Covariance and Contravariance FAQ
In this post I’ll try to answer the most common questions I find on forums and in documentation feedback about C# covariance and contravariance. It’s a big topic for a single blog post, so expect to see a lot of “more information” links.
Special thanks to Eric Lippert and Chris Burrows for reviewing and providing helpful comments.
What are covariance and contravariance?
In C#, covariance and contravariance enable implicit reference conversion for array types, delegate types, and generic type arguments.Covariance preserves assignment compatibility and contravariance reverses it.
The following code demonstrates the difference between assignment compatibility, covariance, and contravariance.
// Assignment compatibility.
string str = "test";
// An object of a more derived type is assigned to an object of a less derived type.
object obj = str;
// Covariance.
IEnumerable<string> strings = new List<string>();
// An object that is instantiated with a more derived type argument
// is assigned to an object instantiated with a less derived type argument.
// Assignment compatibility is preserved.
IEnumerable<object> objects = strings;
// Contravariance.
// Assume that I have this method:
// static void SetObject(object o) { }
Action<object> actObject = SetObject;
// An object that is instantiated with a less derived type argument
// is assigned to an object instantiated with a more derived type argument.
// Assignment compatibility is reversed.
Action<string> actString = actObject;
In C#, variance is supported in the following scenarios:
Covariance in arrays (since C# 1.0)
Covariance and contravariance in delegates, also known as “method group variance” (since C# 2.0)
Variance for generic type parameters in interfaces and delegates (since C# 4.0)
What is array covariance?
Arrays are covariant since C# 1.0. You can always do the following:
object[] obj = new String[10];
In the above code, I assigned an array of strings to an array of objects. So I used a more derived type than that originally specified, which is covariance.
Covariance in arrays is considered “not safe,” because you can also do this:
obj[0] = 5;
This code compiles, but it throws an exception at run time because obj is in fact an array of strings and cannot contain integers.
What is delegate, or method group, variance?
This feature was added in C# 2.0. When you instantiate a delegate, you can assign it a method that has a more derived return type than that specified in the delegate (covariance). You can also assign a method that has parameter types less derived than those in the delegate (contravariance).
Here’s a quick code example illustrating the feature and some of its limitations.
static object GetObject() { return null; }
static void SetObject(object obj) { }
static string GetString() { return ""; }
static void SetString(string str) { }
static void Main()
{
// Covariance. A delegate specifies a return type as object,
// but I can assign a method that returns a string.
Func<object> del = GetString;
// Contravariance. A delegate specifies a parameter type as string,
// but I can assign a method that takes an object.
Action<string> del2 = SetObject;
// But implicit conversion between generic delegates is not supported until C# 4.0.
Func<string> del3 = GetString;
Func<object> del4 = del3; // Compiler error here until C# 4.0.
}
By the way, this feature works for all delegates, both generic and non-generic, not just for Func and Action delegates.
For more information and examples, see Covariance and Contravariance in Delegates on MSDN and Eric Lippert’s post Covariance and Contravariance in C#, Part Three: Method Group Conversion Variance.
What is variance for generic type parameters?
This is a new feature in C# 4.0. Now, when creating a generic interface, you can specify whether there is an implicit conversion between interface instances that have different type arguments. For example, you can use an interface instance that has methods with more derived return types than originally specified (covariance) or that has methods with less derived parameter types (contravariance). The same rules are applied to generic delegates.
While you can create variant interfaces and delegates yourself, this is not the main purpose for this feature. What is more important is that a set of interfaces and delegates in .NET Framework 4 have been updated to become variant.
Here’s the list of updated interfaces:
IEnumerable<T> (T is covariant)
IEnumerator<T> (T is covariant)
IQueryable<T> (T is covariant)
IGrouping<TKey, TElement> (TKey and TElement are covariant)
IComparer<T> (T is contravariant)
IEqualityComparer<T> (T is contravariant)
IComparable<T> (T is contravariant)
Read more: C# Frequently Asked Questions
2011 Japanese Earthquake and Tsunami
Posted by
jasper22
at
10:23
|

A massive 8.9 magnitude earthquake hit the Pacific Ocean nearby Northeastern Japan at around 2:46pm on March 11 (JST) causing damage with blackouts, fire and tsunami. On this page we are providing the information regarding the disaster and damage with realtime updates.
The large earthquake triggered a tsunami warning for countries all around the Pacific ocean.
Local Japan Emergency dials:
171 + 1 + line phone number to leave a message
171 + 2 + line phone number to listen to the message
Read more: Google Crisis response
Help The Victims of the Japanese Earthquake
Posted by
jasper22
at
10:22
|
As you have probably heard, around 2:46PM in Japan on March 11th an 8.9 Magnitude earthquake struck near the coast of Honshu, Japan, which triggered a widespread Tsunami warning all along the Pacific Ocean Coastline, displacing and killing many people, and flooding the land in Japan with Tsunamis. We at XDA don’t normally get involved in such situations but we feel the need to support the victims of this tragedy in any way possible. Here are few resources you can utilize to learn more:
- Google’s Person Finder Online App
- Pacific Tsunami Warning Center
- Google Crisis Response
- News Results for Japan Earthquake
- USGS Earthquake Results
- Twitter Search for Japan Earthquake
- Japan News App made by XDA Member dy57
- BBC Live Feed Updater
Although we are not affiliated with any charities or relief organizations, we stress the importance of supporting the victims in Japan and helping in any way possible. If you feel inclined to donate to a relief organization in order to help this effort, you can use one of the few below that we have listed or any one of your choice.
Read more: XDA developers and me
Monodrid Preview 14 Available
Posted by
jasper22
at
10:18
|
Good news everyone!
We are happy to release another preview of MonoDroid (r9779)!
Release notes are available here:
Enjoy!
Jonathan
Read more: Monodroid list
Security Technical Implementation Guides (STIGS) and Supporting Documents
The STIGs and the NSA Guides are the configuration standards for DOD IA and IA-enabled devices/systems.
A Security Checklist (sometimes referred to as a lockdown guide, hardening guide, or benchmark configuration) is essentially a document that contains instructions or procedures to verify compliance to a baseline level of security.
Security Readiness Review Scripts (SRRs) test products for STIG compliance. SRR Scripts are available for all operating systems and databases that have STIGs, and web servers using IIS. The SRR scripts are unlicensed tools developed by the Field Security Office (FSO) and the use of these tools on products is completely at the user's own risk.
Read more: STIGS
WPF PasswordBox Control
The password box control is a special type of TextBox designed to enter passwords. The typed in characters are replaced by asterisks. Since the password box contains a sensible password it does not allow cut, copy, undo and redo commands. 

<StackPanel>
<Label Content="Password:" />
<PasswordBox x:Name="passwordBox" Width="130" />
</StackPanel>
Databind the Password Property of a WPF PasswordBox
When you try to databind the password property of a PasswordBox you will recognize that you cannot do data binding on it. The reason for this is, that the password property is not backed by a DependencyProperty.
The reason is databinding passwords is not a good design for security reasons and should be avoided. But sometimes this security is not necessary, then it's only cumbersome that you cannot bind to the password property. In this special cases you can take advantage of the following PasswortBoxHelper.
<StackPanel>
<PasswordBox w:PasswordHelper.Attach="True"
w:PasswordHelper.Password="{Binding Text, ElementName=plain, Mode=TwoWay}"
Width="130"/>
<TextBlock Padding="10,0" x:Name="plain" />
</StackPanel>
The PasswordHelper is attached to the password box by calling the PasswordHelper.Attach property. The attached property PasswordHelper.Password provides a bindable copy of the original password property of the PasswordBox control.
public static class PasswordHelper
{
public static readonly DependencyProperty PasswordProperty =
DependencyProperty.RegisterAttached("Password",
typeof(string), typeof(PasswordHelper),
new FrameworkPropertyMetadata(string.Empty, OnPasswordPropertyChanged));
public static readonly DependencyProperty AttachProperty =
DependencyProperty.RegisterAttached("Attach",
typeof(bool), typeof(PasswordHelper), new PropertyMetadata(false, Attach));
private static readonly DependencyProperty IsUpdatingProperty =
DependencyProperty.RegisterAttached("IsUpdating", typeof(bool),
typeof(PasswordHelper));
public static void SetAttach(DependencyObject dp, bool value)
{
dp.SetValue(AttachProperty, value);
}
public static bool GetAttach(DependencyObject dp)
{
return (bool)dp.GetValue(AttachProperty);
}
public static string GetPassword(DependencyObject dp)
{
return (string)dp.GetValue(PasswordProperty);
}
Read more: WPF Tutorial
Sending MMS with Android
Posted by
jasper22
at
13:42
|
Following on from my last post about saving a view as an image, today I want to demonstrate how to send this image via MMS. We used this technique in our application, PinPoint to send out maps via MMS.
I have previously written about how to send SMSs with Android intents and while sending MMS via intents goes to the same application, the intent that needs to be constructed is quite different. The intent requires the following items to be set:
- the action set to be ACTION_SEND
- the mime type set to image/*
- a Uri extra inserted with the key Intent.EXTRA_STREAM
- optionally some text body with the key sms_body
Intent sendIntent = new Intent(Intent.ACTION_SEND);
sendIntent.putExtra("sms_body", "some text");
sendIntent.putExtra(Intent.EXTRA_STREAM, Uri.parse(url));
sendIntent.setType("image/png");
The url being passed to the Uri.parse method should be of the form used to access the media store such as content://media/external/images/media/23. The previous article of views and images returns a url String that can directly be used by this MMS intent.
Read more: jTribe
30 Creative Resume (CV) Designs for Inspiration
Posted by
jasper22
at
13:17
|
You cannot use your CV just like a tool to store information and showcase it to your future employers from time to time. It’s time to think out of the box and get creative, do something that will make people appreciate your creativity and get inspired to create something as well. I have recently discovered some amazing samples of creatively designed resumes, and I was truly amazed. I have decided to go creative as well, after seeing these and hope you will too!


A highlighting AutoCompleteBox in Silverlight
Posted by
jasper22
at
09:57
|
Some days ago someone in the Silverlight forums asked about how an auto-complete box that highlights the matched parts of potential hits could be made. Something like:

There is no built-in feature for this in the AutoCompleteBox and I didn't have a simple answer to that question. However I found it an interesting topic and a useful feature, so I decided to put some research in it as soon as I have some time. As it happens, I just had that time, and the result is this blog post :-). As always you can download the full source code at the end of the post.
Preliminary consideration
When I thought about the problem and the fact that you can put virtually everything into an auto-complete box item (it fully supports data templates for its items, see my article on the control here), I realized that this cannot be an extension of the control itself, but has to be a separate element, like a custom text block that can be used within the item template of an auto-complete box. At first I wanted to do exactly that, create a custom text block, but that class is sealed; so I had to use a user control that wraps a text block instead. Here is what I did.
A custom text block user control
The idea was to use multiple runs in a single text block, with a font weight of bold for the highlighted parts, and normal runs for the rest. To this end, the item text potentially needs to be split into several fragments first. To accomplish this, the following pieces of information are needed:
- The text to process.
- The filter string.
- The filter mode the auto-complete box is using.
Obviously all of this has to come from the auto-complete box control, so the user control I created has dependency properties, one for each of these three pieces, that can be used for data binding in XAML to retrieve these values automatically. Like that:
<sdk:AutoCompleteBox x:Name="ContainsAutoCompleteBox"
ItemsSource="{Binding Items}"
Width="300"
FilterMode="Contains"
MinimumPrefixLength="2">
<sdk:AutoCompleteBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<local:HighlightingTextBlock Text="{Binding}"
HighlightedText="{Binding Text, ElementName=ContainsAutoCompleteBox}"
FilterMode="{Binding FilterMode, ElementName=ContainsAutoCompleteBox}" />
</StackPanel>
Read more: Mister Goodcat
XNA for Silverlight developers
This article is part 6 of the series "XNA for Silverlight developers":
- XNA for Silverlight developers: Part 0 – Why should I care?
- XNA for Silverlight developers: Part 1 – Fundamentals
- XNA for Silverlight developers: Part 2 – Text rendering
- XNA for Silverlight developers: Part 3 - Animation (transforms)
- XNA for Silverlight developers: Part 4 - Animation (frame-based)
- XNA for Silverlight developers: Part 5 - Input (touch + gestures)
- XNA for Silverlight developers: Part 6 - Input (accelerometer)
Read more: Silverlight Show
DEV-BOX: VIRTUAL MACHINES FOR DEVELOPERS
Posted by
jasper22
at
19:32
|
This post goes out to all the coders using a Windows machine for development. Might be interesting for others aswell but I can’t say much about that as I’m a Windows user myself ;)
So what is this about? Well, some time ago I ran into smaller problems when trying to move a website from my local machine (which used XAMPP) to a real server (which was running on Ubuntu or some other Linux distro) and it was hard to fix the problems because of the differences between Windows and Linux servers.
I talked about this with a friend of mine and he told me about Virtual Machines (=VM). I had never heard of them before but what I heard was intriguing. Virtual Machines allow you to run a machine inside your machine, e.g. a Linux server inside your Windows machine. So you can use this VM with Linux to test your website locally before moving it to the real server. I know that no server is like the other and you might still run into some problems due to different library versions and so on, but you gotta admit that two Linux servers have way more in common than a Linux and a Windows server ;)
So in this post I’ll tell you how to setup your own VM with all the libs you need. Even if you haven’t worked with Linux before, you should be able to follow this tutorial/example easily.
Read more: DAVID BEHLER
Iterate Over Object Properties and Property Attributes w/Reflection
Posted by
jasper22
at
19:31
|
Define custom attribute class as follows as well as placing the attribute reference on the property “FirstName”. In the following we are creating our own custom attribute by defining an attribute class which derives from Attribute which makes identifying attribute definitions in metadata easy. The AttributeUsage attribute can be used to limit which asset the attribute can be placed such as class, struct, property etc. In addition, in the example below
I have disallowed multiple similar attributes from being used on the same property designated by AllowMultiple = false
[AttributeUsage(AttributeTargets.Property, AllowMultiple = false, Inherited = false)]
public class CustomItemAttribute : Attribute {
public string FieldName { get; set; }
private bool _isMultiValue = false;
public bool IsMultiValue {
get { return _isMultiValue; }
set {
_isMultiValue = value;
}
}
public CustomItemAttribute(string fieldName) {
this.FieldName = fieldName;
}
}
public class Item{
[CustomItem("myfieldname")]
public string FirstName { get; set;}
}
Now to iterate over the properties. Notice in the class Item above how we can exclude the 'Attribute' text in the name of the attribute applied to the FirstName property. Below we can use Type.GetProperties method to get the names of the properties for a specific type. The method GetProperties returns an array of PropertyInfo objects and the property names aer available through PropertyInfo.Name. If you want to get only a subset of the properties such as public static ones you can use BindingFlags parameters (Public/NonPublic, Instance/Static). i.e. PropertyInfo[] infos = typeof(Item).GetProperties(BindingFlags.Public|BindingFlags.Static);
private void IterateOverProperties() {
CustomItemAttribute customItemAttribute;
Type type = typeof(Item);
//for each property of object of Item
foreach (PropertyInfo propInfo in type.GetProperties()) {
//for each custom attribute on the property loop
foreach CustomItemAttribute attr in propInfo.GetCustomAttributes(typeof(CustomItemAttribute), false)) {
customItemAttribute = attr as CustomItemAttribute;
Read more: david yardy pe, mcsd.net
How to test a class member that is not public using Visual Studio 2010 ?
Posted by
jasper22
at
19:30
|
There are various ways to test a Class member which is not public
- By making the private members public of a class under test
- Breaks the encapsulation principle
- API is modified just for the sake of testing
- Breaks the encapsulation principle
- API is modified just for the sake of testing
- Breaks the encapsulation principle
- API is modified just for the sake of testing
- PrivateObject to access non public Instance members of a class under test
- PrivateType to access static members of a class under test
These classes are inside Microsoft.VisualStudio.TestTools.UnitTesting namespace. I have created code snippets in Visual Studio 2010.CodeProject
Read more: Daily .NET tips
How Google Tests Software - A Brief Interlude
Posted by
jasper22
at
19:28
|
These posts have garnered a number of interesting comments. I want to address two of the negative ones in this post. Both are of the same general opinion that I am abandoning testers and that Google is not a nice place to ply this trade. I am puzzled by these comments because nothing could be further from the truth. One such negative comment I can take as a one-off but two smart people (hey they are reading this blog, right?) having this impression requires a rebuttal. Here are the comments:
"A sad day for testers around the world. Our own spokesman has turned his back on us. What happened to 'devs can't test'?" by Gengodo
"I am a test engineer and Google has been one of my dream companies. Reading your blog I feel that Testers are so unimportant at Google and can be easily laid off. It's sad." by Maggi
First of all, I don't know of any tester or developer for that matter being laid off from Google. We're hiring at a rapid pace right now. However, we do change projects a lot so perhaps you read 'taken off a project' to mean something far worse than the reality of just moving to another project. A tester here may move every couple of years or so and it is a badge of honor to get to the point where you've worked yourself out of a job by building robust test frameworks for others to contribute tests to or to pass off what you've done to a junior tester and move on to a bigger challenge. Maggi, please keep the dream alive. If Google was a hostile place for testers, I would be working somewhere else.
Second, I am going to dodge the negative undertones of the developer vs tester debate. Whether developers can test or testers can code seems downright combative. Both types of engineers share the common goal of shipping a product that will be successful. There is enough negativity in this world and testers hating developers seems so 2001.
Read more: Google test blog
How to rescue a broken stack trace: Recovering the EBP chain
Posted by
jasper22
at
19:27
|
When debugging, you may find that the stack trace falls apart:
ChildEBP RetAddr
001af118 773806a0 ntdll!KiFastSystemCallRet
001af11c 7735b18c ntdll!ZwWaitForSingleObject+0xc
001af180 7735b071 ntdll!RtlpWaitOnCriticalSection+0x154
001af1a8 2f6db1a9 ntdll!RtlEnterCriticalSection+0x152
001af1b4 2fe8d533 ABC!CCriticalSection::Lock+0x12
001af1d0 2fe8d56a ABC!CMessageList::Lock+0x24
001af234 2f6e47ac ABC!CMessageWindow::UpdateMessageList+0x231
001af274 2f6f040e ABC!CMessageWindow::UpdateContents+0x84
001af28c 2f6e4474 ABC!CMessageWindow::Refresh+0x1a8
001af360 2f6e4359 ABC!CMessageWindow::OnChar+0x4c
001af384 761a1a10 ABC!CMessageWindow::WndProc+0xb31
00000000 00000000 USER32!GetMessageW+0x6e
This can't possible be the complete stack. I mean, where's the thread procedure? That should be at the start of the stack for any thread.
What happened is that the EBP chain got broken, and the debugger can't walk the stack any further. If the code was compiled with frame pointer optimization (FPO), then the compiler will not create EBP frames, permitting it to use EBP as a general purpose register instead. This is great for optimization, but it causes trouble for the debugger when it tries to take a stack trace through code compiled with FPO for which it does not have the necessary information to decode these types of stacks.
Begin digression: Traditionally, every function began with the sequence
push ebp ;; save caller's EBP
mov ebp, esp ;; set our EBP to point to this "frame"
sub esp, n ;; reserve space for local variables
and ended with
mov esp, ebp ;; discard local variables
pop ebp ;; recover caller's EBP
ret n
This pattern is so common that the x86 has dedicated instructions for it. The ENTER n,0 instruction does the push / mov / sub, and the LEAVE instruction does the mov / pop. (In C/C++, the value after the comma is always zero.)
Read more: The old new thing
Introduction to the Nmap Security Scanner
Posted by
jasper22
at
19:27
|
A talk presented by SkullSpace member Mak Kolybabi at Winnipeg Code Camp 2011 on February 26th.
In recent years, the Nmap Security Scanner has evolved from a simple port scanner written during the Internet`s infancy into the most widely used security scanning tool in the world. We`ll look at Nmap`s past, present, and future, paying special attention to the embedded scripting language that`s been the most important factor in Nmap`s evolution, and how the community`s contributions, particularly a group of Winnipeg programmers, have shaped Nmap`s history.
Read more: Vimeo video
Silverlight Unit Test For Phone
Posted by
jasper22
at
19:26
|
There has been some confusion about how to make the Silverlight Unit Tests work on Windows Phone. The latest release of the Silverlight Unit Tests comes with the Silverlight Toolkit, and it targeted at Silverlight 4. Windows Phone is based on an enhanced version of Silverlight 3 and cannot use these DLLs.
Fortunately, Jeff Willcox has made the right DLLs available on his web site.
Silverlight 3 binaries for the Silverlight Unit Test Framework
You will need to ‘unblock’ the zip file before unpacking the files. These binaries are strong named, but are not Authenticode signed, so they are not official
To get started, follow these steps:
- Make sure Visual Studio 2010 is installed
- Download, unblock and install the Silverlight Unit Tests
- Create a new Windows Phone project
- Add references to the two DLLs
- Microsoft.Silverlight.Testing
- Microsoft.VisualStudio.QualityTools.UnitTesting.Silverlight
Be sure to put the unit tests inside the same project as the project you are testing, though it is good programming practice to isolate the tests in their own folder and namespace.
Be sure to add using statements in each file that must recognize the testing framework (certainly in mainpage.xaml.cs) for the two DLLs
using Microsoft.Phone.Controls;
using Microsoft.Silverlight.Testing;
Read more: Jesse Liberty
Chrome Stable Release
Posted by
jasper22
at
19:25
|
The Google Chrome team is excited to announce the arrival of Chrome 10.0.648.127 to the Stable Channel for Windows, Mac, Linux, and Chrome Frame. Chrome 10 contains some really great improvements including:
New version of V8 - Crankshaft - which greatly improves javascript performance
New settings pages that open in a tab, rather than a dialog box
Improved security with malware reporting and disabling outdated plugins by default
Sandboxed Adobe Flash on Windows
Password sync as part of Chrome Sync now enabled by default
GPU Accelerated Video
Background WebApps
webNavigation extension API (experimental but ready for testing)
Security fixes and rewards:
Please see the Chromium security page for more detail. Note that the referenced bugs may be kept private until a majority of our users are up to date with the fix.
As can be seen, a few lower-severity issues were rewarded on account of being particularly interesting or clever. And some rewards were issued at the $1500 and $2000 level, reflecting bug reports where the reporter also worked with Chromium developers to provide an accepted patch.
Read more: Google chrome releases
Mapping Sockets to a Process In .NET Code
Posted by
jasper22
at
19:25
|
One feature added to Fiddler a few years ago is the ability to map a given HTTP request back to the local process that initiated it. It turns out that this requires a bit of interesting code, because the .NET Framework itself doesn’t expose any built-in access to the relevant IPHelper APIs that provide this information.
I found a number of samples on the web, but for Fiddler, performance is a critical consideration because Fiddler needs to determine the originating process for every new connection. Hence, I’ve written the following code, which maximizes performance by minimizing copies between Windows and managed code.
// This sample is provided "AS IS" and confers no warranties.
// You are granted a non-exclusive, worldwide, royalty-free license to reproduce this code,
// prepare derivative works, and distribute it or any derivative works that you create.
//
// This class invokes the Windows IPHelper APIs that allow us to map sockets to processes.
// See http://www.pinvoke.net/default.aspx/iphlpapi/GetExtendedTcpTable.html as a reference
//
// We could consider a cache of recent hits to improve performance, but the performance is already pretty good, and
// creating a reasonable cache expiration policy could prove tricky. Client connection reuse already provides a significant
// optimization as it behaves in the same way as an explicit cache would.
//
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
using System.Net.NetworkInformation;
using System.Net;
using System.Diagnostics;
using System.Collections;
namespace Fiddler
{
internal class Winsock
{
#region IPHelper_PInvokes
private const int AF_INET = 2; // IPv4
private const int AF_INET6 = 23; // IPv6
private const int ERROR_INSUFFICIENT_BUFFER = 0x7a;
private const int NO_ERROR = 0x0;
// Learn about IPHelper here: http://msdn2.microsoft.com/en-us/library/aa366073.aspx and http://msdn2.microsoft.com/en-us/library/aa365928.aspx
// Note: C++'s ulong is ALWAYS 32bits, unlike C#'s ulong. See http://medo64.blogspot.com/2009/05/why-ulong-is-32-bit-even-on-64-bit.html
[DllImport("iphlpapi.dll", ExactSpelling = true, SetLastError = true)]
private static extern uint GetExtendedTcpTable(IntPtr pTcpTable, ref UInt32 dwTcpTableLength, [MarshalAs(UnmanagedType.Bool)] bool sort, UInt32 ipVersion, TcpTableType tcpTableType, UInt32 reserved);
/// <summary>
/// Enumeration of possible queries that can be issued using GetExtendedTcpTable
/// </summary>
private enum TcpTableType
{
BasicListener,
BasicConnections,
BasicAll,
OwnerPidListener,
OwnerPidConnections,
OwnerPidAll,
OwnerModuleListener,
OwnerModuleConnections,
OwnerModuleAll
}
/* This code is now obsolete as I'm now using pointer-arithmetic to directly access the table rows instead of mapping structs on top of the
* returned block of data. I'm keeping the code here for now for debugging purposes.
[StructLayout(LayoutKind.Sequential)]
private struct TcpRow
{
[MarshalAs(UnmanagedType.U4)]
internal TcpState state;
[MarshalAs(UnmanagedType.U4)]
internal UInt32 localAddr;
[MarshalAs(UnmanagedType.U4)]
internal UInt32 localPortInNetworkOrder;
[MarshalAs(UnmanagedType.U4)]
internal UInt32 remoteAddr;
[MarshalAs(UnmanagedType.U4)]
internal UInt32 remotePortInNetworkOrder;
[MarshalAs(UnmanagedType.U4)]
internal Int32 owningPid;
}
private static string TcpRowToString(TcpRow rowInput)
{
return String.Format(">{0}:{1} to {2}:{3} is {4} by 0x{5:x}",
(rowInput.localAddr & 0xFF) + "." + ((rowInput.localAddr & 0xFF00) >> 8) + "." + ((rowInput.localAddr & 0xFF0000) >> 16) + "." + ((rowInput.localAddr & 0xFF000000) >> 24),
((rowInput.localPortInNetworkOrder & 0xFF00) >> 8) + ((rowInput.localPortInNetworkOrder & 0xFF) << 8),
(rowInput.remoteAddr & 0xFF) + "." + ((rowInput.remoteAddr & 0xFF00) >> 8) + "." + ((rowInput.remoteAddr & 0xFF0000) >> 16) + "." + ((rowInput.remoteAddr & 0xFF000000) >> 24),
((rowInput.remotePortInNetworkOrder & 0xFF00) >> 8) + ((rowInput.remotePortInNetworkOrder & 0xFF) << 8),
rowInput.state,
rowInput.owningPid);
}
*/
Using MvvmLight with (XAML) Silverligtht 4 or WPF
Posted by
jasper22
at
19:23
|
Introduction
This tutorial will try and explain the advantages and the 'how to' of using MVVMLight within your XAML application. So that you can produce code that easier mantainable and more importantly testable.
Why Use The MVVM Pattern
The tight coupling of GUI to code behind has over the years meant that testing the code-behind can be some what tricky. In that how do you mimic the selected event in the GUI to call the binded event code - you can mimic teh call to your event code but how do you get the selected item from the combo (in the GUI) that doesn't exist, as you are not running the GUI but testing the methods that the GUI uses.
What was needed was a loose coupling between the GUI and the code behind, this is where Context classes come in - XAML (Silverlight or WPF) have a concept where a Silverlight page can have a (context) class associated with it for it's events and also (more importantly) have setters and getters associated with the bindings to gui controls. So that, when a property is updated by it's setter - a call to your 'NotifyPropertyChanged' method in the class 'INotifyPropertyChanged' will trigger the rebinding to the control.
Install And Integrating MVVMLight Into Your Project
You can download and follow the instructions from Gala's web site here http://www.galasoft.ch/mvvm/getstarted/
OR
Get NUGet (which is a handy plugin for Visual Studio - http://nuget.codeplex.com/releases) to convert your application into a MVVMLight application.
How to integret NUGet into VS2010-> http://nuget.codeplex.com/wikipage?title=Using%20the%20Extension%20Manager%20to%20Install%20the%20Library%20Package%20Manager%20(NuGet)
How to add MVVMLight dlls to your project.
http://nuget.codeplex.com/wikipage?title=Finding%20and%20Installing%20a%20Package%20Using%20the%20Add%20Library%20Package%20Reference%20Dialog%20Box (in this tutorial search for MVVMLight)
Use this tutorial as a guide to adding the dll's to your project
Below is how to convert you project to an MVVMLight project after installing NUGet and running the MVVMLight installer.
Read more: Codeproject
Light probes
Posted by
jasper22
at
18:44
|
Hello everyone. I wanted to show you something I’ve been working on last couple of FAFFs. The purpose of this post is to interest some of the technical types among you. If you don’t care about a technical discussion about an unfinished feature, you can just read about the basics or jump right to the video showing some WIP pretty pixels.
The Basics
The problem we are trying to solve is how to use baked lighting on dynamic objects and characters. After lightmapping a scene all static objects have nice, high quality lighting. If we don’t do anything about the dynamic objects though, they might look dull and detached from the environment with their direct light and ambient only.
One solution is to use light probes to sample the nice baked lighting at various points in the scene. We can then interpolate the nearby probes to guess what would the lighting be at the current location of our character (or other moving object) and use the result to light the character.
Let’s see how it looks like in action! (Apologies for the cheesy lighting with over the top light bounce, but it makes it easier to illustrate the effect)
Read more: Unity 3D blog
Creating a Custom Markup Extension in WPF (and soon, Silverlight)
Posted by
jasper22
at
11:51
|
WPF currently, and Silverlight in v5, enables you to create your own custom markup extensions. Markup extensions are those little strings in XAML that are typically (but not always) enclosed in {curly braces} which, as the name implies, add new functionality to XAML. The most commonly used markup extensions are the {StaticResource}, {TemplateBinding}, and {Binding} extensions. Creating your own markup extensions is a nice way to integrate your view of the world into the toolset. They're particularly popular with MVVM and similar toolkits.
This article is, in part, an excerpt from early work in Silverlight 5 in Action, the revised edition of Silverlight 4 in Action. SL5 in Action is due out by the end of 2011, and will have a MEAP (early access bits) around the time of the first public developer release of Silverlight 5.
Creating a Simple Markup Extension
There's not much ceremony to creating a markup extension. The amount of effort required is directly proportional to the complexity of what you're trying to do. For example:
public class HelloExtension : MarkupExtension
{
public HelloExtension() { }
public override object ProvideValue(
IServiceProvider serviceProvider)
{
return "Hello";
}
}
As shown in this example, to create a markup extension, you need only inherit from MarkupExtension and override the ProvideValue method. The empty constructor is necessary for use in XAML, specifically when you include additional parameterized constructors. When you use the markup extension, you must declare the namespace as explained in section 2.1.2 (of Silverlight 4/5 in Action), and use the class name in your XAML. For example, the following XAML uses the HelloExtension markup extension we just created.
<Grid xmlns:ext="clr-namespace:CustomMarkupExtensions">
<TextBlock Text="{ext:Hello}" />
</Grid>
Read more: 10Rem.NET
NEW APP: Wave Launcher
Posted by
jasper22
at
11:50
|
We’ve just been alerted to the existence of www.wavelauncher.com%2F&sref=rss" rel="nofollow">Wave Launcher, a new Home screen tool for Android 2.1 phones and higher. It adds a curved swipe bar to the bottom of your Home screen, allowing easy access to groups of apps and links.
It’s all customisable, so you can stick whatever links and apps you like in your little shortcut menu. And it looks a bit like this:

Read more: Android Flow
Kudos for the Amanuens Translation System!
Posted by
jasper22
at
11:47
|
Back in August we tried out what was then a fairly new service, Amanuens, that makes it easy to crowd source translation. We tried it with several translators, but at the time it seemed confusing and difficult to use so we stopped using it for a while. Since then Amanuens has made a lot of improvements to the usability and now we have used it successfully with a small group of translators and it seems very much easier. So if you are interested in translating mojoPortal into a new language or updating an existing translation, let us know on this forum thread and we can see about setting up an account for you to help with translation. The Amanuens service makes it easy to translate the .resx files used for all labels and buttons, and these are the most important files to translate, but there are also message templates and help files that are just plain text files. These files are not supported by Amanuens so we still work by having you send those in a .zip if you translate them. 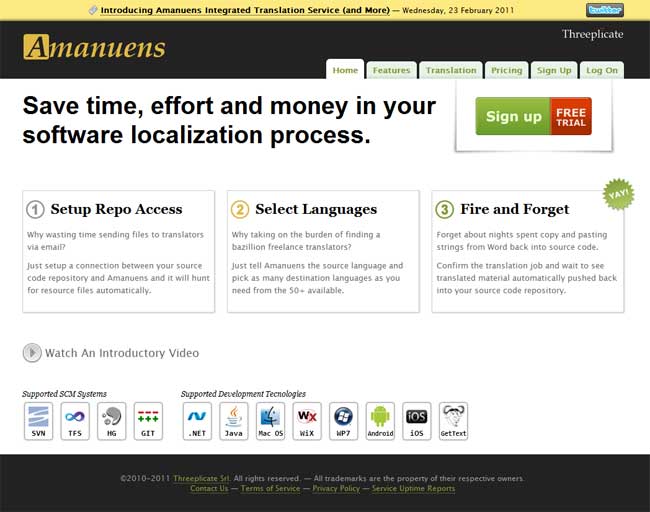
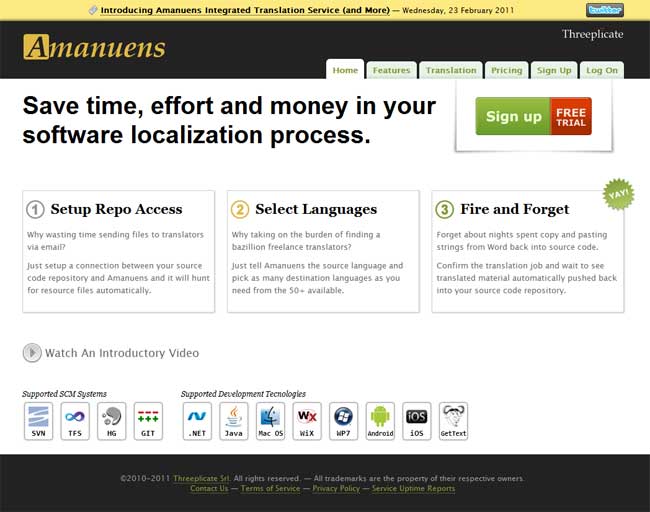
Read more: MojoPortal
SGen – Finalization And Weak References
Posted by
jasper22
at
11:46
|
Introduction
In this installment of my series on SGen, Mono’s new garbage collector, we shall be looking at how finalizers and weak references are implemented, and why you (almost certainly) should not use finalizers.
Tracking Object Lifetime
Both finalizers and weak references need to track the lifetime of certain objects in order to take an action when those objects become unreachable. To that end SGen keeps lists of finalizable objects and weak references which it checks against at the end of every collection.
If the object referred to by a weak reference has become unreachable, the weak reference is nulled.
If a finalizable object is deemed unreachable by the collector, it is put onto the finalization queue and it is marked, since it must be kept alive until the finalizer has run. Of course, all objects that it references have to be marked as well, so the main collection loop is activated again.
Generations
A nursery collection cannot collect an object in the major heap, so it is not necessary to check the status of old objects after a nursery collection. That is why SGen keeps separate finalization and weak reference lists for the nursery and major heaps.
Invoking Finalizers
SGen uses a dedicated thread for invoking finalizers. The finalization queue is processed one object at a time. As long as an object is in the finalization queue it is also considered live, i.e. the finalization queue is a GC root.
Resurrection
Resurrecting an object means making it reachable again from within its finalizer or a finalizer that can still reach the object (or via a tracking weak reference). The garbage collector does not have to treat this case specially—until the finalizer has run the object is considered live by virtue of its being in the finalization queue, and afterwards it is live because it is reachable through some other root(s).
Tracking Weak References
If an object is weakly referenced and has a finalizer, the weak reference will be nulled during the same collection as the finalizer is put in the finalization queue. That is not always desirable, especially for objects that might be resurrected.
Tracking references solve the problem by keeping the reference intact at least until the finalizer has run. Once the finalizer has finished, a tracking reference acts like a standard weak reference, i.e. it will be nulled once the object becomes unreachable, typically during the next collection (unless the object was resurrected).
Read more: Juggling, Photography, Software, and Atheism
Random tip: wtf is"7 CDCs" and debugging on a Samsung Galaxy Tab
Posted by
jasper22
at
11:44
|
ust a quick note on some issues I had debugging a MonoDroid app I've been playing with (MonoDroid is very cool, BTW)... for some reason I couldn't get a Samsumg Galaxy Tab to be recognised as a USB device when connected to my 64-bit Windows 7 PC.
Googling turned up a few different 'ideas' like Samsung Galaxy S Problems, Samsung Kies Software and PC connectivity and Connect Samsung Galaxy S with Kies on your PC - The MTP Error. None of those suggestions worked for me, despite a lot of mucking about with driver installs.
It turned out a hint in this post on USB connection trouble fixed my problem... "somehow" the device's USB port was set to 'modem mode' and I had to type *#7284# on the phone keypad to re-set it! Un-in-tuitive!
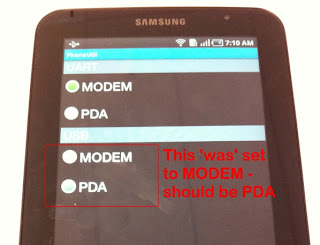
Read more: ConceptDev (Craig Dunn's blog)
gui-thread-check
Posted by
jasper22
at
11:44
|
MonoDevelop often makes use of threads to run operations on the background. Although we make sure to invoke all GUI update methods through the main GUI thread, sometimes there is a bug and an update is done in the secondary thread, which causes all sort of random locks and crashes.
To make it easier to track down those bugs, I created a simple profiler module for Mono which can detect invocations to GTK# methods from a thread other than the main GUI thread. This module is available here:
To use it, build and install the module, and then run your application with
the command:
mono --profile=gui-thread-check yourapp.exe
If the profiler is properly installed, you'll see an output like this:
*** Running with gui-thread-check ***
*** GUI THREAD INITIALIZED: 2861676352
Read more: Food for Monkeys
Linux From Scratch 6.8 is released! Step-by-step instructions on how to build your own Linux-based OS from scratch
Posted by
jasper22
at
11:43
|
Linux from scratch6.8 is released, This release includes numerous changes to LFS-6.7 and security fixes. It also includes editorial work on the explanatory material throughout the book, improving both the clarity and accuracy of the text.
About Linux From Scratch:
"Linux From Scratch (LFS) is a project that provides you with the steps necessary to build your own custom Linux system. There are a lot of reasons why somebody would want to install an LFS system. The question most people raise is "why go through all the hassle of manually installing a Linux system from scratch when you can just download an existing distribution like Debian or Redhat". That is a valid question which I hope to answer for you. The most important reason for LFS's existence is teaching people how a Linux system works internally. Building an LFS system teaches you about all that makes Linux tick, how things work together, and depend on each other. And most importantly, how to customize it to your own taste and needs." LFS
Linux from scratch is more a project than a Linux distribution in a traditional sense of the word, Linux From Scratch is a book of step-by-step instructions on how to build a minimalist Linux-based operating system from scratch - either by using an existing Linux installation or from a live CD. The book also introduces and guides the reader through additions to the system including networking, X, sound, printer and scanner support.
Read more: Unixmen
How Dynamic Language Runtime(DLR) feature in .NET Framework 4 is a boon for debugging COM objects
Posted by
jasper22
at
11:42
|
The dynamic language runtime (DLR) is a new runtime environment that adds a set of services for dynamic languages to the CLR. For more details regarding new features of .NET Framework 4 please read New C# Features in the .NET Framework 4 and What’s New in the .NET Framework 4
In this blog I’ll show how this feature can make life easy while developing/debugging COM applications and compare the same COM application running in frameworks prior to .NET Framework 4.
I’ll compare how easy it is to debug a Word Addin in .NET Framework 4 than frameworks prior to it e.g. .NET Framework 3.5.
As displayed below I’ve the screenshot from VS 2008(.NET Framework 3.5) Quickwatch window
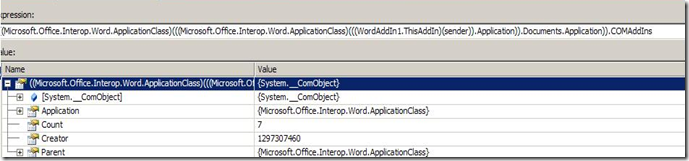
...
...
When the Watch window displays an object that implements the IDynamicMetaObjectProvider. interface, the debugger adds a special Dynamic View node to the watch display. The Dynamic View node shows members of the dynamic object but does not allow editing of the member values. For more information please read Dynamic View
Now I can view the item(ComAddin in this example) at index 1 of COMAddins object in .NET Framework 4 because of DLR as displayed below

Read more: Daily .NET Tips
References and Pointers, Part One
Posted by
jasper22
at
11:38
|
Writing code in C# is really all about the programmatic manipulation of values. A value is either of a value type, like an integer or a decimal, or it's a reference to an instance of a reference type, like a string or an exception. Values you manipulate always have a storage location that stores the value; those storage locations are called "variables". Often in a C# program you manipulate the values by describing which variable you're interested in.
In C# there are three basic operations you can do to variables:
* Read a value from a variable
* Write a value to a variable
* Make an alias to a variable
The first two are straightforward. The last one is accomplished by the "ref" and/or "out" keywords:
void M(ref int x)
{
x = 123;
}
...
int y = 456;
M(ref y);
The "ref y" means "make x an alias to the variable y". (I wish that the original designers of C# had chosen "alias" or some other word that is less confusing than "ref", since many C# programmers confuse the "ref" in "ref int x" with reference types. But we're stuck with it now.) While inside M, the variable x is just another name for the variable y; they are two names for the same storage location.
There's a fourth operation you can do to a variable in C# that is not used very often because it requires unsafe code. You can take the address of a fixed variable and put that address in a pointer.
unsafe void M(int* x)
{
*x = 123;
}
...
int y = 456;
M(&y);
The purpose of a pointer is to manipulate a variable itself as data, rather than manipulating the value of that variable as data. If x is a pointer then *x is the associated variable.
Clearly pointers are very similar to references, and in fact references are implemented behind the scenes with a special kind of pointer. However, you can do things with pointers that you cannot do with references. For example, this doesn't do anything useful:
int Difference(ref double x, ref double y)
{
return y - x;
}
...
double[] array = whatever;
difference = Difference(ref array[5], ref array[15]);
That's illegal; it just takes the difference of the two doubles and tries to convert it to an int. But with pointers you can actually figure out how far apart in memory the two variables are:
unsafe int Difference(double* x, double* y)
{
return y - x;
}
...
double[] array = whatever;
fixed(double* p1 = &array[5])
fixed(double* p2 = &array[15])
difference = Difference(p1, p2); // 10 doubles apart
Read more: Fabulous Adventures In Coding
Introducing the Google APIs Explorer
Posted by
jasper22
at
11:37
|
Google is always looking for new ways to make it easier for developers to get started with our APIs. When you come across a new Google API, you often want to try it out without investing too much time. With that in mind, we are happy to announce the Google APIs Explorer, an interactive tool that lets you easily try out Google APIs right from your browser. Today, the Explorer supports over a half dozen APIs – and we expect that number to grow rapidly over the coming weeks and months.

By selecting an API you want to explore, you can see all the available methods and parameters along with inline documentation. Just fill out the parameters for the method you want to try and click “Execute”. The Explorer composes the request, executes it, and displays the response in real time. For some APIs that access private data you will need to “Switch to Private Access” and authorize the Explorer to do so.
Read more: The official Google code blog
Windows Forms Training Videos (114)
Posted by
jasper22
at
11:36
|
How Do I: SqlAzureLOB Line of Business
How Do I: Use Dallas Data with WinForms Applications?
How Do I: Use SQL Azure with WinForms applications?
How Do I: WinForms WPF Integration
How Do I: LINQ with Entity Framework
How Do I: Entity Framework and Windows Forms Using VS 2010 beta
How Do I: Use the Windows 7 Taskbar ProgressBar
How Do I: Use JumpLists in Windows 7 Applications
How Do I: Use Overlay Icons in Win 7 app with Windows API Codepack and VS 2010 B2?
How Do I: Customize a user control with the smart tag feature
How Do I: Use the FileSystemWatcher Class
How Do I: Use the Observer Pattern
How Do I: Use The Tag Property of Controls
How Do I: Use Linq With Files
How Do I: Create a Report From a Business Object?
How Do I: Create a Report From a Database?
Using the Enterprise Library – Exploring Validation
WinForms-EnterpriseLibrary
WinForms-FormToForm Using Properties
Control When Bound Data is Updated
FormToForm Using Parameters
How Do I: Use the MSChart Control in VS 2008
How Do I: Use Visual Studio 2008 SP1′s PrintForm Control
How Do I: Use Compiler Constants
How Do I: Use Hashing to Secure Information
How Do I: Use Isolated Storage
How Do I: Use the NotifyIcon Control
How Do I: Use NullableTypes in Windows Forms
How Do I: Use Encryption in Windows Forms
How Do I: Create Bindable Objects
Singleton Design Pattern
How Do I: Use Dallas Data with WinForms Applications?
How Do I: Use SQL Azure with WinForms applications?
How Do I: WinForms WPF Integration
How Do I: LINQ with Entity Framework
How Do I: Entity Framework and Windows Forms Using VS 2010 beta
How Do I: Use the Windows 7 Taskbar ProgressBar
How Do I: Use JumpLists in Windows 7 Applications
How Do I: Use Overlay Icons in Win 7 app with Windows API Codepack and VS 2010 B2?
How Do I: Customize a user control with the smart tag feature
How Do I: Use the FileSystemWatcher Class
How Do I: Use the Observer Pattern
How Do I: Use The Tag Property of Controls
How Do I: Use Linq With Files
How Do I: Create a Report From a Business Object?
How Do I: Create a Report From a Database?
Using the Enterprise Library – Exploring Validation
WinForms-EnterpriseLibrary
WinForms-FormToForm Using Properties
Control When Bound Data is Updated
FormToForm Using Parameters
How Do I: Use the MSChart Control in VS 2008
How Do I: Use Visual Studio 2008 SP1′s PrintForm Control
How Do I: Use Compiler Constants
How Do I: Use Hashing to Secure Information
How Do I: Use Isolated Storage
How Do I: Use the NotifyIcon Control
How Do I: Use NullableTypes in Windows Forms
How Do I: Use Encryption in Windows Forms
How Do I: Create Bindable Objects
Singleton Design Pattern
(more..)
Read more: MS-Joe (Joe Stagner)
WPF – Using Data Triggers
Posted by
jasper22
at
11:33
|
Data triggers are a great feature of WPF. They allow you to change the style properties of a control depending on the data of the bound items of that control.
In this tutorial, I am going to bind a set of data to a ListBox, and use Data Triggers to let me know when something is wrong.
So first, I am going to create a Person class. This class will have three public properties: Name, Age, and IsValid. All three properties will be readonly. The IsValid property will return whether the data of the class is good or not.
public class Person
{
private string name;
public string Name
{
get { return name; }
private set { name = value; }
}
private int age;
public int Age
{
get { return age; }
private set { age = value; }
}
public bool IsValid
{
// will return false if either the name is blank
// or if the age is 0.
get { return (!string.IsNullOrEmpty(name) && age != 0); }
}
Read more: Eclipsed4utoo's Blog
Magnifying Effect in WPF
Posted by
jasper22
at
11:32
|
Intoduction
The purpose of this article is to give you an idea on how you can go about creating a magnification effect in your WPF applications. The concepts you'll pick up will hopefully be of use in a full fledged application that would require such functionality. I will also explain how you can go about using my Magnifier control, a UserControl that functions like an Image control with a magnification region.
Requirements
To run the project provided from the download link above you require either of the following,
Visual Studio 2010
Expression blend
If you have Visual Studio 2008 you can download the source files from here.
NB: If you're using the express edition of Visual Studio, ensure that you open the solution using Visual Basic Express.
Magnifier (The demo)
How it Works
Move the mouse pointer over the image. A magnification region will show up. Move your mouse to move the magnification region around the image. (If you start feeling concerned I urge you not to worry. You should see the other guy's car.)
Read more: Codeproject
Why am I receiving "Error C000009A" after installing Windows 7 Service Pack 1 (SP1)?
Posted by
jasper22
at
11:30
|
“After installing Windows 7 Service Pack 1 (SP1), you might receive the following error message on a blue screen:
"Error C000009A applying update operation {###} of {###} (\Registry...)"
To resolve this issue, restore your computer to a point in time before you installed Windows 7 SP1, uninstall any unused language packs, and then reinstall SP1. To restore your computer to a previous point in time, you'll need to use the System Recovery Options menu.”
Read more about error C000009A and how to resolve the error
Read more: I'm PC
Read more: Microsoft Windows
WCF Client Server Application with Custom Authentication, Authorization, Encryption and Compression
Posted by
jasper22
at
11:28
|
Introduction
Logical solution
WCF considerations
Implementation
Security considerations
How to use the code
Notes
References
History
Logical solution
WCF considerations
Implementation
Security considerations
How to use the code
Notes
References
History
Introduction
With the appearance of Windows Communication Foundation building Service Oriented Applications became easier than ever. And lots of articles poured in with extensions for special cases. Even so, there are still situations left untreated. Like the following I had to resolve:
- Client-server application – http protocol – NO IIS
- Authentication – user/password from a database – NO SSL/X509 certificate
- Authorization – roles from a database
- Encryption for the credentials (with option for the entire request/response)
- Compression for both the request and response.
Logical solution
For each of the above requirements/restrictions we have:
- No IIS – we need our own http server – can be easily done by WCF in a few lines of code - no need to insist on this.
- User/Password authentication – this easily done by default, but an X509 certificate is required. Therefore we need our own mechanism: we’ll add the credentials to the header of the message and encrypt them (or the entire message).
- Encryption – we’ll use an asymmetric algorithm[1] (RSA) with public/private keys. Usually it is needed that the server and the client have their own set of public/private keys to encrypt both the request and the response but we’ll use an artifice to avoid the client set of keys.
With RSA only a small amount of data can be encrypted (for 2048 bit encryption only 128 bytes of data). Therefore it is used to encrypt a random generated password that will be used by a symmetric algorithm[2] (AES) to encrypt the message. The server decrypts with its private key, the password of the client, with that password decrypts the message. And using the same password encrypts the response message.
Additional security for the credentials – even if the credentials are encrypted, a listener can get those encrypted credentials and launch a new request, therefore we’ll add an expiration date.
- Compression – we’ll use gzip compression/decompression just before sending/receiving the message and the response.
Now, let’s see how the above generic considerations look in the client-server message flow:
1. Server starts; a new RSA key is generated (or loaded from the disk).
2. Client starts; it asks the server for the public key and time; based on server time and client time it will calculate the client-server-timespan.
3. Client prepares the request message
a. The credentials are added to the message header; the Credentials token will have User/Password/Expires properties; the Expires will be calculated as client time + client-server-timespan + a few seconds;
b. The message (or only the credentials part) is encrypted; more exactly:
- a random key is generated and saved along with the message id;
- the message (or the credentials part) is encrypted with the AES algorithm using the previous generated key;
- the AES’ key is encrypted using the server’s RSA public key and added to the encrypted message
c. The message is compressed
4. Server receives the request message
a. The message is decompressed
b. The message is decrypted; more exactly:
- the client AES’ key is retrieved by decrypting using the server’s private key;
- the message is decrypted using the client key;
- the client’s key will be saved along with the message id – it will be needed to encrypt the response with the same id;
c. The credentials are extracted from the message; the Expires is compared with server’s current time and if it is bigger an AuthenticationException is thrown;
d. Authentication – the credentials are verified against a database;
e. Authorization – the roles of the authenticated user are retrieved from a database/cache.
5. Server prepares the response message
a. The message is encrypted; more exactly the message will be encrypted (AES) using the client’s key saved during decryption of the request;
b. The message is compressed
6. Client receives the response message
a. The message is decompressed.
b. The message is decrypted using the key saved during encryption (3.b.)
WCF considerations
How to extend WCF to implement the above flow:
On the client, for adding the credentials we’ll use a BehaviorExtensionElement that implements IClientMessageInspector which has a BeforeSendRequest method (see implementation here).
Read more: Codeproject
Subscribe to:
Posts (Atom)