You Can Do With an SSH Server
Posted by
jasper22
at
10:36
|
SSH offers more than just a secure, remote terminal environment. You can use SSH to tunnel your traffic, transfer files, mount remote file systems, and more. These tips and tricks will help you take advantage of your SSH server.
SSH doesn’t just authenticate over an encrypted connection – all your SSH traffic is encrypted. Whether you’re transferring a file, browsing the web, or running a command, your actions are private.
SSH Tunneling
SSH tunneling allows a remote SSH server to function as a proxy server. Network traffic from your local system can be sent through the secure connection to the SSH server. For example, you could direct your web browsing traffic through an SSH tunnel to encrypt it. This would prevent people on public Wi-Fi networks from seeing what you’re browsing or bypass website and content filters on a local network.
Of course, the traffic becomes unencrypted when it leaves the SSH server and accesses the Internet. To a web server you access through the tunnel, your connection will appear to be coming from the computer running your SSH server, no the local system.
On Linux, use the following command to create a SOCKS proxy at port 9999 on your local system:
ssh -D 9999 -C user@host
The tunnel will be open until your SSH connection terminates.
Open your web browser (or other application) and set the SOCKS proxy to port 9999 and localhost. Use localhost because the tunnel entrance is running on your local system.

We’ve also covered using PuTTY to set up an SSH tunnel on Windows.
SCP File Transfers
The scp, or secure copy, command allows you to transfer files between a remote system running an SSH server and your local system.
For example, to copy a local file to a remote system, use the following syntax:
scp /path/to/local/file user@host:/path/to/destination/file
Read more: How-to geek
QR: 
TCP optimization for video streaming, part 2
Posted by
jasper22
at
10:34
|
A few weeks ago, I blogged about the problems we had with TCP at this year's TG, how TCP's bursty behavior causes problems when you stream from a server that has too much bandwidth comparing to your clients, and how all of this ties into bufferbloat. I also promised a followup. I wish I could say this post would be giving you all the answers, but to be honest, it leaves most of the questions unanswered. Not to let that deter us, though :-)
To recap, recall this YouTube video which is how I wanted my TCP video streams to look:
Read more: Steinar H. Gunderson
QR: 
Перспективы OpenStack: Red Hat vs VMware
Posted by
jasper22
at
10:01
|

Одни считают облачную IaaS-платформу OpenStack самым быстро-растущим открытым проектом со времён появления ядра Linux, а другие называют её мутным технологическим «винегретом», которому суждено с грохотом провалиться. Всё зависит от того, у каких компаний вы спрашиваете и от их интересов на рынке облачного ПО.
Вот взгляд на перспективы проекта OpenStack со стороны Red Hat и VMware, а так же мнение генерального директора Virtual Instruments, Джона Томпсона, ветерана технологической отрасли c 40-летним стажем и бывшего руководителя Symantec.
Оптимистический взгляд
Брайн Стивенс (Brian Stevens), технический директор Red Hat, говорит: “только Linux развился так же стремительно, как OpenStack, и с такой же широкой поддержкой сообщества”. Всего лишь два года назад Rackspace и NASA заявили, что они собираются построить открытую облачную инфраструктуру, а OpenStack уже “привлёк заметное внимание”. “Об этой инициативе услышали по всему миру” — продолжил Стивенс.
Мнение Стивенса — не редкость. Похоже, сегодня большинство компаний находится на стороне проекта OpenStack. HP подтвердила, что будет использовать OpenStack для инфраструктуры своего публичного IaaS-облака. Сотрудники NASA проводит масштабные развёртывания OpenStack и открывают код. Десятки технологических поставщиков внедряют OpenStack, а множество предприятий интересуются тем, как создать нечто наподобие собственного Amazon Web Services.
Кстати, Red Hat — третий по величине контрибьютер OpenStack и один из платиновых спонсоров независимой организации OpenStack Foundation. Стивенс утверждает, что OpenStack может стать стандартным решением для IaaS-облаков.
Очевидно, что Стивенс поддерживает OpenStack. У Red Hat есть свои интересы на рынке облачных платформ, и этот рынок может быть хорошим источником дохода. А OpenStack, благодаря поддержке Red Hat может стать важной частью корпоративного ПО.
Read more: Habrahabr.ru
QR: 
HTG Explains: What’s the Difference Between Ubuntu & Linux Mint?
Posted by
jasper22
at
09:58
|

Ubuntu and Linux Mint are two of the most popular desktop Linux distributions at the moment. If you’re looking to take the dive into Linux – or you’ve already used Ubuntu or Mint – you wonder how they’re different.
Linux Mint and Ubuntu are closely related — Mint is based on Ubuntu. Although they were very similar at first, Ubuntu and Linux Mint have become increasingly different Linux distributions with different philosophies over time.
History
Ubuntu and other Linux distributions contain open-source software, so anyone can modify it, remix, and roll their own versions. Linux Mint’s first stable version, “Barbara,” was released in 2006. Barbara was a lightly customized Ubuntu system with a different theme and slightly different default software. Its major differentiating feature was its inclusion of proprietary software like Flash and Java, in addition to patent-encumbered codecs for playing MP3s and other types of multimedia. This software is included in Ubuntu’s repositories, but isn’t included on the Ubuntu disc. Many users liked Mint for the convenience installing the stuff by default, in contrast to Ubuntu’s more idealistic approach.
Over time, Mint differentiated itself from Ubuntu further, customizing the desktop and including a custom main menu and their own configuration tools. Mint is still based on Ubuntu – with the exception of Mint’s Debian Edition, which is based on Debian (Ubuntu itself is actually based on Debian).
With Ubuntu’s launch of the Unity desktop, Mint picked up additional steam. Instead of rolling the Unity desktop into Mint, Mint’s developers listened to their users and saw an opportunity to provide a different desktop experience from Ubuntu.
Read more: How-to geek
QR: 
How To Access The Old Control Panel From Windows 8 Start Screen [Tip]
Posted by
jasper22
at
09:32
|
One of the major changes in Windows 8 is its revamped Start Menu Screen. Instead of introducing the same old Start Menu that we’ve all grown used to, Microsoft has opted for a rather different route – creating a Metro themed Start Screen. If you’re using Windows 8 Consumer Preview, you must have noticed the absence of Control Panel shortcut in the Start Screen. Although you can easily access PC Settings console from Charms Bar, Windows 8 doesn’t offer a quick way to open the classic Control Panel, as one needs to either search it from Start Screen or open Desktop and then access it from Windows Explorer window. In what follows, we will explain how you can create a shortcut to Control Panel and pin it to Start Screen.
To begin, create a shortcut on the desktop. From Desktop right-click context menu, select New > Shortcut. A window will pop up, asking you to enter the shortcut’s target location. Simply enter the following Control Panel shortcut path.
%windir%\explorer.exe shell:::{26EE0668-A00A-44D7-9371-BEB064C98683}
Read more: Addictive tips
QR: 
Performance consideration for Async/Await and MarshalByRefObject
In the previous "What's New for Parallelism in Visual Studio 2012 RC" blog post, I mentioned briefly that for the .NET 4.5 Release Candidate, StreamReader.ReadLineAsync experienced a significant performance improvement over Beta. There's an intriguing story behind that, one I thought I'd share here.
It has to do with some interesting interactions between certain optimizations in the BCL and how the C# and Visual Basic compilers compile async methods. Before I describe the changes made, a bit of experimentation will be useful to help set the stage.
Consider the following code. The code is simply timing how long it takes to run two different async methods (which don’t actually await anything, and so will run to completion synchronously). Each method increments an integer 100 million times. The first method does so by incrementing a field 100 million times, and the second method does so by copying the field into a local, incrementing that local 100 million times, and then storing the local back to the field.
using System;
using System.Diagnostics;
using System.Threading.Tasks;
class Program
{
static void Main()
{
var sw = new Stopwatch();
var obj = new MyObj();
while (true)
{
foreach (var m in new Func<Task>[] {
new MyObj().Foo1, new MyObj().Foo2 })
{
sw.Restart();
m();
sw.Stop();
Console.WriteLine("{0}: {1}", m.Method.Name, sw.Elapsed);
}
Console.WriteLine();
}
}
}
class MyObj
{
const int ITERS = 100000000;
private int m_data;
public async Task Foo1()
{
for (int i = 0; i < ITERS; i++) m_data++;
}
Read more: Stephen Toub - MSFT
QR: 
Subscribe to:
Posts (Atom)